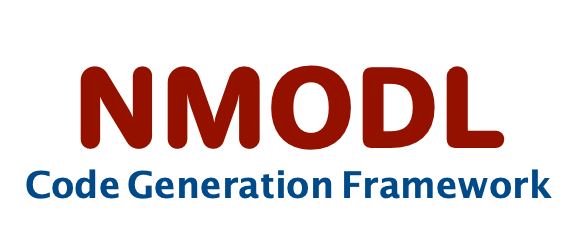 |
User Guide
|
Go to the documentation of this file.
74 std::vector<std::shared_ptr<Symbol>>
symbols;
77 void insert(
const std::shared_ptr<Symbol>& symbol);
80 std::shared_ptr<Symbol>
lookup(
const std::string&
name)
const;
83 void print(std::ostream& stream, std::string
title,
int indent)
const;
107 std::map<std::string, std::shared_ptr<SymbolTable>>
children;
156 bool all =
false)
const;
159 bool all =
false)
const;
165 std::ostringstream s;
170 const std::string&
name() const noexcept {
178 void insert(
const std::shared_ptr<Symbol>& symbol) {
199 std::shared_ptr<Symbol>
lookup(
const std::string&
name)
const {
212 void print(std::ostream& ss,
int level)
const;
214 std::string
title()
const;
263 const std::shared_ptr<Symbol>& second,
266 void update_order(
const std::shared_ptr<Symbol>& present_symbol,
267 const std::shared_ptr<Symbol>& new_symbol);
280 std::shared_ptr<Symbol>
insert(
const std::shared_ptr<Symbol>& symbol);
283 std::shared_ptr<Symbol>
lookup(
const std::string& name);
290 void print(std::ostream& ostr)
const {
SymbolTable * parent
pointer to the symbol table of parent block in the mod file
std::shared_ptr< Symbol > lookup(const std::string &name) const
check if symbol with given name exist
std::string to_string() const
convert symbol table to string
Implement class to represent a symbol in Symbol Table.
Base class for all Abstract Syntax Tree node types.
bool global_scope() const noexcept
void set_parent_table(SymbolTable *block)
SymbolTable * get_parent_table() const noexcept
std::shared_ptr< Symbol > insert(const std::shared_ptr< Symbol > &symbol)
insert new symbol into current table
SymbolTable(std::string name, const ast::Ast *node, bool global=false)
std::shared_ptr< SymbolTable > symtab
symbol table for mod file (always top level symbol table)
const std::string symtab_name
name of the block
void insert(const std::shared_ptr< Symbol > &symbol)
insert new symbol into table
encapsulates code generation backend implementations
const std::string & name() const noexcept
void print(std::ostream &ostr) const
pretty print
std::vector< std::shared_ptr< Symbol > > get_variables_with_properties(syminfo::NmodlType properties, bool all=false) const
get variables with properties
std::string position() const
void leave_scope()
leaving current nmodl block
Status
state during various compiler passes
SymbolTable * clone() const
Create a copy of symbol table.
int definition_order
current order of variable being defined
std::vector< std::shared_ptr< Symbol > > get_variables(syminfo::NmodlType with=syminfo::NmodlType::empty, syminfo::NmodlType without=syminfo::NmodlType::empty) const
get variables
void emit_message(const std::shared_ptr< Symbol > &first, const std::shared_ptr< Symbol > &second, bool redefinition)
std::map< std::string, std::shared_ptr< SymbolTable > > children
symbol table for each enclosing block in the current nmodl block construct.
bool is_method_defined(const std::string &name) const
check if procedure/function with given name is defined
std::string title() const
construct title for symbol table
std::shared_ptr< Symbol > update_mode_insert(const std::shared_ptr< Symbol > &symbol)
insert symbol table in update mode
SymbolTable * current_symtab
current symbol table being constructed
void insert_table(const std::string &name, const std::shared_ptr< SymbolTable > &table)
insert new symbol table as one of the children block
std::shared_ptr< Symbol > lookup(const std::string &name)
lookup for symbol into current as well as all parent tables
std::vector< std::shared_ptr< Symbol > > get_variables_with_status(syminfo::Status status, bool all=false) const
Helper class for implementing symbol table.
std::string get_parent_table_name() const
Represent symbol table for a NMODL block.
bool global
true if current symbol table is global.
void insert(const std::shared_ptr< Symbol > &symbol)
void print(std::ostream &stream, std::string title, int indent) const
pretty print
SymbolTable * enter_scope(const std::string &name, ast::Ast *node, bool global, SymbolTable *node_symtab)
entering into new nmodl block
const ast::Ast * node
pointer to ast node for which current symbol table created
void set_mode(bool update_mode)
re-initialize members to throw away old symbol tables this is required as symtab visitor pass runs mu...
NmodlType
NMODL variable properties.
const std::string GLOBAL_SYMTAB_NAME
name of top level global symbol table
static int counter
number of tables
void update_order(const std::shared_ptr< Symbol > &present_symbol, const std::shared_ptr< Symbol > &new_symbol)
void print(std::ostream &ss, int level) const
std::shared_ptr< Symbol > lookup(const std::string &name) const
check if symbol with given name exist in the current table (but not in parents)
Table table
table holding all symbols in the current block
bool update_table
default mode of symbol table: if update is true then we update exisiting symbols otherwise we throw a...
std::string get_unique_name(const std::string &name, ast::Ast *node, bool is_global)
return unique name by appending some counter value
std::shared_ptr< Symbol > lookup_in_scope(const std::string &name) const
check if symbol with given name exist in the current table (including all parents)
std::vector< std::shared_ptr< Symbol > > symbols
map of symbol name and associated symbol for faster lookup
bool under_global_scope()
check if currently we are visiting global scope node