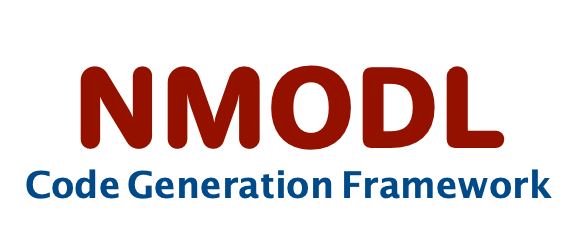 |
User Guide
|
Go to the documentation of this file.
24 #include <string_view>
44 using printer::CodePrinter;
63 const std::shared_ptr<symtab::Symbol>
symbol;
77 return var.
symbol->get_name();
239 const std::string& name,
240 const std::unordered_set<CppObjectSpecifier>& specifiers = {
369 const std::
string& verbatim);
385 const std::
string& concentration) override;
391 std::
string hoc_function_name(const std::
string& function_or_procedure_name) const;
404 std::
string py_function_name(const std::
string& function_or_procedure_name) const;
454 const std::
string& name,
455 bool use_instance) const override;
466 bool use_instance = true) const override;
477 bool use_instance = true) const;
491 std::
string get_variable_name(const std::
string& name,
bool use_instance = true) const override;
573 const std::
string& function_name = "") override;
829 void print_cvode_setup(const std::
string& setup_name, const std::
string& update_name);
void print_verbatim_cleanup(const std::vector< std::string > ¯os_defined)
Print #undefs to erase all compatibility macros.
void print_nrn_destructor() override
Print nrn_destructor function definition.
void print_global_var_external_access()
Print functions for EXTERNAL use.
Represents a C code block.
void print_nrn_cur_non_conductance_kernel() override
Print the nrn_cur kernel without NMODL conductance keyword provisions.
std::string py_function_signature(const std::string &function_or_procedure_name) const
Get the signature of the npy <func_or_proc_name> function.
Helper to represent information about index/int variables.
void print_nrn_cur() override
Print nrn_cur / current update function definition.
void print_macro_definitions()
Print all NEURON macros.
void print_function_definitions()
Print function and procedures prototype definitions.
void print_net_event_call(const ast::FunctionCall &node) override
Print call to net_event.
std::string hoc_py_wrapper_signature(const ast::Block *function_or_procedure_block, InterpreterWrapper wrapper_type)
Return the wrapper signature.
void print_nrn_constructor_declaration()
void print_neuron_includes()
Print includes from NEURON.
void print_codegen_routines_regular()
Anything not SUFFIX nothing.
void print_nrn_init(bool skip_init_check=true)
Print the nrn_init function definition.
std::string internal_method_arguments() override
Arguments for functions that are defined and used internally.
void print_nrn_alloc() override
Print nrn_alloc function definition.
std::string register_mechanism_arguments() const override
Arguments for register_mech or point_register_mech function.
std::string get_name(ast::Ast const *sym)
int position_of_int_var(const std::string &name) const override
Determine the position in the data array for a given int variable.
void visit_lon_diffuse(const ast::LonDiffuse &node) override
visit node of type ast::LonDiffuse
std::string nrn_current_arguments()
std::string table_thread_function_name() const
Name of the threaded table checking function.
bool optimize_ion_variable_copies() const override
Check if ion variable copies should be avoided.
void print_cvode_tolerances()
Print the CVODE function for setup of tolerances.
std::string hoc_function_name(const std::string &function_or_procedure_name) const
All functions and procedures need a hoc <func_or_proc_name> to be available to the HOC interpreter.
encapsulates code generation backend implementations
std::string global_variable_name(const SymbolType &symbol, bool use_instance=true) const override
Determine the variable name for a global variable given its symbol.
Represent ions used in mod file.
std::string nrn_thread_internal_arguments() override
Arguments for "_threadargs_" macro in neuron implementation.
Implement classes for representing symbol table at block and file scope.
std::string backend_name() const override
Name of the code generation backend.
ParamVector nrn_current_parameters()
void print_entrypoint_setup_code_from_prop()
Prints setup code for entrypoints NEURON.
void print_global_param_default_values()
Print global struct with default value of RANGE PARAMETERs.
ParamVector ldifusfunc1_parameters() const
Parameters for what NEURON calls ldifusfunc1_t.
void print_g_unused() const override
Set g_unused (conductance) for NRN_PRCELLSTATE feature.
void visit_watch_statement(const ast::WatchStatement &node) override
TODO: Edit for NEURON.
void print_net_send_call(const ast::FunctionCall &node) override
Print call to net_send.
void print_net_move_call(const ast::FunctionCall &node) override
Print call to net_move.
std::string thread_variable_name(const ThreadVariableInfo &var_info, bool use_instance=true) const
Determine the C++ string to print for thread variables.
void print_cvode_setup(const std::string &setup_name, const std::string &update_name)
Print the CVODE setup function setup_name that calls the CVODE update function update_name.
void print_nrn_state() override
Print nrn_state / state update function definition.
void print_initial_block(const ast::InitialBlock *node)
Print the initial block.
bool needs_v_unused() const override
void print_global_function_common_code(BlockType type, const std::string &function_name="") override
Print common code for global functions like nrn_init, nrn_cur and nrn_state.
std::string float_variable_name(const SymbolType &symbol, bool use_instance) const override
Determine the name of a float variable given its symbol.
Auto generated AST classes declaration.
void print_hoc_py_wrapper_function_definitions()
void print_cvode_definitions()
Print all callbacks for CVODE.
void print_v_unused() const override
Set v_unused (voltage) for NRN_PRCELLSTATE feature.
void print_ion_variable() override
ParamVector functor_params() override
The parameters of the Newton solver "functor".
void print_mechanism_register_regular()
Function body for anything not SUFFIX nothing.
void print_mechanism_register_nothing()
Function body for SUFFIX nothing.
Visitor for printing C++ code compatible with legacy api of CoreNEURON
Base class for all block scoped nodes.
Represents a INITIAL block in the NMODL.
void print_point_process_function_definitions()
Print POINT_PROCESS related functions Wrap external NEURON functions related to POINT_PROCESS mechani...
void print_nrn_destructor_declaration()
void print_net_init()
Print NET_RECEIVE{ INITIAL{ ...
Represent WATCH statement in NMODL.
void print_neuron_global_variable_declarations()
Print extern declarations for neuron global variables.
Represents a BREAKPOINT block in NMODL.
void print_nrn_cur_conductance_kernel(const ast::BreakpointBlock &node) override
Print the nrn_cur kernel with NMODL conductance keyword provisions.
std::string int_variable_name(const IndexVariableInfo &symbol, const std::string &name, bool use_instance) const override
Determine the name of an int variable given its symbol.
InterpreterWrapper
Enum to switch between HOC and Python wrappers for functions and procedures defined in mechanisms.
void print_global_variables_for_hoc() override
Print byte arrays that register scalar and vector variables for hoc interface.
ParamVector cvode_setup_parameters()
Get the parameters for functions that setup (initialize) CVODE.
ParamVector internalthreadargs_parameters()
The parameters for the four macros _internalthreadargs*_.
std::string get_pointer_name(const std::string &name) const
Determine the C++ string to replace pointer names with.
void print_standard_includes() override
Print standard C/C++ includes.
std::vector< ThreadVariableInfo > codegen_thread_variables
GLOBAL variables in THREADSAFE MOD files that are not read-only are converted to thread variables.
void print_mechanism_range_var_structure(bool print_initializers) override
Print the structure that wraps all range and int variables required for the NMODL.
Extracts information required for LONGITUDINAL_DIFFUSION for each KINETIC block.
void add_variable_tqitem(std::vector< IndexVariableInfo > &variables) override
Add the variable tqitem during get_int_variables.
Helper class for printing C/C++ code.
std::string hoc_function_signature(const std::string &function_or_procedure_name) const
Get the signature of the hoc <func_or_proc_name> function.
std::pair< ParamVector, ParamVector > function_table_parameters(const ast::FunctionTableBlock &) override
Parameters of the function itself "{}" and "table_{}".
void print_thread_variables_structure(bool print_initializers)
Print the data structure used to access thread variables.
void visit_longitudinal_diffusion_block(const ast::LongitudinalDiffusionBlock &node) override
visit node of type ast::LongitudinalDiffusionBlock
void print_function_table_call(const ast::FunctionCall &node) override
Print special code when calling FUNCTION_TABLEs.
void print_compute_functions() override
Print all compute functions for every backend.
void print_thread_memory_callbacks()
Print thread variable (de-)initialization functions.
void print_entrypoint_setup_code_from_memb_list()
Prints setup code for entrypoints from NEURON.
void visit_verbatim(const ast::Verbatim &node) override
visit node of type ast::Verbatim
void print_nrn_cur_kernel(const ast::BreakpointBlock &node) override
Print main body of nrn_cur function.
void print_mechanism_register() override
Print the mechanism registration function.
void print_nrn_jacob()
Print nrn_jacob function definition.
void print_net_receive_common_code()
Various types to store code generation specific information.
void print_fast_imem_calculation() override
Print fast membrane current calculation code.
std::vector< std::string > print_verbatim_setup(const ast::Verbatim &node, const std::string &verbatim)
Print compatibility macros required for VERBATIM blocks.
void print_data_structures(bool print_initializers) override
Print all classes.
Auto generated AST classes declaration.
void print_sdlists_init(bool print_initializers) override
const std::string external_method_arguments() noexcept override
Arguments for external functions called from generated code.
Represent LONGITUDINAL_DIFFUSION statement in NMODL.
std::string py_function_name(const std::string &function_or_procedure_name) const
In non POINT_PROCESS mechanisms all functions and procedures need a py <func_or_proc_name> to be avai...
size_t offset
The global variables ahead of this one require offset doubles to store.
void print_node_data_structure(bool print_initializers)
Print the structure that wraps all node variables required for the NMODL.
void visit_protect_statement(const ast::ProtectStatement &node) override
visit node of type ast::ProtectStatement
void print_cvode_update(const std::string &name, const ast::StatementBlock &block)
Print the CVODE update function name contained in block.
void print_make_instance() const
Print make_*_instance.
Represents block encapsulating list of statements.
void print_setdata_functions()
Print NEURON functions related to setting global variables of the mechanism.
Represents ion write statement during code generation.
void print_mechanism_variables_macros()
Print mechanism variables' related macros.
Visitor for printing C++ code compatible with legacy api of NEURON
ParamVector threadargs_parameters()
The parameters for the four macros _threadargs*_.
void print_nrn_constructor() override
Print nrn_constructor function definition.
Implement logger based on spdlog library.
parser::NmodlParser::symbol_type SymbolType
BlockType
Helper to represent various block types.
size_t index
There index global variables ahead of this one.
void print_headers_include() override
Print all includes.
void print_check_table_entrypoint()
Print all check_* function declarations.
const ParamVector external_method_parameters(bool table=false) noexcept override
Parameters for functions in generated code that are called back from external code.
void visit_for_netcon(const ast::ForNetcon &node) override
visit node of type ast::ForNetcon
void print_function_or_procedure(const ast::Block &node, const std::string &name, const std::unordered_set< CppObjectSpecifier > &specifiers={ CppObjectSpecifier::Inline}) override
Print nmodl function or procedure (common code)
const std::shared_ptr< symtab::Symbol > symbol
void print_nrn_current(const ast::BreakpointBlock &node) override
Print the nrn_current kernel.
int position_of_float_var(const std::string &name) const override
Determine the position in the data array for a given float variable.
std::string get_variable_name(const std::string &name, bool use_instance=true) const override
Determine the C++ string to replace variable names with.
void print_hoc_py_wrapper_call_impl(const ast::Block *function_or_procedure_block, InterpreterWrapper wrapper_type)
Print the code that calls the impl from the HOC/Py wrapper.
void print_codegen_routines_nothing()
SUFFIX nothing is special.
Visitor for printing C++ code compatible with legacy api of CoreNEURON
CodegenCppVisitor(std::string mod_filename, std::ostream &stream, std::string float_type, const bool optimize_ionvar_copies, std::unique_ptr< nmodl::utils::Blame > blame=nullptr)
Constructs the C++ code generator visitor.
void print_make_node_data() const
Print make_*_node_data.
std::string simulator_name() override
Name of the simulator the code was generated for.
void print_function_procedure_helper(const ast::Block &node) override
Common helper function to help printing function or procedure blocks.
std::string process_verbatim_text(const std::string &verbatim)
void add_variable_point_process(std::vector< IndexVariableInfo > &variables) override
Add the variable point_process during get_int_variables.
std::string nrn_thread_arguments() const override
Arguments for "_threadargs_" macro in neuron implementation.
ParamVector ldifusfunc3_parameters() const
Parameters for what NEURON calls ldifusfunc3_t.
ParamVector cvode_update_parameters()
Get the parameters for functions that update state at given timestep in CVODE.
void print_net_receive_registration()
Print code to register the call-back for the NET_RECEIVE block.
void print_codegen_routines() override
Print entry point to code generation.
void append_conc_write_statements(std::vector< ShadowUseStatement > &statements, const Ion &ion, const std::string &concentration) override
Generate Function call statement for nrn_wrote_conc.
void print_longitudinal_diffusion_callbacks()
Prints the callbacks required for LONGITUDINAL_DIFFUSION.
ParamVector internal_method_parameters() override
Parameters for internally defined functions.
void print_hoc_py_wrapper(const ast::Block *function_or_procedure_block, InterpreterWrapper wrapper_type)
Print the wrapper for calling FUNCION/PROCEDURES from HOC/Py.
void print_cvode_count()
Print the CVODE function returning the number of ODEs to solve.
ParamVector net_receive_args()
void print_net_receive()
Print net_receive call-back.
void print_function_prototypes() override
Print function and procedures prototype declaration.
void print_mechanism_global_var_structure(bool print_initializers) override
Print the structure that wraps all global variables used in the NMODL.
void print_hoc_py_wrapper_setup(const ast::Block *function_or_procedure_block, InterpreterWrapper wrapper_type)
Print the setup code for HOC/Py wrapper.
std::string namespace_name() override
Name of "our" namespace.
void print_global_macros()
Print NEURON global variable macros.
std::vector< std::tuple< std::string, std::string, std::string, std::string > > ParamVector
A vector of parameters represented by a 4-tuple of strings:
Concrete visitor for all AST classes.