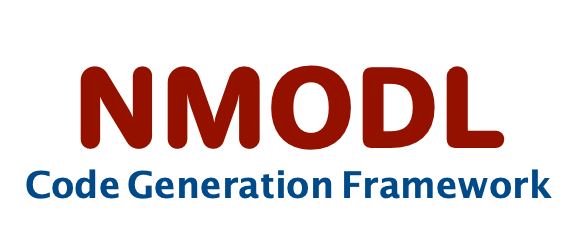 |
User Guide
|
Concrete constant visitor for all AST classes.
void visit_function_block(const ast::FunctionBlock &node) override
visit node of type ast::FunctionBlock
void visit_program(const ast::Program &node) override
visit node of type ast::Program
encapsulates code generation backend implementations
std::vector< const ast::Block * > visited_functions_or_procedures
Vector of currently visited functions or procedures (used as a searchable stack)
Visitor for traversing FunctionBlock s and ProcedureBlocks through their FunctionCall s
Represent symbol table for a NMODL block.
symtab::SymbolTable * psymtab
symbol table for the program
void visit_var_name(const ast::VarName &node) override
visit node of type ast::VarName
void visit_function_call(const ast::FunctionCall &node) override
visit node of type ast::FunctionCall
Represents top level AST node for whole NMODL input.
Forward declarations of symbols in namespace nmodl::symtab.
void visit_procedure_block(const ast::ProcedureBlock &node) override
visit node of type ast::ProcedureBlock
Auto generated AST classes declaration.
Concrete visitor for all AST classes.