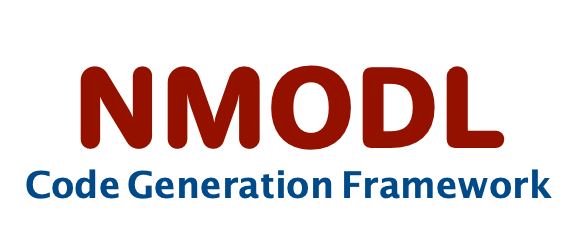 |
User Guide
|
Go to the documentation of this file.
25 const auto properties = NmodlType::range_var | NmodlType::pointer_var |
26 NmodlType::bbcore_pointer_var;
27 if (sym && sym->has_any_property(properties)) {
29 const auto caller_func_name =
30 top->is_function_block()
33 auto caller_func_proc_sym =
psymtab->
lookup(caller_func_name);
34 caller_func_proc_sym->add_properties(NmodlType::use_range_ptr_var);
45 !func_symbol->has_any_property(NmodlType::function_block | NmodlType::procedure_block) ||
46 func_symbol->get_nodes().empty()) {
52 const auto func_block = func_symbol->get_nodes()[0];
53 func_block->accept(*
this);
54 if (func_symbol->has_any_property(NmodlType::use_range_ptr_var)) {
56 auto caller_func_name =
57 top->is_function_block()
60 auto caller_func_proc_sym =
psymtab->
lookup(caller_func_name);
61 caller_func_proc_sym->add_properties(NmodlType::use_range_ptr_var);
std::string get_node_name() const override
Return name of the node.
void visit_function_block(const ast::FunctionBlock &node) override
visit node of type ast::FunctionBlock
void visit_program(const ast::Program &node) override
visit node of type ast::Program
encapsulates code generation backend implementations
std::vector< const ast::Block * > visited_functions_or_procedures
Vector of currently visited functions or procedures (used as a searchable stack)
void visit_children(visitor::Visitor &v) override
visit children i.e.
void visit_children(visitor::Visitor &v) override
visit children i.e.
void visit_children(visitor::Visitor &v) override
visit children i.e.
symtab::SymbolTable * psymtab
symbol table for the program
NmodlType
NMODL variable properties.
Visitor for traversing FunctionBlock s and ProcedureBlocks through their FunctionCall s
void visit_var_name(const ast::VarName &node) override
visit node of type ast::VarName
symtab::SymbolTable * get_symbol_table() const override
Return associated symbol table for the current ast node.
void visit_function_call(const ast::FunctionCall &node) override
visit node of type ast::FunctionCall
Represents top level AST node for whole NMODL input.
std::shared_ptr< Symbol > lookup(const std::string &name) const
check if symbol with given name exist in the current table (but not in parents)
void visit_procedure_block(const ast::ProcedureBlock &node) override
visit node of type ast::ProcedureBlock
std::string get_node_name() const override
Return name of the node.