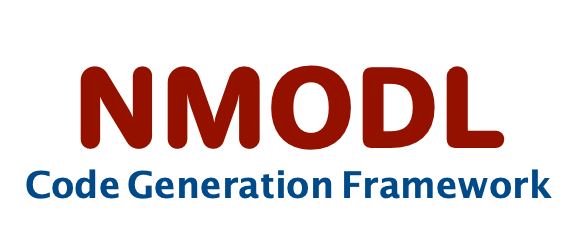 |
User Guide
|
Go to the documentation of this file.
24 #include <unordered_set>
69 struct Ast:
public std::enable_shared_from_this<Ast> {
101 virtual ~Ast() =
default;
142 throw std::runtime_error(
"get_nmodl_name not implemented");
297 virtual void set_name(
const std::string& name);
320 virtual bool is_ast() const noexcept;
326 virtual
bool is_node () const noexcept;
344 virtual
bool is_block () const noexcept;
356 virtual
bool is_number () const noexcept;
362 virtual
bool is_string () const noexcept;
374 virtual
bool is_float () const noexcept;
380 virtual
bool is_double () const noexcept;
392 virtual
bool is_name () const noexcept;
644 virtual
bool is_unit () const noexcept;
662 virtual
bool is_limits () const noexcept;
740 virtual
bool is_watch () const noexcept;
782 virtual
bool is_model () const noexcept;
788 virtual
bool is_define () const noexcept;
920 virtual
bool is_suffix () const noexcept;
926 virtual
bool is_useion () const noexcept;
944 virtual
bool is_range () const noexcept;
950 virtual
bool is_global () const noexcept;
virtual std::string get_nmodl_name() const
Return NMODL statement of ast node as std::string.
virtual bool is_argument() const noexcept
Check if the ast node is an instance of ast::Argument.
Abstract base class for all constant visitors implementation.
virtual bool is_external() const noexcept
Check if the ast node is an instance of ast::External.
virtual bool is_double_unit() const noexcept
Check if the ast node is an instance of ast::DoubleUnit.
virtual bool is_kinetic_block() const noexcept
Check if the ast node is an instance of ast::KineticBlock.
virtual bool is_local_list_statement() const noexcept
Check if the ast node is an instance of ast::LocalListStatement.
virtual bool is_statement() const noexcept
Check if the ast node is an instance of ast::Statement.
virtual bool is_block() const noexcept
Check if the ast node is an instance of ast::Block.
virtual bool is_unit_block() const noexcept
Check if the ast node is an instance of ast::UnitBlock.
virtual bool is_range() const noexcept
Check if the ast node is an instance of ast::Range.
virtual bool is_net_receive_block() const noexcept
Check if the ast node is an instance of ast::NetReceiveBlock.
Base class for all Abstract Syntax Tree node types.
virtual bool is_paren_expression() const noexcept
Check if the ast node is an instance of ast::ParenExpression.
virtual bool is_string() const noexcept
Check if the ast node is an instance of ast::String.
virtual bool is_non_linear_block() const noexcept
Check if the ast node is an instance of ast::NonLinearBlock.
virtual bool is_eigen_newton_solver_block() const noexcept
Check if the ast node is an instance of ast::EigenNewtonSolverBlock.
virtual bool is_local_var() const noexcept
Check if the ast node is an instance of ast::LocalVar.
virtual bool is_ontology_statement() const noexcept
Check if the ast node is an instance of ast::OntologyStatement.
virtual bool is_global() const noexcept
Check if the ast node is an instance of ast::Global.
virtual bool is_conserve() const noexcept
Check if the ast node is an instance of ast::Conserve.
virtual bool is_destructor_block() const noexcept
Check if the ast node is an instance of ast::DestructorBlock.
virtual bool is_conductance_hint() const noexcept
Check if the ast node is an instance of ast::ConductanceHint.
virtual bool is_function_call() const noexcept
Check if the ast node is an instance of ast::FunctionCall.
virtual bool is_suffix() const noexcept
Check if the ast node is an instance of ast::Suffix.
THIS FILE IS GENERATED AT BUILD TIME AND SHALL NOT BE EDITED.
virtual bool is_assigned_block() const noexcept
Check if the ast node is an instance of ast::AssignedBlock.
virtual bool is_integer() const noexcept
Check if the ast node is an instance of ast::Integer.
virtual bool is_bbcore_pointer() const noexcept
Check if the ast node is an instance of ast::BbcorePointer.
virtual bool is_pointer() const noexcept
Check if the ast node is an instance of ast::Pointer.
virtual bool is_procedure_block() const noexcept
Check if the ast node is an instance of ast::ProcedureBlock.
virtual bool is_protect_statement() const noexcept
Check if the ast node is an instance of ast::ProtectStatement.
virtual bool is_var_name() const noexcept
Check if the ast node is an instance of ast::VarName.
Abstract Syntax Tree (AST) related implementations.
virtual bool is_expression_statement() const noexcept
Check if the ast node is an instance of ast::ExpressionStatement.
virtual bool is_extern_var() const noexcept
Check if the ast node is an instance of ast::ExternVar.
virtual bool is_function_block() const noexcept
Check if the ast node is an instance of ast::FunctionBlock.
virtual bool is_constant_var() const noexcept
Check if the ast node is an instance of ast::ConstantVar.
virtual bool is_reaction_statement() const noexcept
Check if the ast node is an instance of ast::ReactionStatement.
virtual bool is_global_var() const noexcept
Check if the ast node is an instance of ast::GlobalVar.
AstNodeType
Enum type for every AST node type.
Implementation of AST base class and it's properties.
virtual bool is_nrn_state_block() const noexcept
Check if the ast node is an instance of ast::NrnStateBlock.
virtual bool is_assigned_definition() const noexcept
Check if the ast node is an instance of ast::AssignedDefinition.
virtual void set_parent(Ast *p)
Parent setter.
virtual bool is_random_var_list() const noexcept
Check if the ast node is an instance of ast::RandomVarList.
virtual bool is_function_table_block() const noexcept
Check if the ast node is an instance of ast::FunctionTableBlock.
virtual bool is_mutex_lock() const noexcept
Check if the ast node is an instance of ast::MutexLock.
virtual bool is_lag_statement() const noexcept
Check if the ast node is an instance of ast::LagStatement.
virtual bool is_ba_block_type() const noexcept
Check if the ast node is an instance of ast::BABlockType.
virtual bool is_if_statement() const noexcept
Check if the ast node is an instance of ast::IfStatement.
virtual void set_name(const std::string &name)
Set name for the AST node.
virtual bool is_diff_eq_expression() const noexcept
Check if the ast node is an instance of ast::DiffEqExpression.
virtual bool is_discrete_block() const noexcept
Check if the ast node is an instance of ast::DiscreteBlock.
virtual bool is_name() const noexcept
Check if the ast node is an instance of ast::Name.
virtual bool is_verbatim() const noexcept
Check if the ast node is an instance of ast::Verbatim.
virtual std::string get_node_type_name() const =0
Return type (ast::AstNodeType) of ast node as std::string.
virtual symtab::SymbolTable * get_symbol_table() const
Return associated symbol table for the AST node.
virtual bool is_update_dt() const noexcept
Check if the ast node is an instance of ast::UpdateDt.
Abstract base class for all visitors implementation.
virtual bool is_pointer_var() const noexcept
Check if the ast node is an instance of ast::PointerVar.
virtual bool is_valence() const noexcept
Check if the ast node is an instance of ast::Valence.
virtual bool is_else_if_statement() const noexcept
Check if the ast node is an instance of ast::ElseIfStatement.
virtual bool is_table_statement() const noexcept
Check if the ast node is an instance of ast::TableStatement.
virtual bool is_unit() const noexcept
Check if the ast node is an instance of ast::Unit.
virtual bool is_thread_safe() const noexcept
Check if the ast node is an instance of ast::ThreadSafe.
virtual bool is_longitudinal_diffusion_block() const noexcept
Check if the ast node is an instance of ast::LongitudinalDiffusionBlock.
virtual bool is_double() const noexcept
Check if the ast node is an instance of ast::Double.
virtual Ast * clone() const
Create a copy of the current node.
virtual bool is_number() const noexcept
Check if the ast node is an instance of ast::Number.
virtual bool is_model() const noexcept
Check if the ast node is an instance of ast::Model.
virtual void negate()
Negate the value of AST node.
virtual bool is_ast() const noexcept
Check if the ast node is an instance of ast::Ast.
virtual bool is_block_comment() const noexcept
Check if the ast node is an instance of ast::BlockComment.
virtual bool is_initial_block() const noexcept
Check if the ast node is an instance of ast::InitialBlock.
virtual bool is_write_ion_var() const noexcept
Check if the ast node is an instance of ast::WriteIonVar.
virtual bool is_bbcore_pointer_var() const noexcept
Check if the ast node is an instance of ast::BbcorePointerVar.
virtual bool is_electrode_cur_var() const noexcept
Check if the ast node is an instance of ast::ElectrodeCurVar.
virtual bool is_watch_statement() const noexcept
Check if the ast node is an instance of ast::WatchStatement.
virtual bool is_random_var() const noexcept
Check if the ast node is an instance of ast::RandomVar.
virtual bool is_constant_statement() const noexcept
Check if the ast node is an instance of ast::ConstantStatement.
virtual bool is_from_statement() const noexcept
Check if the ast node is an instance of ast::FromStatement.
virtual bool is_state_block() const noexcept
Check if the ast node is an instance of ast::StateBlock.
virtual const ModToken * get_token() const
Return associated token for the AST node.
virtual bool is_neuron_block() const noexcept
Check if the ast node is an instance of ast::NeuronBlock.
virtual void accept(visitor::Visitor &v)=0
Accept (or visit) the AST node using current visitor.
virtual bool is_watch() const noexcept
Check if the ast node is an instance of ast::Watch.
Represent symbol table for a NMODL block.
virtual bool is_mutex_unlock() const noexcept
Check if the ast node is an instance of ast::MutexUnlock.
virtual bool is_breakpoint_block() const noexcept
Check if the ast node is an instance of ast::BreakpointBlock.
virtual bool is_wrapped_expression() const noexcept
Check if the ast node is an instance of ast::WrappedExpression.
virtual bool is_binary_expression() const noexcept
Check if the ast node is an instance of ast::BinaryExpression.
virtual bool is_expression() const noexcept
Check if the ast node is an instance of ast::Expression.
virtual bool is_react_var_name() const noexcept
Check if the ast node is an instance of ast::ReactVarName.
virtual bool is_compartment() const noexcept
Check if the ast node is an instance of ast::Compartment.
virtual bool is_factor_def() const noexcept
Check if the ast node is an instance of ast::FactorDef.
virtual bool is_reaction_operator() const noexcept
Check if the ast node is an instance of ast::ReactionOperator.
virtual void set_symbol_table(symtab::SymbolTable *symtab)
Set symbol table for the AST node.
virtual bool is_lin_equation() const noexcept
Check if the ast node is an instance of ast::LinEquation.
virtual bool is_define() const noexcept
Check if the ast node is an instance of ast::Define.
virtual bool is_statement_block() const noexcept
Check if the ast node is an instance of ast::StatementBlock.
virtual bool is_non_lin_equation() const noexcept
Check if the ast node is an instance of ast::NonLinEquation.
virtual bool is_indexed_name() const noexcept
Check if the ast node is an instance of ast::IndexedName.
virtual std::shared_ptr< Ast > get_shared_ptr()
get std::shared_ptr from this pointer of the AST node
virtual bool is_unit_state() const noexcept
Check if the ast node is an instance of ast::UnitState.
virtual bool is_include() const noexcept
Check if the ast node is an instance of ast::Include.
virtual bool is_param_assign() const noexcept
Check if the ast node is an instance of ast::ParamAssign.
virtual bool is_node() const noexcept
Check if the ast node is an instance of ast::Node.
Ast * parent
Generic pointer to the parent.
virtual bool is_lon_diffuse() const noexcept
Check if the ast node is an instance of ast::LonDiffuse.
virtual bool is_ba_block() const noexcept
Check if the ast node is an instance of ast::BABlock.
virtual bool is_number_range() const noexcept
Check if the ast node is an instance of ast::NumberRange.
virtual bool is_unary_expression() const noexcept
Check if the ast node is an instance of ast::UnaryExpression.
virtual bool is_unit_def() const noexcept
Check if the ast node is an instance of ast::UnitDef.
virtual bool is_linear_block() const noexcept
Check if the ast node is an instance of ast::LinearBlock.
virtual bool is_param_block() const noexcept
Check if the ast node is an instance of ast::ParamBlock.
virtual bool is_prime_name() const noexcept
Check if the ast node is an instance of ast::PrimeName.
virtual bool is_solution_expression() const noexcept
Check if the ast node is an instance of ast::SolutionExpression.
virtual bool is_limits() const noexcept
Check if the ast node is an instance of ast::Limits.
virtual bool is_eigen_linear_solver_block() const noexcept
Check if the ast node is an instance of ast::EigenLinearSolverBlock.
virtual bool is_program() const noexcept
Check if the ast node is an instance of ast::Program.
virtual bool is_cvode_block() const noexcept
Check if the ast node is an instance of ast::CvodeBlock.
virtual bool is_independent_block() const noexcept
Check if the ast node is an instance of ast::IndependentBlock.
virtual bool is_solve_block() const noexcept
Check if the ast node is an instance of ast::SolveBlock.
virtual bool is_identifier() const noexcept
Check if the ast node is an instance of ast::Identifier.
virtual bool is_line_comment() const noexcept
Check if the ast node is an instance of ast::LineComment.
virtual bool is_while_statement() const noexcept
Check if the ast node is an instance of ast::WhileStatement.
virtual std::shared_ptr< StatementBlock > get_statement_block() const
Return associated statement block for the AST node.
virtual bool is_else_statement() const noexcept
Check if the ast node is an instance of ast::ElseStatement.
virtual bool is_nonspecific_cur_var() const noexcept
Check if the ast node is an instance of ast::NonspecificCurVar.
virtual bool is_derivimplicit_callback() const noexcept
Check if the ast node is an instance of ast::DerivimplicitCallback.
virtual bool is_before_block() const noexcept
Check if the ast node is an instance of ast::BeforeBlock.
virtual bool is_electrode_current() const noexcept
Check if the ast node is an instance of ast::ElectrodeCurrent.
virtual bool is_binary_operator() const noexcept
Check if the ast node is an instance of ast::BinaryOperator.
virtual bool is_constant_block() const noexcept
Check if the ast node is an instance of ast::ConstantBlock.
virtual void visit_children(visitor::Visitor &v)=0
Visit children i.e.
virtual AstNodeType get_node_type() const =0
Return type (ast::AstNodeType) of AST node.
virtual bool is_nonspecific() const noexcept
Check if the ast node is an instance of ast::Nonspecific.
virtual bool is_after_block() const noexcept
Check if the ast node is an instance of ast::AfterBlock.
virtual bool is_for_netcon() const noexcept
Check if the ast node is an instance of ast::ForNetcon.
virtual bool is_float() const noexcept
Check if the ast node is an instance of ast::Float.
Represent token returned by scanner.
virtual bool is_derivative_block() const noexcept
Check if the ast node is an instance of ast::DerivativeBlock.
virtual bool is_boolean() const noexcept
Check if the ast node is an instance of ast::Boolean.
virtual Ast * get_parent() const
Parent getter.
virtual bool is_unary_operator() const noexcept
Check if the ast node is an instance of ast::UnaryOperator.
virtual std::string get_node_name() const
Return name of of the node.
Common utility functions for file/dir manipulation.
virtual bool is_range_var() const noexcept
Check if the ast node is an instance of ast::RangeVar.
virtual bool is_useion() const noexcept
Check if the ast node is an instance of ast::Useion.
virtual bool is_read_ion_var() const noexcept
Check if the ast node is an instance of ast::ReadIonVar.
virtual bool is_constructor_block() const noexcept
Check if the ast node is an instance of ast::ConstructorBlock.