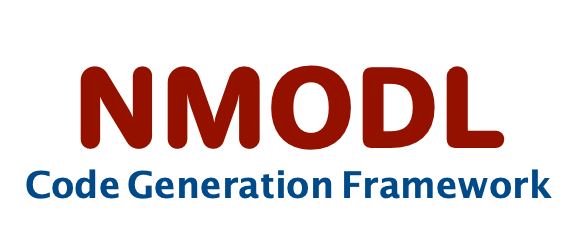 |
User Guide
|
Go to the documentation of this file.
26 Ast *
Ast::clone()
const {
throw std::logic_error(
"clone not implemented"); }
29 throw std::logic_error(
"get_node_name() not implemented");
33 throw std::runtime_error(
"get_statement_block not implemented");
39 throw std::runtime_error(
"get_symbol_table not implemented");
43 throw std::runtime_error(
"set_symbol_table not implemented");
47 throw std::runtime_error(
"set_name not implemented");
50 void Ast::negate() {
throw std::runtime_error(
"negate not implemented"); }
53 return std::static_pointer_cast<Ast>(shared_from_this());
57 return std::static_pointer_cast<const Ast>(shared_from_this());
488 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
526 this->
macro->accept(v);
534 this->
macro->accept(v);
567 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
580 macro->set_parent(
this);
598 macro->set_parent(
this);
606 macro->set_parent(
this);
647 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
706 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
765 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
796 return value->eval();
837 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
853 value->set_parent(
this);
864 value->set_parent(
this);
872 value->set_parent(
this);
885 return value->eval();
939 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
952 value->set_parent(
this);
956 order->set_parent(
this);
967 value->set_parent(
this);
975 value->set_parent(
this);
984 order->set_parent(
this);
992 order->set_parent(
this);
1005 return name->get_node_name();
1050 this->
name.reset(obj.
name->clone());
1059 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
1072 name->set_parent(
this);
1076 length->set_parent(
this);
1087 name->set_parent(
this);
1095 name->set_parent(
this);
1104 length->set_parent(
this);
1112 length->set_parent(
this);
1125 return name->get_node_name();
1140 this->
at->accept(v);
1145 this->
index->accept(v);
1156 this->
at->accept(v);
1161 this->
index->accept(v);
1179 VarName::VarName(std::shared_ptr<Identifier> name, std::shared_ptr<Integer> at, std::shared_ptr<Expression> index)
1187 this->
name.reset(obj.
name->clone());
1191 this->
at.reset(obj.
at->clone());
1200 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
1213 name->set_parent(
this);
1217 at->set_parent(
this);
1221 index->set_parent(
this);
1232 name->set_parent(
this);
1240 name->set_parent(
this);
1249 at->set_parent(
this);
1257 at->set_parent(
this);
1266 index->set_parent(
this);
1271 this->index =
index;
1274 index->set_parent(
this);
1287 return name->get_node_name();
1299 this->
unit->accept(v);
1310 this->
unit->accept(v);
1336 this->
name.reset(obj.
name->clone());
1340 this->
unit.reset(obj.
unit->clone());
1345 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
1358 name->set_parent(
this);
1362 unit->set_parent(
this);
1373 name->set_parent(
this);
1381 name->set_parent(
this);
1390 unit->set_parent(
this);
1398 unit->set_parent(
this);
1414 return name->get_node_name();
1420 this->
value->accept(v);
1431 this->
value->accept(v);
1464 this->
name.reset(obj.
name->clone());
1469 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
1482 value->set_parent(
this);
1486 name->set_parent(
this);
1497 value->set_parent(
this);
1502 this->value =
value;
1505 value->set_parent(
this);
1514 name->set_parent(
this);
1522 name->set_parent(
this);
1535 return name->get_node_name();
1571 this->
name.reset(obj.
name->clone());
1576 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
1589 name->set_parent(
this);
1600 name->set_parent(
this);
1608 name->set_parent(
this);
1621 return name->get_node_name();
1657 this->
name.reset(obj.
name->clone());
1662 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
1675 name->set_parent(
this);
1686 name->set_parent(
this);
1694 name->set_parent(
this);
1707 return name->get_node_name();
1743 this->
name.reset(obj.
name->clone());
1748 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
1761 name->set_parent(
this);
1772 name->set_parent(
this);
1780 name->set_parent(
this);
1793 return name->get_node_name();
1829 this->
name.reset(obj.
name->clone());
1834 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
1847 name->set_parent(
this);
1858 name->set_parent(
this);
1866 name->set_parent(
this);
1879 return name->get_node_name();
1915 this->
name.reset(obj.
name->clone());
1920 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
1933 name->set_parent(
this);
1944 name->set_parent(
this);
1952 name->set_parent(
this);
1965 return name->get_node_name();
2001 this->
name.reset(obj.
name->clone());
2006 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
2019 name->set_parent(
this);
2030 name->set_parent(
this);
2038 name->set_parent(
this);
2051 return name->get_node_name();
2087 this->
name.reset(obj.
name->clone());
2092 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
2105 name->set_parent(
this);
2116 name->set_parent(
this);
2124 name->set_parent(
this);
2137 return name->get_node_name();
2173 this->
name.reset(obj.
name->clone());
2178 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
2191 name->set_parent(
this);
2202 name->set_parent(
this);
2210 name->set_parent(
this);
2223 return name->get_node_name();
2259 this->
name.reset(obj.
name->clone());
2264 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
2277 name->set_parent(
this);
2288 name->set_parent(
this);
2296 name->set_parent(
this);
2309 return name->get_node_name();
2345 this->
name.reset(obj.
name->clone());
2350 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
2363 name->set_parent(
this);
2374 name->set_parent(
this);
2382 name->set_parent(
this);
2430 this->
statements.emplace_back(item->clone());
2435 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
2448 item->set_parent(
this);
2460 ii->set_parent(
this);
2468 ii->set_parent(
this);
2516 this->
variables.emplace_back(item->clone());
2521 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
2534 item->set_parent(
this);
2546 ii->set_parent(
this);
2554 ii->set_parent(
this);
2581 n->set_parent(
this);
2604 auto result = first;
2606 while (first != last) {
2609 if (to_be_erased.erase(first->get()) == 0) {
2616 size_t out = last - result;
2626 n->set_parent(
this);
2645 n->set_parent(
this);
2692 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
2705 item->set_parent(
this);
2717 ii->set_parent(
this);
2725 ii->set_parent(
this);
2778 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
2791 item->set_parent(
this);
2803 ii->set_parent(
this);
2811 ii->set_parent(
this);
2863 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
2947 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
3031 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
3090 n->set_parent(
this);
3113 auto result = first;
3115 while (first != last) {
3118 if (to_be_erased.erase(first->get()) == 0) {
3125 size_t out = last - result;
3135 n->set_parent(
this);
3154 n->set_parent(
this);
3196 this->
statements.emplace_back(item->clone());
3201 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
3214 item->set_parent(
this);
3226 ii->set_parent(
this);
3234 ii->set_parent(
this);
3247 return name->get_node_name();
3292 this->
name.reset(obj.
name->clone());
3301 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
3314 name->set_parent(
this);
3329 name->set_parent(
this);
3337 name->set_parent(
this);
3367 return name->get_node_name();
3381 for (
auto& item : this->
solvefor) {
3395 for (
auto& item : this->
solvefor) {
3425 this->
name.reset(obj.
name->clone());
3429 this->
solvefor.emplace_back(item->clone());
3438 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
3451 name->set_parent(
this);
3455 item->set_parent(
this);
3471 name->set_parent(
this);
3479 name->set_parent(
this);
3488 ii->set_parent(
this);
3496 ii->set_parent(
this);
3526 return name->get_node_name();
3540 for (
auto& item : this->
solvefor) {
3554 for (
auto& item : this->
solvefor) {
3584 this->
name.reset(obj.
name->clone());
3588 this->
solvefor.emplace_back(item->clone());
3597 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
3610 name->set_parent(
this);
3614 item->set_parent(
this);
3630 name->set_parent(
this);
3638 name->set_parent(
this);
3647 ii->set_parent(
this);
3655 ii->set_parent(
this);
3685 return name->get_node_name();
3730 this->
name.reset(obj.
name->clone());
3739 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
3752 name->set_parent(
this);
3767 name->set_parent(
this);
3775 name->set_parent(
this);
3805 return name->get_node_name();
3825 this->
unit->accept(v);
3841 this->
unit->accept(v);
3867 this->
name.reset(obj.
name->clone());
3871 this->
parameters.emplace_back(item->clone());
3875 this->
unit.reset(obj.
unit->clone());
3880 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
3893 name->set_parent(
this);
3897 item->set_parent(
this);
3902 unit->set_parent(
this);
3913 name->set_parent(
this);
3921 name->set_parent(
this);
3930 ii->set_parent(
this);
3938 ii->set_parent(
this);
3947 unit->set_parent(
this);
3955 unit->set_parent(
this);
3968 return name->get_node_name();
3991 this->
unit->accept(v);
4010 this->
unit->accept(v);
4028 : name(name), parameters(parameters), unit(unit), statement_block(statement_block) {
set_parent_in_children(); }
4032 : name(name), parameters(parameters), unit(unit), statement_block(statement_block) {
set_parent_in_children(); }
4039 this->
name.reset(obj.
name->clone());
4043 this->
parameters.emplace_back(item->clone());
4047 this->
unit.reset(obj.
unit->clone());
4056 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
4069 name->set_parent(
this);
4073 item->set_parent(
this);
4078 unit->set_parent(
this);
4093 name->set_parent(
this);
4101 name->set_parent(
this);
4110 ii->set_parent(
this);
4118 ii->set_parent(
this);
4127 unit->set_parent(
this);
4135 unit->set_parent(
this);
4165 return name->get_node_name();
4188 this->
unit->accept(v);
4207 this->
unit->accept(v);
4225 : name(name), parameters(parameters), unit(unit), statement_block(statement_block) {
set_parent_in_children(); }
4229 : name(name), parameters(parameters), unit(unit), statement_block(statement_block) {
set_parent_in_children(); }
4236 this->
name.reset(obj.
name->clone());
4240 this->
parameters.emplace_back(item->clone());
4244 this->
unit.reset(obj.
unit->clone());
4253 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
4266 name->set_parent(
this);
4270 item->set_parent(
this);
4275 unit->set_parent(
this);
4290 name->set_parent(
this);
4298 name->set_parent(
this);
4307 ii->set_parent(
this);
4315 ii->set_parent(
this);
4324 unit->set_parent(
this);
4332 unit->set_parent(
this);
4409 this->
parameters.emplace_back(item->clone());
4418 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
4431 item->set_parent(
this);
4447 ii->set_parent(
this);
4455 ii->set_parent(
this);
4558 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
4575 method->set_parent(
this);
4607 method->set_parent(
this);
4615 method->set_parent(
this);
4684 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
4768 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
4852 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
4932 BABlock::BABlock(std::shared_ptr<BABlockType> type, std::shared_ptr<StatementBlock> statement_block)
4940 this->
type.reset(obj.
type->clone());
4949 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
4962 type->set_parent(
this);
4977 type->set_parent(
this);
4985 type->set_parent(
this);
5062 this->
parameters.emplace_back(item->clone());
5071 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
5084 item->set_parent(
this);
5100 ii->set_parent(
this);
5108 ii->set_parent(
this);
5138 return name->get_node_name();
5152 for (
auto& item : this->
solvefor) {
5166 for (
auto& item : this->
solvefor) {
5196 this->
name.reset(obj.
name->clone());
5200 this->
solvefor.emplace_back(item->clone());
5209 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
5222 name->set_parent(
this);
5226 item->set_parent(
this);
5242 name->set_parent(
this);
5250 name->set_parent(
this);
5259 ii->set_parent(
this);
5267 ii->set_parent(
this);
5337 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
5350 item->set_parent(
this);
5362 ii->set_parent(
this);
5370 ii->set_parent(
this);
5418 this->
statements.emplace_back(item->clone());
5423 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
5436 item->set_parent(
this);
5448 ii->set_parent(
this);
5456 ii->set_parent(
this);
5508 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
5553 return name->eval();
5589 this->
name.reset(obj.
name->clone());
5594 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
5607 name->set_parent(
this);
5618 name->set_parent(
this);
5626 name->set_parent(
this);
5649 this->
unit->accept(v);
5660 this->
unit->accept(v);
5690 this->
unit.reset(obj.
unit->clone());
5695 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
5708 value->set_parent(
this);
5712 unit->set_parent(
this);
5723 value->set_parent(
this);
5728 this->value =
value;
5731 value->set_parent(
this);
5740 unit->set_parent(
this);
5748 unit->set_parent(
this);
5761 return name->get_node_name();
5797 this->
name.reset(obj.
name->clone());
5802 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
5815 name->set_parent(
this);
5826 name->set_parent(
this);
5834 name->set_parent(
this);
5890 this->
min.reset(obj.
min->clone());
5894 this->
max.reset(obj.
max->clone());
5899 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
5912 min->set_parent(
this);
5916 max->set_parent(
this);
5927 min->set_parent(
this);
5935 min->set_parent(
this);
5944 max->set_parent(
this);
5952 max->set_parent(
this);
6008 this->
min.reset(obj.
min->clone());
6012 this->
max.reset(obj.
max->clone());
6017 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
6030 min->set_parent(
this);
6034 max->set_parent(
this);
6045 min->set_parent(
this);
6053 min->set_parent(
this);
6062 max->set_parent(
this);
6070 max->set_parent(
this);
6083 return name->get_node_name();
6101 this->
unit->accept(v);
6115 this->
unit->accept(v);
6141 this->
name.reset(obj.
name->clone());
6149 this->
unit.reset(obj.
unit->clone());
6154 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
6167 name->set_parent(
this);
6171 value->set_parent(
this);
6175 unit->set_parent(
this);
6186 name->set_parent(
this);
6194 name->set_parent(
this);
6203 value->set_parent(
this);
6208 this->value =
value;
6211 value->set_parent(
this);
6220 unit->set_parent(
this);
6228 unit->set_parent(
this);
6269 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
6288 this->value =
value;
6328 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
6347 this->value =
value;
6387 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
6406 this->value =
value;
6457 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
6554 this->
lhs.reset(obj.
lhs->clone());
6560 this->
rhs.reset(obj.
rhs->clone());
6565 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
6578 lhs->set_parent(
this);
6583 rhs->set_parent(
this);
6594 lhs->set_parent(
this);
6602 lhs->set_parent(
this);
6620 rhs->set_parent(
this);
6628 rhs->set_parent(
this);
6680 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
6775 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
6873 this->
lhs.reset(obj.
lhs->clone());
6877 this->
rhs.reset(obj.
rhs->clone());
6882 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
6895 lhs->set_parent(
this);
6899 rhs->set_parent(
this);
6910 lhs->set_parent(
this);
6918 lhs->set_parent(
this);
6927 rhs->set_parent(
this);
6935 rhs->set_parent(
this);
6991 this->
lhs.reset(obj.
lhs->clone());
6995 this->
rhs.reset(obj.
rhs->clone());
7000 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
7013 lhs->set_parent(
this);
7017 rhs->set_parent(
this);
7028 lhs->set_parent(
this);
7036 lhs->set_parent(
this);
7045 rhs->set_parent(
this);
7053 rhs->set_parent(
this);
7066 return name->get_node_name();
7115 this->
name.reset(obj.
name->clone());
7119 this->
arguments.emplace_back(item->clone());
7124 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
7137 name->set_parent(
this);
7141 item->set_parent(
this);
7153 name->set_parent(
this);
7161 name->set_parent(
this);
7170 ii->set_parent(
this);
7178 ii->set_parent(
this);
7226 Watch::Watch(std::shared_ptr<Expression> expression, std::shared_ptr<Expression> value)
7243 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
7260 value->set_parent(
this);
7288 value->set_parent(
this);
7293 this->value =
value;
7296 value->set_parent(
this);
7337 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
7356 this->value =
value;
7368 return unit1->get_node_name();
7422 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
7435 unit1->set_parent(
this);
7439 unit2->set_parent(
this);
7450 unit1->set_parent(
this);
7455 this->unit1 =
unit1;
7458 unit1->set_parent(
this);
7467 unit2->set_parent(
this);
7472 this->unit2 =
unit2;
7475 unit2->set_parent(
this);
7488 return name->get_node_name();
7509 this->
value->accept(v);
7517 this->
gt->accept(v);
7522 this->
unit2->accept(v);
7533 this->
value->accept(v);
7541 this->
gt->accept(v);
7546 this->
unit2->accept(v);
7564 FactorDef::FactorDef(std::shared_ptr<Name> name, std::shared_ptr<Double> value, std::shared_ptr<Unit> unit1, std::shared_ptr<Boolean> gt, std::shared_ptr<Unit> unit2)
7572 this->
name.reset(obj.
name->clone());
7584 this->
gt.reset(obj.
gt->clone());
7593 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
7606 name->set_parent(
this);
7610 value->set_parent(
this);
7614 unit1->set_parent(
this);
7618 gt->set_parent(
this);
7622 unit2->set_parent(
this);
7633 name->set_parent(
this);
7641 name->set_parent(
this);
7650 value->set_parent(
this);
7655 this->value =
value;
7658 value->set_parent(
this);
7667 unit1->set_parent(
this);
7672 this->unit1 =
unit1;
7675 unit1->set_parent(
this);
7684 gt->set_parent(
this);
7692 gt->set_parent(
this);
7701 unit2->set_parent(
this);
7706 this->unit2 =
unit2;
7709 unit2->set_parent(
this);
7765 this->
type.reset(obj.
type->clone());
7774 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
7787 type->set_parent(
this);
7791 value->set_parent(
this);
7802 type->set_parent(
this);
7810 type->set_parent(
this);
7819 value->set_parent(
this);
7824 this->value =
value;
7827 value->set_parent(
this);
7868 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
7887 this->value =
value;
7913 n->set_parent(
this);
7936 auto result = first;
7938 while (first != last) {
7941 if (to_be_erased.erase(first->get()) == 0) {
7948 size_t out = last - result;
7958 n->set_parent(
this);
7977 n->set_parent(
this);
8019 this->
variables.emplace_back(item->clone());
8024 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
8037 item->set_parent(
this);
8049 ii->set_parent(
this);
8057 ii->set_parent(
this);
8109 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
8122 title->set_parent(
this);
8133 title->set_parent(
this);
8138 this->title =
title;
8141 title->set_parent(
this);
8154 return name->get_node_name();
8199 this->
name.reset(obj.
name->clone());
8208 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
8221 name->set_parent(
this);
8225 value->set_parent(
this);
8236 name->set_parent(
this);
8244 name->set_parent(
this);
8253 value->set_parent(
this);
8258 this->value =
value;
8261 value->set_parent(
this);
8283 for (
auto& item : this->
blocks) {
8294 for (
auto& item : this->
blocks) {
8324 for (
auto& item : obj.
blocks) {
8325 this->
blocks.emplace_back(item->clone());
8330 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
8346 for (
auto& item :
blocks) {
8347 item->set_parent(
this);
8375 for (
auto& ii :
blocks) {
8376 ii->set_parent(
this);
8383 for (
auto& ii :
blocks) {
8384 ii->set_parent(
this);
8397 return name->get_node_name();
8415 this->
value->accept(v);
8420 this->
unit->accept(v);
8425 this->
limit->accept(v);
8436 this->
value->accept(v);
8441 this->
unit->accept(v);
8446 this->
limit->accept(v);
8464 ParamAssign::ParamAssign(std::shared_ptr<Identifier> name, std::shared_ptr<Number> value, std::shared_ptr<Unit> unit, std::shared_ptr<Limits> limit)
8472 this->
name.reset(obj.
name->clone());
8480 this->
unit.reset(obj.
unit->clone());
8489 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
8502 name->set_parent(
this);
8506 value->set_parent(
this);
8510 unit->set_parent(
this);
8514 limit->set_parent(
this);
8525 name->set_parent(
this);
8533 name->set_parent(
this);
8542 value->set_parent(
this);
8547 this->value =
value;
8550 value->set_parent(
this);
8559 unit->set_parent(
this);
8567 unit->set_parent(
this);
8576 limit->set_parent(
this);
8581 this->limit =
limit;
8584 limit->set_parent(
this);
8597 return name->get_node_name();
8629 this->
from->accept(v);
8634 this->
to->accept(v);
8639 this->
start->accept(v);
8644 this->
unit->accept(v);
8665 this->
from->accept(v);
8670 this->
to->accept(v);
8675 this->
start->accept(v);
8680 this->
unit->accept(v);
8700 : name(name), length(length), from(from), to(to), start(start), unit(unit), abstol(abstol) {
set_parent_in_children(); }
8703 AssignedDefinition::AssignedDefinition(std::shared_ptr<Identifier> name, std::shared_ptr<Integer> length, std::shared_ptr<Number> from, std::shared_ptr<Number> to, std::shared_ptr<Number> start, std::shared_ptr<Unit> unit, std::shared_ptr<Double> abstol)
8704 : name(name), length(length), from(from), to(to), start(start), unit(unit), abstol(abstol) {
set_parent_in_children(); }
8711 this->
name.reset(obj.
name->clone());
8719 this->
from.reset(obj.
from->clone());
8723 this->
to.reset(obj.
to->clone());
8731 this->
unit.reset(obj.
unit->clone());
8740 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
8753 name->set_parent(
this);
8757 length->set_parent(
this);
8761 from->set_parent(
this);
8765 to->set_parent(
this);
8769 start->set_parent(
this);
8773 unit->set_parent(
this);
8777 abstol->set_parent(
this);
8788 name->set_parent(
this);
8796 name->set_parent(
this);
8805 length->set_parent(
this);
8813 length->set_parent(
this);
8822 from->set_parent(
this);
8830 from->set_parent(
this);
8839 to->set_parent(
this);
8847 to->set_parent(
this);
8856 start->set_parent(
this);
8861 this->start =
start;
8864 start->set_parent(
this);
8873 unit->set_parent(
this);
8881 unit->set_parent(
this);
8890 abstol->set_parent(
this);
8898 abstol->set_parent(
this);
8921 this->
ion->accept(v);
8932 this->
ion->accept(v);
8962 this->
ion.reset(obj.
ion->clone());
8967 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
8984 ion->set_parent(
this);
9012 ion->set_parent(
this);
9020 ion->set_parent(
this);
9072 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
9156 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
9201 return name->get_node_name();
9266 : name(name), from(from), to(to), increment(increment), statement_block(statement_block) {
set_parent_in_children(); }
9269 FromStatement::FromStatement(std::shared_ptr<Name> name, std::shared_ptr<Expression> from, std::shared_ptr<Expression> to, std::shared_ptr<Expression> increment, std::shared_ptr<StatementBlock> statement_block)
9270 : name(name), from(from), to(to), increment(increment), statement_block(statement_block) {
set_parent_in_children(); }
9277 this->
name.reset(obj.
name->clone());
9281 this->
from.reset(obj.
from->clone());
9285 this->
to.reset(obj.
to->clone());
9298 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
9311 name->set_parent(
this);
9315 from->set_parent(
this);
9319 to->set_parent(
this);
9338 name->set_parent(
this);
9346 name->set_parent(
this);
9355 from->set_parent(
this);
9363 from->set_parent(
this);
9372 to->set_parent(
this);
9380 to->set_parent(
this);
9479 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
9563 for (
auto& item : this->
elseifs) {
9569 this->
elses->accept(v);
9582 for (
auto& item : this->
elseifs) {
9588 this->
elses->accept(v);
9603 : condition(condition), statement_block(statement_block), elseifs(elseifs), elses(elses) {
set_parent_in_children(); }
9607 : condition(condition), statement_block(statement_block), elseifs(elseifs), elses(elses) {
set_parent_in_children(); }
9621 for (
auto& item : obj.
elseifs) {
9622 this->
elseifs.emplace_back(item->clone());
9631 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
9652 item->set_parent(
this);
9657 elses->set_parent(
this);
9702 ii->set_parent(
this);
9710 ii->set_parent(
this);
9719 elses->set_parent(
this);
9724 this->elses =
elses;
9727 elses->set_parent(
this);
9792 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
9897 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
9956 n->set_parent(
this);
9979 auto result = first;
9981 while (first != last) {
9984 if (to_be_erased.erase(first->get()) == 0) {
9991 size_t out = last - result;
10001 n->set_parent(
this);
10020 n->set_parent(
this);
10062 this->
statements.emplace_back(item->clone());
10067 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
10080 item->set_parent(
this);
10092 ii->set_parent(
this);
10100 ii->set_parent(
this);
10204 this->
expr.reset(obj.
expr->clone());
10209 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
10222 react->set_parent(
this);
10226 expr->set_parent(
this);
10237 react->set_parent(
this);
10242 this->react =
react;
10245 react->set_parent(
this);
10254 expr->set_parent(
this);
10262 expr->set_parent(
this);
10292 for (
auto& item : this->
species) {
10308 for (
auto& item : this->
species) {
10342 for (
auto& item : obj.
species) {
10343 this->
species.emplace_back(item->clone());
10348 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
10365 volume->set_parent(
this);
10369 item->set_parent(
this);
10398 volume->set_parent(
this);
10406 volume->set_parent(
this);
10415 ii->set_parent(
this);
10423 ii->set_parent(
this);
10453 for (
auto& item : this->
species) {
10469 for (
auto& item : this->
species) {
10500 this->
rate.reset(obj.
rate->clone());
10503 for (
auto& item : obj.
species) {
10504 this->
species.emplace_back(item->clone());
10509 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
10526 rate->set_parent(
this);
10530 item->set_parent(
this);
10559 rate->set_parent(
this);
10567 rate->set_parent(
this);
10576 ii->set_parent(
this);
10584 ii->set_parent(
this);
10664 : reaction1(reaction1), op(op), reaction2(reaction2), expression1(expression1), expression2(expression2) {
set_parent_in_children(); }
10668 : reaction1(reaction1), op(op), reaction2(reaction2), expression1(expression1), expression2(expression2) {
set_parent_in_children(); }
10694 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
10855 this->
name.reset(obj.
name->clone());
10864 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
10877 name->set_parent(
this);
10881 byname->set_parent(
this);
10892 name->set_parent(
this);
10900 name->set_parent(
this);
10909 byname->set_parent(
this);
10917 byname->set_parent(
this);
10969 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
11081 : table_vars(table_vars), depend_vars(depend_vars), from(from), to(to), with(with) {
set_parent_in_children(); }
11085 : table_vars(table_vars), depend_vars(depend_vars), from(from), to(to), with(with) {
set_parent_in_children(); }
11092 this->
table_vars.emplace_back(item->clone());
11100 this->
from.reset(obj.
from->clone());
11104 this->
to.reset(obj.
to->clone());
11108 this->
with.reset(obj.
with->clone());
11113 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
11126 item->set_parent(
this);
11131 item->set_parent(
this);
11136 from->set_parent(
this);
11140 to->set_parent(
this);
11144 with->set_parent(
this);
11155 ii->set_parent(
this);
11163 ii->set_parent(
this);
11172 ii->set_parent(
this);
11180 ii->set_parent(
this);
11189 from->set_parent(
this);
11197 from->set_parent(
this);
11206 to->set_parent(
this);
11214 to->set_parent(
this);
11223 with->set_parent(
this);
11231 with->set_parent(
this);
11247 return name->get_node_name();
11289 this->
type.reset(obj.
type->clone());
11293 this->
name.reset(obj.
name->clone());
11298 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
11311 type->set_parent(
this);
11315 name->set_parent(
this);
11326 type->set_parent(
this);
11334 type->set_parent(
this);
11343 name->set_parent(
this);
11351 name->set_parent(
this);
11364 return name->get_node_name();
11384 for (
auto& item : this->
readlist) {
11410 for (
auto& item : this->
readlist) {
11441 : name(name), readlist(readlist), writelist(writelist), valence(valence), ontology_id(ontology_id) {
set_parent_in_children(); }
11445 : name(name), readlist(readlist), writelist(writelist), valence(valence), ontology_id(ontology_id) {
set_parent_in_children(); }
11452 this->
name.reset(obj.
name->clone());
11456 this->
readlist.emplace_back(item->clone());
11460 this->
writelist.emplace_back(item->clone());
11473 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
11486 name->set_parent(
this);
11490 item->set_parent(
this);
11495 item->set_parent(
this);
11515 name->set_parent(
this);
11523 name->set_parent(
this);
11532 ii->set_parent(
this);
11540 ii->set_parent(
this);
11549 ii->set_parent(
this);
11557 ii->set_parent(
this);
11607 for (
auto& item : this->
currents) {
11615 for (
auto& item : this->
currents) {
11639 this->
currents.emplace_back(item->clone());
11644 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
11657 item->set_parent(
this);
11669 ii->set_parent(
this);
11677 ii->set_parent(
this);
11693 for (
auto& item : this->
currents) {
11701 for (
auto& item : this->
currents) {
11725 this->
currents.emplace_back(item->clone());
11730 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
11743 item->set_parent(
this);
11755 ii->set_parent(
this);
11763 ii->set_parent(
this);
11811 this->
variables.emplace_back(item->clone());
11816 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
11829 item->set_parent(
this);
11841 ii->set_parent(
this);
11849 ii->set_parent(
this);
11876 n->set_parent(
this);
11899 auto result = first;
11901 while (first != last) {
11904 if (to_be_erased.erase(first->get()) == 0) {
11911 size_t out = last - result;
11921 n->set_parent(
this);
11940 n->set_parent(
this);
11982 this->
variables.emplace_back(item->clone());
11987 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
12000 item->set_parent(
this);
12012 ii->set_parent(
this);
12020 ii->set_parent(
this);
12068 this->
variables.emplace_back(item->clone());
12073 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
12086 item->set_parent(
this);
12098 ii->set_parent(
this);
12106 ii->set_parent(
this);
12133 n->set_parent(
this);
12156 auto result = first;
12158 while (first != last) {
12161 if (to_be_erased.erase(first->get()) == 0) {
12168 size_t out = last - result;
12178 n->set_parent(
this);
12197 n->set_parent(
this);
12239 this->
variables.emplace_back(item->clone());
12244 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
12257 item->set_parent(
this);
12269 ii->set_parent(
this);
12277 ii->set_parent(
this);
12325 this->
variables.emplace_back(item->clone());
12330 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
12343 item->set_parent(
this);
12355 ii->set_parent(
this);
12363 ii->set_parent(
this);
12411 this->
variables.emplace_back(item->clone());
12416 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
12429 item->set_parent(
this);
12441 ii->set_parent(
this);
12449 ii->set_parent(
this);
12523 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
12607 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
12691 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
12775 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
12834 n->set_parent(
this);
12841 return blocks.erase(first);
12846 NodeVector::const_iterator
Program::erase_node(NodeVector::const_iterator first, NodeVector::const_iterator last) {
12847 return blocks.erase(first, last);
12855 auto first =
blocks.begin();
12856 auto last =
blocks.end();
12857 auto result = first;
12859 while (first != last) {
12862 if (to_be_erased.erase(first->get()) == 0) {
12869 size_t out = last - result;
12879 n->set_parent(
this);
12880 return blocks.insert(position, n);
12898 n->set_parent(
this);
12908 for (
auto& item : this->
blocks) {
12916 for (
auto& item : this->
blocks) {
12939 for (
auto& item : obj.
blocks) {
12940 this->
blocks.emplace_back(item->clone());
12945 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
12957 for (
auto& item :
blocks) {
12958 item->set_parent(
this);
12969 for (
auto& ii :
blocks) {
12970 ii->set_parent(
this);
12977 for (
auto& ii :
blocks) {
12978 ii->set_parent(
this);
13031 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
13044 item->set_parent(
this);
13056 ii->set_parent(
this);
13064 ii->set_parent(
this);
13154 : n_state_vars(n_state_vars), variable_block(variable_block), initialize_block(initialize_block), setup_x_block(setup_x_block), functor_block(functor_block), update_states_block(update_states_block), finalize_block(finalize_block) {
set_parent_in_children(); }
13157 EigenNewtonSolverBlock::EigenNewtonSolverBlock(std::shared_ptr<Integer> n_state_vars, std::shared_ptr<StatementBlock> variable_block, std::shared_ptr<StatementBlock> initialize_block, std::shared_ptr<StatementBlock> setup_x_block, std::shared_ptr<StatementBlock> functor_block, std::shared_ptr<StatementBlock> update_states_block, std::shared_ptr<StatementBlock> finalize_block)
13158 : n_state_vars(n_state_vars), variable_block(variable_block), initialize_block(initialize_block), setup_x_block(setup_x_block), functor_block(functor_block), update_states_block(update_states_block), finalize_block(finalize_block) {
set_parent_in_children(); }
13194 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
13433 : n_state_vars(n_state_vars), variable_block(variable_block), initialize_block(initialize_block), setup_x_block(setup_x_block), update_states_block(update_states_block), finalize_block(finalize_block) {
set_parent_in_children(); }
13436 EigenLinearSolverBlock::EigenLinearSolverBlock(std::shared_ptr<Integer> n_state_vars, std::shared_ptr<StatementBlock> variable_block, std::shared_ptr<StatementBlock> initialize_block, std::shared_ptr<StatementBlock> setup_x_block, std::shared_ptr<StatementBlock> update_states_block, std::shared_ptr<StatementBlock> finalize_block)
13437 : n_state_vars(n_state_vars), variable_block(variable_block), initialize_block(initialize_block), setup_x_block(setup_x_block), update_states_block(update_states_block), finalize_block(finalize_block) {
set_parent_in_children(); }
13469 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
13619 return name->get_node_name();
13671 : name(name), n_odes(n_odes), non_stiff_block(non_stiff_block), stiff_block(stiff_block) {
set_parent_in_children(); }
13674 CvodeBlock::CvodeBlock(std::shared_ptr<Name> name, std::shared_ptr<Integer> n_odes, std::shared_ptr<StatementBlock> non_stiff_block, std::shared_ptr<StatementBlock> stiff_block)
13675 : name(name), n_odes(n_odes), non_stiff_block(non_stiff_block), stiff_block(stiff_block) {
set_parent_in_children(); }
13682 this->
name.reset(obj.
name->clone());
13699 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
13712 name->set_parent(
this);
13716 n_odes->set_parent(
this);
13735 name->set_parent(
this);
13743 name->set_parent(
this);
13752 n_odes->set_parent(
this);
13760 n_odes->set_parent(
this);
13807 return name->get_node_name();
13850 : name(name), longitudinal_diffusion_statements(longitudinal_diffusion_statements), compartment_statements(compartment_statements) {
set_parent_in_children(); }
13854 : name(name), longitudinal_diffusion_statements(longitudinal_diffusion_statements), compartment_statements(compartment_statements) {
set_parent_in_children(); }
13861 this->
name.reset(obj.
name->clone());
13874 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
13887 name->set_parent(
this);
13906 name->set_parent(
this);
13914 name->set_parent(
this);
14000 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
14084 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
14181 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
14286 this->
token = std::shared_ptr<ModToken>(obj.
token->clone());
14299 value->set_parent(
this);
14310 value->set_parent(
this);
14315 this->value =
value;
14318 value->set_parent(
this);
void set_parent_in_children()
Set this object as parent for all the children.
void set_variables(LocalVarVector &&variables)
Setter for member variable LocalListStatement::variables (rvalue reference)
std::string get_node_name() const override
Return name of the node.
BinaryOp
enum Type for binary operators in NMODL
std::vector< std::shared_ptr< ConstantStatement > > ConstantStatementVector
virtual void visit_react_var_name(const ast::ReactVarName &node)=0
visit node of type ast::ReactVarName
std::shared_ptr< String > ontology_id
Ontology to indicate the chemical ion.
ConstantStatement(ConstantVar *constant)
std::shared_ptr< ModToken > token
token with location information
std::shared_ptr< StatementBlock > statement_block
TODO.
void visit_children(visitor::Visitor &v) override
visit children i.e.
void visit_children(visitor::Visitor &v) override
visit children i.e.
void visit_children(visitor::Visitor &v) override
visit children i.e.
BinaryOperator op
Operator.
virtual bool is_argument() const noexcept
Check if the ast node is an instance of ast::Argument.
Abstract base class for all constant visitors implementation.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
void set_value(std::shared_ptr< Integer > &&value)
Setter for member variable Define::value (rvalue reference)
StatementVector statements
Vector of statements.
void set_to(std::shared_ptr< Expression > &&to)
Setter for member variable FromStatement::to (rvalue reference)
std::shared_ptr< ModToken > token
token with location information
std::shared_ptr< ModToken > token
token with location information
void set_table_vars(NameVector &&table_vars)
Setter for member variable TableStatement::table_vars (rvalue reference)
void visit_children(visitor::Visitor &v) override
visit children i.e.
Represents a BEFORE block in NMODL.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
void set_parent_in_children()
Set this object as parent for all the children.
std::shared_ptr< ModToken > token
token with location information
Represents a variable in the ast::ConstantBlock.
void set_value(std::string value)
Setter for member variable Float::value.
void set_solve_block(std::shared_ptr< SolveBlock > &&solve_block)
Setter for member variable SolutionExpression::solve_block (rvalue reference)
virtual void visit_after_block(ast::AfterBlock &node)=0
visit node of type ast::AfterBlock
std::shared_ptr< Expression > rhs
RHS of the binary expression.
LagStatement(Identifier *name, Name *byname)
void set_statement_block(std::shared_ptr< StatementBlock > &&statement_block)
Setter for member variable BABlock::statement_block (rvalue reference)
virtual void visit_eigen_linear_solver_block(const ast::EigenLinearSolverBlock &node)=0
visit node of type ast::EigenLinearSolverBlock
std::shared_ptr< Identifier > name
TODO.
void set_parameters(ArgumentVector &¶meters)
Setter for member variable NetReceiveBlock::parameters (rvalue reference)
void visit_children(visitor::Visitor &v) override
visit children i.e.
LonDiffuse(Name *index_name, Expression *rate, const NameVector &species)
virtual bool is_external() const noexcept
Check if the ast node is an instance of ast::External.
std::shared_ptr< ModToken > token
token with location information
void set_start(std::shared_ptr< Number > &&start)
Setter for member variable AssignedDefinition::start (rvalue reference)
Represents an INCLUDE statement in NMODL.
Useion(Name *name, const ReadIonVarVector &readlist, const WriteIonVarVector &writelist, Valence *valence, String *ontology_id)
virtual void visit_function_block(ast::FunctionBlock &node)=0
visit node of type ast::FunctionBlock
RangeVarVector variables
Vector of range variables.
void set_finalize_block(std::shared_ptr< StatementBlock > &&finalize_block)
Setter for member variable EigenLinearSolverBlock::finalize_block (rvalue reference)
virtual bool is_double_unit() const noexcept
Check if the ast node is an instance of ast::DoubleUnit.
std::shared_ptr< ModToken > token
token with location information
void set_unit(std::shared_ptr< Unit > &&unit)
Setter for member variable ConstantVar::unit (rvalue reference)
void set_to(std::shared_ptr< Number > &&to)
Setter for member variable AssignedDefinition::to (rvalue reference)
virtual bool is_kinetic_block() const noexcept
Check if the ast node is an instance of ast::KineticBlock.
NrnStateBlock(const StatementVector &solve_statements)
void visit_children(visitor::Visitor &v) override
visit children i.e.
std::shared_ptr< Number > min
TODO.
virtual void visit_expression(const ast::Expression &node)=0
visit node of type ast::Expression
void set_value(std::shared_ptr< Expression > &&value)
Setter for member variable Watch::value (rvalue reference)
void set_statements(WatchVector &&statements)
Setter for member variable WatchStatement::statements (rvalue reference)
virtual void visit_binary_operator(ast::BinaryOperator &node)=0
visit node of type ast::BinaryOperator
virtual void visit_expression_statement(const ast::ExpressionStatement &node)=0
visit node of type ast::ExpressionStatement
virtual bool is_local_list_statement() const noexcept
Check if the ast node is an instance of ast::LocalListStatement.
UnaryOp
enum type for unary operators
std::shared_ptr< Name > index_name
Index variable name.
void visit_children(visitor::Visitor &v) override
visit children i.e.
void visit_children(visitor::Visitor &v) override
visit children i.e.
ParamBlock(const ParamAssignVector &statements)
std::shared_ptr< ModToken > token
token with location information
Base class for all AST node.
void set_statement_block(std::shared_ptr< StatementBlock > &&statement_block)
Setter for member variable ProcedureBlock::statement_block (rvalue reference)
void set_parent_in_children()
Set this object as parent for all the children.
std::shared_ptr< Expression > expression1
TODO.
Represents a block to be executed before or after another block.
virtual void visit_table_statement(ast::TableStatement &node)=0
visit node of type ast::TableStatement
Represents a C code block.
void visit_children(visitor::Visitor &v) override
visit children i.e.
void set_parameters(ArgumentVector &¶meters)
Setter for member variable ForNetcon::parameters (rvalue reference)
std::shared_ptr< ModToken > token
token with location information
void set_name(std::shared_ptr< Name > &&name)
Setter for member variable LongitudinalDiffusionBlock::name (rvalue reference)
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
virtual void visit_eigen_linear_solver_block(ast::EigenLinearSolverBlock &node)=0
visit node of type ast::EigenLinearSolverBlock
virtual void visit_assigned_definition(const ast::AssignedDefinition &node)=0
visit node of type ast::AssignedDefinition
void set_statement_block(std::shared_ptr< StatementBlock > &&statement_block)
Setter for member variable ConstructorBlock::statement_block (rvalue reference)
void set_op(ReactionOperator &&op)
Setter for member variable ReactionStatement::op (rvalue reference)
std::shared_ptr< ModToken > token
token with location information
virtual bool is_statement() const noexcept
Check if the ast node is an instance of ast::Statement.
void set_variables(NameVector &&variables)
Setter for member variable IndependentBlock::variables (rvalue reference)
void set_expression(std::shared_ptr< Expression > &&expression)
Setter for member variable Watch::expression (rvalue reference)
std::shared_ptr< Number > max
TODO.
std::shared_ptr< ModToken > token
token with location information
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
virtual bool is_block() const noexcept
Check if the ast node is an instance of ast::Block.
void set_statement_block(std::shared_ptr< StatementBlock > &&statement_block)
Setter for member variable FunctionBlock::statement_block (rvalue reference)
virtual void visit_else_if_statement(const ast::ElseIfStatement &node)=0
visit node of type ast::ElseIfStatement
void visit_children(visitor::Visitor &v) override
visit children i.e.
virtual void visit_after_block(const ast::AfterBlock &node)=0
visit node of type ast::AfterBlock
void set_parent_in_children()
Set this object as parent for all the children.
std::shared_ptr< Name > type
type of channel
virtual bool is_unit_block() const noexcept
Check if the ast node is an instance of ast::UnitBlock.
void visit_children(visitor::Visitor &v) override
visit children i.e.
void set_unit(std::shared_ptr< Unit > &&unit)
Setter for member variable FunctionTableBlock::unit (rvalue reference)
void visit_children(visitor::Visitor &v) override
visit children i.e.
void visit_children(visitor::Visitor &v) override
visit children i.e.
virtual void visit_argument(ast::Argument &node)=0
visit node of type ast::Argument
std::shared_ptr< Integer > n_odes
number of ODEs to solve
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
std::shared_ptr< StatementBlock > statement_block
TODO.
void set_statement_block(std::shared_ptr< StatementBlock > &&statement_block)
Setter for member variable BreakpointBlock::statement_block (rvalue reference)
std::shared_ptr< Valence > valence
(TODO)
virtual void visit_mutex_unlock(ast::MutexUnlock &node)=0
visit node of type ast::MutexUnlock
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
Base class for all identifiers.
virtual bool is_range() const noexcept
Check if the ast node is an instance of ast::Range.
virtual bool is_net_receive_block() const noexcept
Check if the ast node is an instance of ast::NetReceiveBlock.
void set_variables(ExternVarVector &&variables)
Setter for member variable External::variables (rvalue reference)
LinearBlock(Name *name, const NameVector &solvefor, StatementBlock *statement_block)
virtual void visit_node(ast::Node &node)=0
visit node of type ast::Node
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
virtual void visit_watch_statement(const ast::WatchStatement &node)=0
visit node of type ast::WatchStatement
void set_rhs(std::shared_ptr< Expression > &&rhs)
Setter for member variable LinEquation::rhs (rvalue reference)
std::shared_ptr< ModToken > token
token with location information
void set_name(std::shared_ptr< Identifier > &&name)
Setter for member variable ParamAssign::name (rvalue reference)
std::shared_ptr< ModToken > token
token with location information
void set_name(std::shared_ptr< Identifier > &&name)
Setter for member variable Argument::name (rvalue reference)
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
IndependentBlock(const NameVector &variables)
std::string get_node_name() const override
Return name of the node.
virtual void visit_range(const ast::Range &node)=0
visit node of type ast::Range
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
virtual void visit_useion(ast::Useion &node)=0
visit node of type ast::Useion
virtual void visit_eigen_newton_solver_block(const ast::EigenNewtonSolverBlock &node)=0
visit node of type ast::EigenNewtonSolverBlock
std::shared_ptr< Name > conductance
Conductance variable.
void set_update_states_block(std::shared_ptr< StatementBlock > &&update_states_block)
Setter for member variable EigenLinearSolverBlock::update_states_block (rvalue reference)
std::shared_ptr< Expression > expression2
TODO.
Represents differential equation in DERIVATIVE block.
int value
Value of integer.
std::shared_ptr< Expression > lhs
Left-hand-side of the equation.
void set_unit(std::shared_ptr< Unit > &&unit)
Setter for member variable DoubleUnit::unit (rvalue reference)
LocalVarVector::const_iterator erase_local_var(LocalVarVector::const_iterator first)
Erase member to variables.
Base class for all Abstract Syntax Tree node types.
virtual void visit_constant_block(ast::ConstantBlock &node)=0
visit node of type ast::ConstantBlock
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
std::shared_ptr< Boolean > gt
Todo: Michael : rename variable gt as well.
virtual void visit_pointer(ast::Pointer &node)=0
visit node of type ast::Pointer
std::string get_node_name() const override
Return name of the node.
Represents a statement in ASSIGNED or STATE block.
ReadIonVarVector readlist
Variables being read.
void set_statement_block(std::shared_ptr< StatementBlock > &&statement_block)
Setter for member variable DiscreteBlock::statement_block (rvalue reference)
std::shared_ptr< Identifier > name
TODO.
virtual void visit_watch_statement(ast::WatchStatement &node)=0
visit node of type ast::WatchStatement
virtual void visit_boolean(ast::Boolean &node)=0
visit node of type ast::Boolean
void set_finalize_block(std::shared_ptr< StatementBlock > &&finalize_block)
Setter for member variable EigenNewtonSolverBlock::finalize_block (rvalue reference)
virtual void visit_electrode_current(ast::ElectrodeCurrent &node)=0
visit node of type ast::ElectrodeCurrent
BAType
enum type to distinguish BEFORE or AFTER blocks
std::shared_ptr< ModToken > token
token with location information
std::shared_ptr< Name > name
TODO.
virtual void visit_non_linear_block(ast::NonLinearBlock &node)=0
visit node of type ast::NonLinearBlock
virtual void visit_define(ast::Define &node)=0
visit node of type ast::Define
void set_parent_in_children()
Set this object as parent for all the children.
void visit_children(visitor::Visitor &v) override
visit children i.e.
ForNetcon(const ArgumentVector ¶meters, StatementBlock *statement_block)
std::shared_ptr< Name > name
TODO.
std::shared_ptr< Expression > to
TODO.
virtual void visit_paren_expression(const ast::ParenExpression &node)=0
visit node of type ast::ParenExpression
std::shared_ptr< ModToken > token
token with location information
std::shared_ptr< Expression > reaction2
TODO.
std::shared_ptr< ModToken > token
token with location information
virtual bool is_paren_expression() const noexcept
Check if the ast node is an instance of ast::ParenExpression.
std::shared_ptr< ModToken > token
token with location information
virtual bool is_string() const noexcept
Check if the ast node is an instance of ast::String.
std::shared_ptr< ModToken > token
token with location information
std::shared_ptr< ModToken > token
token with location information
std::shared_ptr< Expression > expression
TODO.
virtual bool is_non_linear_block() const noexcept
Check if the ast node is an instance of ast::NonLinearBlock.
virtual void visit_assigned_block(ast::AssignedBlock &node)=0
visit node of type ast::AssignedBlock
void set_currents(ElectrodeCurVarVector &¤ts)
Setter for member variable ElectrodeCurrent::currents (rvalue reference)
std::shared_ptr< ModToken > token
token with location information
void set_ontology_id(std::shared_ptr< String > &&ontology_id)
Setter for member variable OntologyStatement::ontology_id (rvalue reference)
Represents a double variable.
virtual bool is_eigen_newton_solver_block() const noexcept
Check if the ast node is an instance of ast::EigenNewtonSolverBlock.
Represents RANGE variables statement in NMODL.
virtual void visit_statement(ast::Statement &node)=0
visit node of type ast::Statement
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
std::shared_ptr< StatementBlock > longitudinal_diffusion_statements
All LONGITUDINAL_DIFFUSION statements in the KINETIC block.
virtual bool is_local_var() const noexcept
Check if the ast node is an instance of ast::LocalVar.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
void set_parent_in_children()
Set this object as parent for all the children.
virtual void visit_verbatim(ast::Verbatim &node)=0
visit node of type ast::Verbatim
virtual void visit_non_lin_equation(ast::NonLinEquation &node)=0
visit node of type ast::NonLinEquation
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
NameVector table_vars
Variables in the table.
std::shared_ptr< Integer > order
order of ODE
One equation in a system of equations that collectively make a NONLINEAR block.
Represents RANDOM statement in NMODL.
Pointer(const PointerVarVector &variables)
void set_update_states_block(std::shared_ptr< StatementBlock > &&update_states_block)
Setter for member variable EigenNewtonSolverBlock::update_states_block (rvalue reference)
std::shared_ptr< ModToken > token
token with location information
void set_node_to_solve(std::shared_ptr< Block > &&node_to_solve)
Setter for member variable DerivimplicitCallback::node_to_solve (rvalue reference)
void set_n_state_vars(std::shared_ptr< Integer > &&n_state_vars)
Setter for member variable EigenLinearSolverBlock::n_state_vars (rvalue reference)
void set_statement_block(std::shared_ptr< StatementBlock > &&statement_block)
Setter for member variable FromStatement::statement_block (rvalue reference)
void set_expression(std::shared_ptr< Expression > &&expression)
Setter for member variable ProtectStatement::expression (rvalue reference)
AssignedBlock(const AssignedDefinitionVector &definitions)
ExternVarVector variables
Vector of external variables.
Represent a single variable of type BBCOREPOINTER.
void visit_children(visitor::Visitor &v) override
visit children i.e.
LongitudinalDiffusionBlock(Name *name, StatementBlock *longitudinal_diffusion_statements, StatementBlock *compartment_statements)
std::shared_ptr< Integer > with
an increment factor
std::string get_node_name() const override
Return name of the node.
virtual void visit_statement(const ast::Statement &node)=0
visit node of type ast::Statement
ParenExpression(Expression *expression)
std::shared_ptr< Integer > value
TODO.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
std::vector< std::shared_ptr< PointerVar > > PointerVarVector
void set_parent_in_children()
Set this object as parent for all the children.
virtual void visit_conductance_hint(const ast::ConductanceHint &node)=0
visit node of type ast::ConductanceHint
std::shared_ptr< ModToken > token
token with location information
Verbatim(String *statement)
void set_expression2(std::shared_ptr< Expression > &&expression2)
Setter for member variable ReactionStatement::expression2 (rvalue reference)
std::shared_ptr< ModToken > token
token with location information
virtual void visit_expression_statement(ast::ExpressionStatement &node)=0
visit node of type ast::ExpressionStatement
std::shared_ptr< ModToken > token
token with location information
void set_value(ReactionOp value)
Setter for member variable ReactionOperator::value.
virtual void visit_local_var(ast::LocalVar &node)=0
visit node of type ast::LocalVar
void set_statement_block(std::shared_ptr< StatementBlock > &&statement_block)
Setter for member variable ForNetcon::statement_block (rvalue reference)
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
void set_definitions(AssignedDefinitionVector &&definitions)
Setter for member variable StateBlock::definitions (rvalue reference)
void set_to(std::shared_ptr< Expression > &&to)
Setter for member variable TableStatement::to (rvalue reference)
void set_parent_in_children()
Set this object as parent for all the children.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
void set_parent_in_children()
Set this object as parent for all the children.
virtual void visit_reaction_statement(const ast::ReactionStatement &node)=0
visit node of type ast::ReactionStatement
virtual bool is_ontology_statement() const noexcept
Check if the ast node is an instance of ast::OntologyStatement.
ConstructorBlock(StatementBlock *statement_block)
KineticBlock(Name *name, const NameVector &solvefor, StatementBlock *statement_block)
void set_value(std::shared_ptr< Double > &&value)
Setter for member variable UpdateDt::value (rvalue reference)
std::shared_ptr< ModToken > token
token with location information
std::shared_ptr< ModToken > token
token with location information
virtual void visit_suffix(const ast::Suffix &node)=0
visit node of type ast::Suffix
void set_parent_in_children()
Set this object as parent for all the children.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
ArgumentVector parameters
Parameters to the net receive block.
virtual bool is_global() const noexcept
Check if the ast node is an instance of ast::Global.
std::shared_ptr< Name > name
Name of the discrete block.
std::string get_node_name() const override
Return name of the node.
void visit_children(visitor::Visitor &v) override
visit children i.e.
Represent CONSERVE statement in NMODL.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
void set_parent_in_children()
Set this object as parent for all the children.
virtual bool is_conserve() const noexcept
Check if the ast node is an instance of ast::Conserve.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
StateBlock(const AssignedDefinitionVector &definitions)
virtual bool is_destructor_block() const noexcept
Check if the ast node is an instance of ast::DestructorBlock.
virtual bool is_conductance_hint() const noexcept
Check if the ast node is an instance of ast::ConductanceHint.
void set_elses(std::shared_ptr< ElseStatement > &&elses)
Setter for member variable IfStatement::elses (rvalue reference)
void visit_children(visitor::Visitor &v) override
visit children i.e.
AssignedDefinitionVector::const_iterator insert_assigned_definition(AssignedDefinitionVector::const_iterator position, const std::shared_ptr< AssignedDefinition > &n)
Insert member to definitions.
VarName(Identifier *name, Integer *at, Expression *index)
void visit_children(visitor::Visitor &v) override
visit children i.e.
std::shared_ptr< StatementBlock > statement_block
Block with statements vector.
void set_parent_in_children()
Set this object as parent for all the children.
void set_statement_block(std::shared_ptr< StatementBlock > &&statement_block)
Setter for member variable ElseIfStatement::statement_block (rvalue reference)
std::shared_ptr< StatementBlock > update_states_block
update back states from X
std::shared_ptr< String > title
TODO.
std::vector< std::shared_ptr< Statement > > StatementVector
std::shared_ptr< StatementBlock > statement_block
Block with statements vector.
virtual void visit_destructor_block(const ast::DestructorBlock &node)=0
visit node of type ast::DestructorBlock
virtual bool is_function_call() const noexcept
Check if the ast node is an instance of ast::FunctionCall.
std::shared_ptr< ModToken > token
token with location information
ConductanceHint(Name *conductance, Name *ion)
virtual void visit_mutex_lock(ast::MutexLock &node)=0
visit node of type ast::MutexLock
virtual void visit_breakpoint_block(ast::BreakpointBlock &node)=0
visit node of type ast::BreakpointBlock
CvodeBlock(Name *name, Integer *n_odes, StatementBlock *non_stiff_block, StatementBlock *stiff_block)
std::string get_node_name() const override
Return name of the node.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
virtual void visit_global(const ast::Global &node)=0
visit node of type ast::Global
AssignedDefinitionVector::const_iterator erase_assigned_definition(AssignedDefinitionVector::const_iterator first)
Erase member to definitions.
virtual void visit_number_range(const ast::NumberRange &node)=0
visit node of type ast::NumberRange
Represents CONDUCTANCE statement in NMODL.
std::shared_ptr< ModToken > token
token with location information
virtual void visit_else_statement(ast::ElseStatement &node)=0
visit node of type ast::ElseStatement
virtual void visit_ba_block(ast::BABlock &node)=0
visit node of type ast::BABlock
virtual bool is_suffix() const noexcept
Check if the ast node is an instance of ast::Suffix.
DoubleUnit(Double *value, Unit *unit)
std::shared_ptr< BABlock > bablock
Block to be called before.
std::vector< std::shared_ptr< ParamAssign > > ParamAssignVector
std::vector< std::shared_ptr< RangeVar > > RangeVarVector
void set_parent_in_children()
Set this object as parent for all the children.
void set_parent_in_children()
Set this object as parent for all the children.
void set_statement_block(std::shared_ptr< StatementBlock > &&statement_block)
Setter for member variable IfStatement::statement_block (rvalue reference)
PointerVarVector::const_iterator erase_pointer_var(PointerVarVector::const_iterator first)
Erase member to variables.
void set_initialize_block(std::shared_ptr< StatementBlock > &&initialize_block)
Setter for member variable EigenNewtonSolverBlock::initialize_block (rvalue reference)
void set_method(std::shared_ptr< Name > &&method)
Setter for member variable SolveBlock::method (rvalue reference)
PointerVarVector variables
Vector of pointer variables.
void visit_children(visitor::Visitor &v) override
visit children i.e.
void visit_children(visitor::Visitor &v) override
visit children i.e.
void set_parent_in_children()
Set this object as parent for all the children.
virtual void visit_ontology_statement(ast::OntologyStatement &node)=0
visit node of type ast::OntologyStatement
virtual void visit_conductance_hint(ast::ConductanceHint &node)=0
visit node of type ast::ConductanceHint
void set_parent_in_children()
Set this object as parent for all the children.
virtual void visit_function_call(const ast::FunctionCall &node)=0
visit node of type ast::FunctionCall
std::shared_ptr< StatementBlock > statement_block
Block with statements vector.
virtual void visit_lag_statement(ast::LagStatement &node)=0
visit node of type ast::LagStatement
WatchStatement(const WatchVector &statements)
void set_parent_in_children()
Set this object as parent for all the children.
virtual void visit_linear_block(const ast::LinearBlock &node)=0
visit node of type ast::LinearBlock
virtual void visit_block(ast::Block &node)=0
visit node of type ast::Block
std::shared_ptr< Unit > unit
Unit if specified.
std::shared_ptr< ModToken > token
token with location information
void visit_children(visitor::Visitor &v) override
visit children i.e.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
std::shared_ptr< Expression > node_to_solve
Block to be solved (callback node or solution node itself)
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
void set_statements(StatementVector &&statements)
Setter for member variable StatementBlock::statements (rvalue reference)
virtual void visit_string(ast::String &node)=0
visit node of type ast::String
virtual void visit_unit_block(const ast::UnitBlock &node)=0
visit node of type ast::UnitBlock
void set_unit(std::shared_ptr< Unit > &&unit)
Setter for member variable AssignedDefinition::unit (rvalue reference)
ReactVarName(Integer *value, VarName *name)
std::vector< std::shared_ptr< Node > > NodeVector
std::shared_ptr< ModToken > token
token with location information
ProcedureBlock(Name *name, const ArgumentVector ¶meters, Unit *unit, StatementBlock *statement_block)
External(const ExternVarVector &variables)
void set_name(std::shared_ptr< Name > &&name)
Setter for member variable CvodeBlock::name (rvalue reference)
std::shared_ptr< ElseStatement > elses
TODO.
void set_parent_in_children()
Set this object as parent for all the children.
void set_parameters(ArgumentVector &¶meters)
Setter for member variable ProcedureBlock::parameters (rvalue reference)
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
virtual void visit_for_netcon(const ast::ForNetcon &node)=0
visit node of type ast::ForNetcon
std::shared_ptr< StatementBlock > statement_block
TODO.
ReactionOp
enum type used for Reaction statement
void visit_children(visitor::Visitor &v) override
visit children i.e.
void set_name(std::shared_ptr< Identifier > &&name)
Setter for member variable IndexedName::name (rvalue reference)
virtual void visit_diff_eq_expression(ast::DiffEqExpression &node)=0
visit node of type ast::DiffEqExpression
GlobalVarVector::const_iterator erase_global_var(GlobalVarVector::const_iterator first)
Erase member to variables.
void visit_children(visitor::Visitor &v) override
visit children i.e.
void set_name(std::shared_ptr< Name > &&name)
Setter for member variable FunctionTableBlock::name (rvalue reference)
std::shared_ptr< Integer > value
Value of the macro.
SolutionExpression(SolveBlock *solve_block, Expression *node_to_solve)
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
virtual void visit_mutex_unlock(const ast::MutexUnlock &node)=0
visit node of type ast::MutexUnlock
Represents a DEFINE statement in NMODL.
void set_name(std::shared_ptr< Name > &&name)
Setter for member variable ExternVar::name (rvalue reference)
std::shared_ptr< StatementBlock > statement_block
Block with statements vector.
std::string get_node_name() const override
Return name of the node.
virtual void visit_cvode_block(ast::CvodeBlock &node)=0
visit node of type ast::CvodeBlock
void set_parent_in_children()
Set this object as parent for all the children.
Watch(Expression *expression, Expression *value)
NodeVector blocks
AST of the included file.
void set_parent_in_children()
Set this object as parent for all the children.
std::shared_ptr< Expression > rhs
Right-hand-side of the equation.
Represents a block used for variable timestep integration (CVODE) of DERIVATIVE blocks.
std::shared_ptr< Name > name
TODO.
std::shared_ptr< ModToken > token
token with location information
Implement classes for representing symbol table at block and file scope.
virtual void visit_discrete_block(ast::DiscreteBlock &node)=0
visit node of type ast::DiscreteBlock
std::shared_ptr< StatementBlock > statement_block
Block with statements vector.
void set_unit2(std::shared_ptr< Unit > &&unit2)
Setter for member variable FactorDef::unit2 (rvalue reference)
virtual bool is_assigned_block() const noexcept
Check if the ast node is an instance of ast::AssignedBlock.
virtual void visit_random_var(ast::RandomVar &node)=0
visit node of type ast::RandomVar
virtual void visit_react_var_name(ast::ReactVarName &node)=0
visit node of type ast::ReactVarName
virtual void visit_non_linear_block(const ast::NonLinearBlock &node)=0
visit node of type ast::NonLinearBlock
void set_statement_block(std::shared_ptr< StatementBlock > &&statement_block)
Setter for member variable KineticBlock::statement_block (rvalue reference)
void set_abstol(std::shared_ptr< Double > &&abstol)
Setter for member variable AssignedDefinition::abstol (rvalue reference)
virtual void visit_derivative_block(ast::DerivativeBlock &node)=0
visit node of type ast::DerivativeBlock
virtual void visit_conserve(const ast::Conserve &node)=0
visit node of type ast::Conserve
std::shared_ptr< Unit > unit
Unit if specified.
void set_unit2(std::shared_ptr< Unit > &&unit2)
Setter for member variable UnitDef::unit2 (rvalue reference)
virtual void visit_valence(ast::Valence &node)=0
visit node of type ast::Valence
virtual void visit_unit(const ast::Unit &node)=0
visit node of type ast::Unit
NodeVector::const_iterator erase_node(NodeVector::const_iterator first)
Erase member to blocks.
AfterBlock(BABlock *bablock)
virtual void visit_param_block(ast::ParamBlock &node)=0
visit node of type ast::ParamBlock
void set_parent_in_children()
Set this object as parent for all the children.
void set_parent_in_children()
Set this object as parent for all the children.
RandomVarVector variables
Vector of random variables.
virtual void visit_nrn_state_block(ast::NrnStateBlock &node)=0
visit node of type ast::NrnStateBlock
virtual void visit_indexed_name(ast::IndexedName &node)=0
visit node of type ast::IndexedName
StatementVector::const_iterator insert_statement(StatementVector::const_iterator position, const std::shared_ptr< Statement > &n)
Insert member to statements.
virtual void visit_local_var(const ast::LocalVar &node)=0
visit node of type ast::LocalVar
std::shared_ptr< ModToken > token
token with location information
void set_name(std::shared_ptr< Name > &&name)
Setter for member variable ReadIonVar::name (rvalue reference)
std::shared_ptr< ModToken > token
token with location information
void set_variable_block(std::shared_ptr< StatementBlock > &&variable_block)
Setter for member variable EigenNewtonSolverBlock::variable_block (rvalue reference)
virtual void visit_nonspecific_cur_var(ast::NonspecificCurVar &node)=0
visit node of type ast::NonspecificCurVar
void set_parent_in_children()
Set this object as parent for all the children.
virtual bool is_integer() const noexcept
Check if the ast node is an instance of ast::Integer.
std::vector< std::shared_ptr< RandomVar > > RandomVarVector
void set_bablock(std::shared_ptr< BABlock > &&bablock)
Setter for member variable AfterBlock::bablock (rvalue reference)
std::shared_ptr< Expression > expression
TODO.
virtual bool is_bbcore_pointer() const noexcept
Check if the ast node is an instance of ast::BbcorePointer.
std::shared_ptr< ModToken > token
token with location information
virtual void visit_random_var_list(const ast::RandomVarList &node)=0
visit node of type ast::RandomVarList
void set_bablock(std::shared_ptr< BABlock > &&bablock)
Setter for member variable BeforeBlock::bablock (rvalue reference)
void visit_children(visitor::Visitor &v) override
visit children i.e.
virtual bool is_pointer() const noexcept
Check if the ast node is an instance of ast::Pointer.
virtual void visit_write_ion_var(ast::WriteIonVar &node)=0
visit node of type ast::WriteIonVar
virtual void visit_state_block(ast::StateBlock &node)=0
visit node of type ast::StateBlock
virtual void visit_ba_block_type(const ast::BABlockType &node)=0
visit node of type ast::BABlockType
virtual bool is_procedure_block() const noexcept
Check if the ast node is an instance of ast::ProcedureBlock.
BeforeBlock(BABlock *bablock)
std::shared_ptr< ModToken > token
token with location information
void set_writelist(WriteIonVarVector &&writelist)
Setter for member variable Useion::writelist (rvalue reference)
virtual void visit_function_table_block(const ast::FunctionTableBlock &node)=0
visit node of type ast::FunctionTableBlock
Represents TABLE statement in NMODL.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
std::shared_ptr< Integer > n_state_vars
number of state vars used in solve
void set_parent_in_children()
Set this object as parent for all the children.
virtual void visit_model(const ast::Model &node)=0
visit node of type ast::Model
void set_parent_in_children()
Set this object as parent for all the children.
void visit_children(visitor::Visitor &v) override
visit children i.e.
std::string get_node_name() const override
Return name of the node.
virtual bool is_protect_statement() const noexcept
Check if the ast node is an instance of ast::ProtectStatement.
virtual bool is_var_name() const noexcept
Check if the ast node is an instance of ast::VarName.
virtual void visit_assigned_block(const ast::AssignedBlock &node)=0
visit node of type ast::AssignedBlock
void set_parent_in_children()
Set this object as parent for all the children.
virtual void visit_number(ast::Number &node)=0
visit node of type ast::Number
virtual void visit_unit_state(const ast::UnitState &node)=0
visit node of type ast::UnitState
virtual void visit_wrapped_expression(ast::WrappedExpression &node)=0
visit node of type ast::WrappedExpression
Represents an integer variable.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
void set_op(BinaryOperator &&op)
Setter for member variable BinaryExpression::op (rvalue reference)
virtual void visit_float(ast::Float &node)=0
visit node of type ast::Float
void set_setup_x_block(std::shared_ptr< StatementBlock > &&setup_x_block)
Setter for member variable EigenNewtonSolverBlock::setup_x_block (rvalue reference)
Represents SUFFIX statement in NMODL.
std::shared_ptr< ModToken > token
token with location information
void set_name(std::shared_ptr< Name > &&name)
Setter for member variable KineticBlock::name (rvalue reference)
ExpressionVector arguments
TODO.
void set_parent_in_children()
Set this object as parent for all the children.
std::shared_ptr< ModToken > token
token with location information
void set_name(std::shared_ptr< Name > &&name)
Setter for member variable DiscreteBlock::name (rvalue reference)
void set_parent_in_children()
Set this object as parent for all the children.
Abstract Syntax Tree (AST) related implementations.
void set_parent_in_children()
Set this object as parent for all the children.
std::shared_ptr< ModToken > token
token with location information
virtual void visit_bbcore_pointer(ast::BbcorePointer &node)=0
visit node of type ast::BbcorePointer
virtual bool is_expression_statement() const noexcept
Check if the ast node is an instance of ast::ExpressionStatement.
Single variable of type RANDOM.
void set_parent_in_children()
Set this object as parent for all the children.
BABlockType(BAType value)
void set_n_odes(std::shared_ptr< Integer > &&n_odes)
Setter for member variable CvodeBlock::n_odes (rvalue reference)
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
void set_parent_in_children()
Set this object as parent for all the children.
virtual void visit_double(ast::Double &node)=0
visit node of type ast::Double
void set_lhs(std::shared_ptr< Expression > &&lhs)
Setter for member variable NonLinEquation::lhs (rvalue reference)
IfStatement(Expression *condition, StatementBlock *statement_block, const ElseIfStatementVector &elseifs, ElseStatement *elses)
std::shared_ptr< Expression > expression
TODO.
virtual void visit_electrode_cur_var(ast::ElectrodeCurVar &node)=0
visit node of type ast::ElectrodeCurVar
std::string get_node_name() const override
Return name of the node.
void visit_children(visitor::Visitor &v) override
visit children i.e.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
virtual void visit_derivative_block(const ast::DerivativeBlock &node)=0
visit node of type ast::DerivativeBlock
NameVector depend_vars
dependent variables
virtual bool is_extern_var() const noexcept
Check if the ast node is an instance of ast::ExternVar.
void set_name(std::shared_ptr< Name > &&name)
Setter for member variable NonspecificCurVar::name (rvalue reference)
virtual void visit_eigen_newton_solver_block(ast::EigenNewtonSolverBlock &node)=0
visit node of type ast::EigenNewtonSolverBlock
std::shared_ptr< StatementBlock > setup_x_block
update X from states
ArgumentVector parameters
Arguments to the for netcon block.
void visit_children(visitor::Visitor &v) override
visit children i.e.
void set_value(std::string value)
Setter for member variable Double::value.
std::shared_ptr< ModToken > token
token with location information
void visit_children(visitor::Visitor &v) override
visit children i.e.
void visit_children(visitor::Visitor &v) override
visit children i.e.
void visit_children(visitor::Visitor &v) override
visit children i.e.
std::shared_ptr< Number > max
TODO.
virtual void visit_else_if_statement(ast::ElseIfStatement &node)=0
visit node of type ast::ElseIfStatement
ConstantVar(Name *name, Number *value, Unit *unit)
std::shared_ptr< Unit > unit
Unit if specified.
std::shared_ptr< Unit > unit
TODO.
std::shared_ptr< ModToken > token
token with location information
std::shared_ptr< StatementBlock > statement_block
Block with statements vector.
void set_n_state_vars(std::shared_ptr< Integer > &&n_state_vars)
Setter for member variable EigenNewtonSolverBlock::n_state_vars (rvalue reference)
void visit_children(visitor::Visitor &v) override
visit children i.e.
void set_expression(std::shared_ptr< Expression > &&expression)
Setter for member variable UnaryExpression::expression (rvalue reference)
virtual bool is_function_block() const noexcept
Check if the ast node is an instance of ast::FunctionBlock.
std::string get_node_name() const override
Return name of the node.
void visit_children(visitor::Visitor &v) override
visit children i.e.
std::shared_ptr< Unit > unit
TODO.
void set_depend_vars(NameVector &&depend_vars)
Setter for member variable TableStatement::depend_vars (rvalue reference)
void set_value(std::shared_ptr< String > &&value)
Setter for member variable Name::value (rvalue reference)
void reset_global_var(GlobalVarVector::const_iterator position, GlobalVar *n)
Reset member to variables.
void visit_children(visitor::Visitor &v) override
visit children i.e.
virtual void visit_expression(ast::Expression &node)=0
visit node of type ast::Expression
virtual void visit_statement_block(ast::StatementBlock &node)=0
visit node of type ast::StatementBlock
std::shared_ptr< StatementBlock > initialize_block
Statement block to be executed before calling linear solver.
std::shared_ptr< Expression > from
TODO.
void set_parent_in_children()
Set this object as parent for all the children.
void set_expr(std::shared_ptr< Expression > &&expr)
Setter for member variable Conserve::expr (rvalue reference)
void set_name(std::shared_ptr< Name > &&name)
Setter for member variable ProcedureBlock::name (rvalue reference)
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
void visit_children(visitor::Visitor &v) override
visit children i.e.
void set_value(UnitStateType value)
Setter for member variable UnitState::value.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
StatementBlock(const StatementVector &statements)
DiffEqExpression(BinaryExpression *expression)
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
virtual void visit_double(const ast::Double &node)=0
visit node of type ast::Double
std::shared_ptr< ModToken > token
token with location information
void visit_children(visitor::Visitor &v) override
visit children i.e.
virtual void visit_block(const ast::Block &node)=0
visit node of type ast::Block
void visit_children(visitor::Visitor &v) override
visit children i.e.
void set_value(std::string value)
Setter for member variable String::value.
std::shared_ptr< ModToken > token
token with location information
virtual void visit_external(const ast::External &node)=0
visit node of type ast::External
NumberRange(Number *min, Number *max)
Represents a ASSIGNED block in the NMODL.
std::shared_ptr< Number > min
TODO.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
void set_parent_in_children()
Set this object as parent for all the children.
void set_parent_in_children()
Set this object as parent for all the children.
std::shared_ptr< Name > name
Name of the longitudinal diffusion block.
std::string get_node_name() const override
Return name of the node.
std::shared_ptr< ModToken > token
token with location information
void visit_children(visitor::Visitor &v) override
visit children i.e.
void set_arguments(ExpressionVector &&arguments)
Setter for member variable FunctionCall::arguments (rvalue reference)
std::shared_ptr< StatementBlock > functor_block
odes as functor for eigen
void visit_children(visitor::Visitor &v) override
visit children i.e.
AssignedDefinitionVector definitions
Vector of state variables.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
std::string get_node_name() const override
Return name of the node.
virtual void visit_name(const ast::Name &node)=0
visit node of type ast::Name
void set_parent_in_children()
Set this object as parent for all the children.
virtual bool is_constant_var() const noexcept
Check if the ast node is an instance of ast::ConstantVar.
std::shared_ptr< Expression > expression
Expression that is being wrapped.
std::shared_ptr< String > value
Name of prime variable.
StatementVector solve_statements
solve blocks to be called or generated
virtual bool is_reaction_statement() const noexcept
Check if the ast node is an instance of ast::ReactionStatement.
virtual void visit_assigned_definition(ast::AssignedDefinition &node)=0
visit node of type ast::AssignedDefinition
void set_condition(std::shared_ptr< Expression > &&condition)
Setter for member variable WhileStatement::condition (rvalue reference)
RandomVarList(const RandomVarVector &variables)
Represent newton solver solution block based on Eigen.
virtual bool is_global_var() const noexcept
Check if the ast node is an instance of ast::GlobalVar.
virtual void visit_protect_statement(const ast::ProtectStatement &node)=0
visit node of type ast::ProtectStatement
void visit_children(visitor::Visitor &v) override
visit children i.e.
std::vector< std::shared_ptr< LocalVar > > LocalVarVector
std::vector< std::shared_ptr< GlobalVar > > GlobalVarVector
std::shared_ptr< Unit > unit2
TODO.
void set_value(std::shared_ptr< Number > &&value)
Setter for member variable ParamAssign::value (rvalue reference)
virtual void visit_read_ion_var(const ast::ReadIonVar &node)=0
visit node of type ast::ReadIonVar
std::shared_ptr< String > ontology_id
Ontology name.
ElseStatement(StatementBlock *statement_block)
void set_parent_in_children()
Set this object as parent for all the children.
void visit_children(visitor::Visitor &v) override
visit children i.e.
ProtectStatement(Expression *expression)
virtual void visit_param_block(const ast::ParamBlock &node)=0
visit node of type ast::ParamBlock
void set_parent_in_children()
Set this object as parent for all the children.
virtual void visit_line_comment(const ast::LineComment &node)=0
visit node of type ast::LineComment
std::shared_ptr< StatementBlock > statement_block
Block with statements vector.
void visit_children(visitor::Visitor &v) override
visit children i.e.
virtual void visit_thread_safe(const ast::ThreadSafe &node)=0
visit node of type ast::ThreadSafe
std::shared_ptr< Name > name
Name of the macro.
void visit_children(visitor::Visitor &v) override
visit children i.e.
void set_name(std::shared_ptr< Name > &&name)
Setter for member variable BbcorePointerVar::name (rvalue reference)
virtual void visit_bbcore_pointer_var(const ast::BbcorePointerVar &node)=0
visit node of type ast::BbcorePointerVar
virtual void visit_if_statement(ast::IfStatement &node)=0
visit node of type ast::IfStatement
virtual void visit_binary_operator(const ast::BinaryOperator &node)=0
visit node of type ast::BinaryOperator
std::shared_ptr< ModToken > token
token with location information
void set_unit1(std::shared_ptr< Unit > &&unit1)
Setter for member variable UnitDef::unit1 (rvalue reference)
void set_name(std::shared_ptr< Name > &&name)
Setter for member variable LinearBlock::name (rvalue reference)
std::shared_ptr< StatementBlock > stiff_block
Block with statements of the form Dvar = Dvar / (1 - dt * J(f)), used for updating stiff systems.
void visit_children(visitor::Visitor &v) override
visit children i.e.
virtual bool is_nrn_state_block() const noexcept
Check if the ast node is an instance of ast::NrnStateBlock.
virtual void visit_double_unit(const ast::DoubleUnit &node)=0
visit node of type ast::DoubleUnit
void set_name(std::shared_ptr< Identifier > &&name)
Setter for member variable VarName::name (rvalue reference)
void set_length(std::shared_ptr< Integer > &&length)
Setter for member variable AssignedDefinition::length (rvalue reference)
std::shared_ptr< ModToken > token
token with location information
NameVector species
The names of the species that reside in this compartment.
void set_parent_in_children()
Set this object as parent for all the children.
virtual bool is_assigned_definition() const noexcept
Check if the ast node is an instance of ast::AssignedDefinition.
std::string get_node_name() const override
Return name of the node.
void visit_children(visitor::Visitor &v) override
visit children i.e.
void visit_children(visitor::Visitor &v) override
visit children i.e.
std::shared_ptr< ModToken > token
token with location information
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
std::string get_node_name() const override
Return name of the node.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
NameVector variables
List of variable that should be independent.
std::shared_ptr< ModToken > token
token with location information
void set_with(std::shared_ptr< Integer > &&with)
Setter for member variable TableStatement::with (rvalue reference)
std::string get_node_name() const override
Return name of the node.
int value
Value of boolean.
NameVector species
Names of the diffusing species.
PointerVarVector::const_iterator insert_pointer_var(PointerVarVector::const_iterator position, const std::shared_ptr< PointerVar > &n)
Insert member to variables.
virtual void visit_write_ion_var(const ast::WriteIonVar &node)=0
visit node of type ast::WriteIonVar
void visit_children(visitor::Visitor &v) override
visit children i.e.
std::shared_ptr< ModToken > token
token with location information
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
std::shared_ptr< Name > ion
Ion name.
virtual void visit_diff_eq_expression(const ast::DiffEqExpression &node)=0
visit node of type ast::DiffEqExpression
std::shared_ptr< ModToken > token
token with location information
virtual void visit_initial_block(const ast::InitialBlock &node)=0
visit node of type ast::InitialBlock
std::shared_ptr< Name > name
TODO.
void visit_children(visitor::Visitor &v) override
visit children i.e.
virtual void visit_identifier(ast::Identifier &node)=0
visit node of type ast::Identifier
void set_parent_in_children()
Set this object as parent for all the children.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
ArgumentVector parameters
Vector of the parameters.
Base class for all block scoped nodes.
void set_species(NameVector &&species)
Setter for member variable LonDiffuse::species (rvalue reference)
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
virtual void visit_function_block(const ast::FunctionBlock &node)=0
visit node of type ast::FunctionBlock
void visit_children(visitor::Visitor &v) override
visit children i.e.
virtual void visit_binary_expression(ast::BinaryExpression &node)=0
visit node of type ast::BinaryExpression
Represents a INITIAL block in the NMODL.
FunctionCall(Name *name, const ExpressionVector &arguments)
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
std::shared_ptr< ModToken > token
token with location information
void set_rate(std::shared_ptr< Expression > &&rate)
Setter for member variable LonDiffuse::rate (rvalue reference)
void visit_children(visitor::Visitor &v) override
visit children i.e.
std::shared_ptr< ModToken > token
token with location information
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
virtual void set_parent(Ast *p)
Parent setter.
void set_solvefor(NameVector &&solvefor)
Setter for member variable NonLinearBlock::solvefor (rvalue reference)
void set_parent_in_children()
Set this object as parent for all the children.
std::vector< std::shared_ptr< ReadIonVar > > ReadIonVarVector
virtual void visit_independent_block(ast::IndependentBlock &node)=0
visit node of type ast::IndependentBlock
std::shared_ptr< StatementBlock > finalize_block
Statement block to be executed after calling linear solver.
virtual bool is_random_var_list() const noexcept
Check if the ast node is an instance of ast::RandomVarList.
std::string get_node_name() const override
Return name of the node.
std::shared_ptr< Double > value
Value of new timestep.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
void reset_local_var(LocalVarVector::const_iterator position, LocalVar *n)
Reset member to variables.
ConstantBlock(const ConstantStatementVector &statements)
virtual bool is_function_table_block() const noexcept
Check if the ast node is an instance of ast::FunctionTableBlock.
std::shared_ptr< Expression > condition
TODO.
std::vector< std::shared_ptr< ElseIfStatement > > ElseIfStatementVector
std::shared_ptr< Expression > value
TODO.
virtual bool is_mutex_lock() const noexcept
Check if the ast node is an instance of ast::MutexLock.
std::shared_ptr< ModToken > token
token with location information
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
NeuronBlock(StatementBlock *statement_block)
std::shared_ptr< ModToken > token
token with location information
virtual void visit_unit_block(ast::UnitBlock &node)=0
visit node of type ast::UnitBlock
std::shared_ptr< Expression > expr
TODO.
virtual void visit_conserve(ast::Conserve &node)=0
visit node of type ast::Conserve
virtual bool is_lag_statement() const noexcept
Check if the ast node is an instance of ast::LagStatement.
std::shared_ptr< ModToken > token
token with location information
virtual void visit_pointer(const ast::Pointer &node)=0
visit node of type ast::Pointer
std::string value
Value of string.
virtual void visit_include(const ast::Include &node)=0
visit node of type ast::Include
virtual bool is_ba_block_type() const noexcept
Check if the ast node is an instance of ast::BABlockType.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
virtual bool is_if_statement() const noexcept
Check if the ast node is an instance of ast::IfStatement.
void visit_children(visitor::Visitor &v) override
visit children i.e.
std::shared_ptr< ModToken > token
token with location information
void visit_children(visitor::Visitor &v) override
visit children i.e.
std::vector< std::shared_ptr< Name > > NameVector
std::shared_ptr< Number > from
TODO.
virtual void set_name(const std::string &name)
Set name for the AST node.
virtual void visit_independent_block(const ast::IndependentBlock &node)=0
visit node of type ast::IndependentBlock
virtual bool is_diff_eq_expression() const noexcept
Check if the ast node is an instance of ast::DiffEqExpression.
virtual void visit_integer(const ast::Integer &node)=0
visit node of type ast::Integer
std::shared_ptr< Identifier > name
Name of the variable (TODO)
void set_from(std::shared_ptr< Expression > &&from)
Setter for member variable TableStatement::from (rvalue reference)
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
void set_readlist(ReadIonVarVector &&readlist)
Setter for member variable Useion::readlist (rvalue reference)
BbcorePointerVarVector variables
Vector of bbcore pointer variables.
void set_parent_in_children()
Set this object as parent for all the children.
void set_rhs(std::shared_ptr< Expression > &&rhs)
Setter for member variable BinaryExpression::rhs (rvalue reference)
std::shared_ptr< Name > name
TODO.
void set_parent_in_children()
Set this object as parent for all the children.
virtual void visit_for_netcon(ast::ForNetcon &node)=0
visit node of type ast::ForNetcon
Represent WATCH statement in NMODL.
virtual void visit_statement_block(const ast::StatementBlock &node)=0
visit node of type ast::StatementBlock
void set_name(const std::string &name) override
Set name for the current ast node.
virtual bool is_discrete_block() const noexcept
Check if the ast node is an instance of ast::DiscreteBlock.
virtual void visit_constant_block(const ast::ConstantBlock &node)=0
visit node of type ast::ConstantBlock
virtual void visit_program(const ast::Program &node)=0
visit node of type ast::Program
std::shared_ptr< Name > steadystate
Name of the integration method.
Include(String *filename, const NodeVector &blocks)
void set_value(BAType value)
Setter for member variable BABlockType::value.
virtual void visit_verbatim(const ast::Verbatim &node)=0
visit node of type ast::Verbatim
Global(const GlobalVarVector &variables)
void set_condition(std::shared_ptr< Expression > &&condition)
Setter for member variable IfStatement::condition (rvalue reference)
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
Represents a float variable.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
std::shared_ptr< StatementBlock > statement_block
Block with statements vector.
Represents a BREAKPOINT block in NMODL.
void visit_children(visitor::Visitor &v) override
visit children i.e.
virtual void visit_breakpoint_block(const ast::BreakpointBlock &node)=0
visit node of type ast::BreakpointBlock
virtual bool is_name() const noexcept
Check if the ast node is an instance of ast::Name.
std::string get_node_name() const override
Return name of the node.
virtual bool is_verbatim() const noexcept
Check if the ast node is an instance of ast::Verbatim.
std::shared_ptr< ModToken > token
token with location information
void set_name(std::shared_ptr< Name > &&name)
Setter for member variable Suffix::name (rvalue reference)
virtual void visit_before_block(const ast::BeforeBlock &node)=0
visit node of type ast::BeforeBlock
virtual void visit_while_statement(ast::WhileStatement &node)=0
visit node of type ast::WhileStatement
virtual void visit_electrode_cur_var(const ast::ElectrodeCurVar &node)=0
visit node of type ast::ElectrodeCurVar
std::shared_ptr< Name > name
Name of the block.
std::shared_ptr< Unit > unit
TODO.
std::shared_ptr< Identifier > name
Name of the variable.
void visit_children(visitor::Visitor &v) override
visit children i.e.
Represents specific element of an array variable.
void set_statements(ConstantStatementVector &&statements)
Setter for member variable ConstantBlock::statements (rvalue reference)
std::vector< std::shared_ptr< Argument > > ArgumentVector
void visit_children(visitor::Visitor &v) override
visit children i.e.
virtual void visit_net_receive_block(ast::NetReceiveBlock &node)=0
visit node of type ast::NetReceiveBlock
void set_parent_in_children()
Set this object as parent for all the children.
std::shared_ptr< Name > name
Name of the a RANDOM variable.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
void visit_children(visitor::Visitor &v) override
visit children i.e.
virtual symtab::SymbolTable * get_symbol_table() const
Return associated symbol table for the AST node.
std::shared_ptr< BABlock > bablock
Block to be called after.
WrappedExpression(Expression *expression)
std::shared_ptr< StatementBlock > statement_block
Block with statements vector.
virtual void visit_solution_expression(ast::SolutionExpression &node)=0
visit node of type ast::SolutionExpression
void set_functor_block(std::shared_ptr< StatementBlock > &&functor_block)
Setter for member variable EigenNewtonSolverBlock::functor_block (rvalue reference)
virtual void visit_derivimplicit_callback(ast::DerivimplicitCallback &node)=0
visit node of type ast::DerivimplicitCallback
void set_parent_in_children()
Set this object as parent for all the children.
virtual void visit_valence(const ast::Valence &node)=0
visit node of type ast::Valence
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
void emplace_back_local_var(LocalVar *n)
Add member to variables by raw pointer.
StatementVector::const_iterator erase_statement(StatementVector::const_iterator first)
Erase member to statements.
std::shared_ptr< Name > name
Name of the linear block.
void set_from(std::shared_ptr< Number > &&from)
Setter for member variable AssignedDefinition::from (rvalue reference)
virtual void visit_solve_block(ast::SolveBlock &node)=0
visit node of type ast::SolveBlock
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
virtual bool is_update_dt() const noexcept
Check if the ast node is an instance of ast::UpdateDt.
std::vector< std::shared_ptr< BbcorePointerVar > > BbcorePointerVarVector
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
Represents a DESTRUCTOR block in the NMODL.
DerivimplicitCallback(Block *node_to_solve)
void set_lhs(std::shared_ptr< Expression > &&lhs)
Setter for member variable BinaryExpression::lhs (rvalue reference)
Abstract base class for all visitors implementation.
virtual bool is_pointer_var() const noexcept
Check if the ast node is an instance of ast::PointerVar.
void set_parent_in_children()
Set this object as parent for all the children.
FromStatement(Name *name, Expression *from, Expression *to, Expression *increment, StatementBlock *statement_block)
std::shared_ptr< ModToken > token
token with location information
void set_parent_in_children()
Set this object as parent for all the children.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
void visit_children(visitor::Visitor &v) override
visit children i.e.
virtual bool is_valence() const noexcept
Check if the ast node is an instance of ast::Valence.
void visit_children(visitor::Visitor &v) override
visit children i.e.
TableStatement(const NameVector &table_vars, const NameVector &depend_vars, Expression *from, Expression *to, Integer *with)
void visit_children(visitor::Visitor &v) override
visit children i.e.
void visit_children(visitor::Visitor &v) override
visit children i.e.
BABlock(BABlockType *type, StatementBlock *statement_block)
LocalVarVector::const_iterator insert_local_var(LocalVarVector::const_iterator position, const std::shared_ptr< LocalVar > &n)
Insert member to variables.
virtual bool is_else_if_statement() const noexcept
Check if the ast node is an instance of ast::ElseIfStatement.
virtual void visit_var_name(const ast::VarName &node)=0
visit node of type ast::VarName
void set_index_name(std::shared_ptr< Name > &&index_name)
Setter for member variable LonDiffuse::index_name (rvalue reference)
void set_index(std::shared_ptr< Expression > &&index)
Setter for member variable VarName::index (rvalue reference)
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
void set_gt(std::shared_ptr< Boolean > &>)
Setter for member variable FactorDef::gt (rvalue reference)
virtual bool is_table_statement() const noexcept
Check if the ast node is an instance of ast::TableStatement.
void set_block_name(std::shared_ptr< Name > &&block_name)
Setter for member variable SolveBlock::block_name (rvalue reference)
virtual void visit_param_assign(const ast::ParamAssign &node)=0
visit node of type ast::ParamAssign
virtual bool is_unit() const noexcept
Check if the ast node is an instance of ast::Unit.
Represents a CONSTRUCTOR block in the NMODL.
virtual bool is_thread_safe() const noexcept
Check if the ast node is an instance of ast::ThreadSafe.
ElseIfStatementVector elseifs
TODO.
virtual void visit_line_comment(ast::LineComment &node)=0
visit node of type ast::LineComment
void set_parent_in_children()
Set this object as parent for all the children.
virtual void visit_table_statement(const ast::TableStatement &node)=0
visit node of type ast::TableStatement
void set_parent_in_children()
Set this object as parent for all the children.
void set_limit(std::shared_ptr< Limits > &&limit)
Setter for member variable ParamAssign::limit (rvalue reference)
virtual void visit_lin_equation(const ast::LinEquation &node)=0
visit node of type ast::LinEquation
NonLinEquation(Expression *lhs, Expression *rhs)
void set_min(std::shared_ptr< Number > &&min)
Setter for member variable NumberRange::min (rvalue reference)
void visit_children(visitor::Visitor &v) override
visit children i.e.
Extracts information required for LONGITUDINAL_DIFFUSION for each KINETIC block.
virtual void visit_unit_def(ast::UnitDef &node)=0
visit node of type ast::UnitDef
void set_parent_in_children()
Set this object as parent for all the children.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
virtual bool is_longitudinal_diffusion_block() const noexcept
Check if the ast node is an instance of ast::LongitudinalDiffusionBlock.
void emplace_back_assigned_definition(AssignedDefinition *n)
Add member to definitions by raw pointer.
virtual void visit_lag_statement(const ast::LagStatement &node)=0
visit node of type ast::LagStatement
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
virtual void visit_factor_def(const ast::FactorDef &node)=0
visit node of type ast::FactorDef
void set_non_stiff_block(std::shared_ptr< StatementBlock > &&non_stiff_block)
Setter for member variable CvodeBlock::non_stiff_block (rvalue reference)
void set_unit(std::shared_ptr< Unit > &&unit)
Setter for member variable Argument::unit (rvalue reference)
void visit_children(visitor::Visitor &v) override
visit children i.e.
virtual bool is_double() const noexcept
Check if the ast node is an instance of ast::Double.
virtual Ast * clone() const
Create a copy of the current node.
std::string get_node_name() const override
Return name of the node.
std::shared_ptr< StatementBlock > variable_block
Statements to be declared in the functor.
std::shared_ptr< StatementBlock > statement_block
TODO.
std::shared_ptr< ModToken > token
token with location information
std::string get_node_name() const override
Return name of the node.
std::string get_node_name() const override
Return name of the node.
NameVector solvefor
Solve for specification (TODO)
void visit_children(visitor::Visitor &v) override
visit children i.e.
virtual void visit_read_ion_var(ast::ReadIonVar &node)=0
visit node of type ast::ReadIonVar
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
virtual void visit_unary_operator(const ast::UnaryOperator &node)=0
visit node of type ast::UnaryOperator
std::shared_ptr< StatementBlock > statement_block
Block with statements vector.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
virtual void visit_factor_def(ast::FactorDef &node)=0
visit node of type ast::FactorDef
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
virtual void visit_bbcore_pointer_var(ast::BbcorePointerVar &node)=0
visit node of type ast::BbcorePointerVar
void set_definitions(ExpressionVector &&definitions)
Setter for member variable UnitBlock::definitions (rvalue reference)
std::string get_node_name() const override
Return name of the node.
std::shared_ptr< Name > name
Name of the procedure.
std::shared_ptr< ModToken > token
token with location information
std::shared_ptr< Unit > unit
Unit of the argument.
virtual bool is_number() const noexcept
Check if the ast node is an instance of ast::Number.
virtual bool is_model() const noexcept
Check if the ast node is an instance of ast::Model.
Represents USEION statement in NMODL.
std::shared_ptr< StatementBlock > variable_block
Statements to be declared in the functor.
void visit_children(visitor::Visitor &v) override
visit children i.e.
std::shared_ptr< ModToken > token
token with location information
void set_at(std::shared_ptr< Integer > &&at)
Setter for member variable VarName::at (rvalue reference)
EigenLinearSolverBlock(Integer *n_state_vars, StatementBlock *variable_block, StatementBlock *initialize_block, StatementBlock *setup_x_block, StatementBlock *update_states_block, StatementBlock *finalize_block)
std::shared_ptr< Identifier > name
Name of array variable.
virtual void visit_global_var(const ast::GlobalVar &node)=0
visit node of type ast::GlobalVar
virtual void visit_unary_expression(ast::UnaryExpression &node)=0
visit node of type ast::UnaryExpression
virtual void negate()
Negate the value of AST node.
virtual void visit_pointer_var(const ast::PointerVar &node)=0
visit node of type ast::PointerVar
std::shared_ptr< ModToken > token
token with location information
virtual void visit_destructor_block(ast::DestructorBlock &node)=0
visit node of type ast::DestructorBlock
void set_name(std::shared_ptr< Name > &&name)
Setter for member variable WriteIonVar::name (rvalue reference)
void visit_children(visitor::Visitor &v) override
visit children i.e.
void set_parent_in_children()
Set this object as parent for all the children.
virtual bool is_ast() const noexcept
Check if the ast node is an instance of ast::Ast.
void set_blocks(NodeVector &&blocks)
Setter for member variable Include::blocks (rvalue reference)
void set_solvefor(NameVector &&solvefor)
Setter for member variable LinearBlock::solvefor (rvalue reference)
Float(const std::string &value)
void set_parent_in_children()
Set this object as parent for all the children.
std::shared_ptr< Unit > unit2
TODO.
virtual void visit_random_var(const ast::RandomVar &node)=0
visit node of type ast::RandomVar
void set_parent_in_children()
Set this object as parent for all the children.
void visit_children(visitor::Visitor &v) override
visit children i.e.
std::shared_ptr< ModToken > token
token with location information
virtual bool is_block_comment() const noexcept
Check if the ast node is an instance of ast::BlockComment.
Nonspecific(const NonspecificCurVarVector ¤ts)
void visit_children(visitor::Visitor &v) override
visit children i.e.
virtual void visit_reaction_operator(ast::ReactionOperator &node)=0
visit node of type ast::ReactionOperator
virtual bool is_initial_block() const noexcept
Check if the ast node is an instance of ast::InitialBlock.
std::shared_ptr< ModToken > token
token with location information
virtual void visit_identifier(const ast::Identifier &node)=0
visit node of type ast::Identifier
void emplace_back_pointer_var(PointerVar *n)
Add member to variables by raw pointer.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
virtual void visit_electrode_current(const ast::ElectrodeCurrent &node)=0
visit node of type ast::ElectrodeCurrent
void set_type(std::shared_ptr< BABlockType > &&type)
Setter for member variable BABlock::type (rvalue reference)
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
void visit_children(visitor::Visitor &v) override
visit children i.e.
std::shared_ptr< Name > name
Name of the function.
std::shared_ptr< ModToken > token
token with location information
std::shared_ptr< Name > name
Name of ion.
std::shared_ptr< ModToken > token
token with location information
void set_parent_in_children()
Set this object as parent for all the children.
virtual void visit_include(ast::Include &node)=0
visit node of type ast::Include
ReactionStatement(Expression *reaction1, const ReactionOperator &op, Expression *reaction2, Expression *expression1, Expression *expression2)
virtual bool is_write_ion_var() const noexcept
Check if the ast node is an instance of ast::WriteIonVar.
void set_value(std::shared_ptr< String > &&value)
Setter for member variable PrimeName::value (rvalue reference)
virtual void visit_while_statement(const ast::WhileStatement &node)=0
visit node of type ast::WhileStatement
virtual void visit_solve_block(const ast::SolveBlock &node)=0
visit node of type ast::SolveBlock
std::shared_ptr< Expression > to
to values
virtual bool is_bbcore_pointer_var() const noexcept
Check if the ast node is an instance of ast::BbcorePointerVar.
void visit_children(visitor::Visitor &v) override
visit children i.e.
std::shared_ptr< Name > method
Name of the integration method.
virtual void visit_net_receive_block(const ast::NetReceiveBlock &node)=0
visit node of type ast::NetReceiveBlock
LocalListStatement(const LocalVarVector &variables)
void set_name(std::shared_ptr< Name > &&name)
Setter for member variable ElectrodeCurVar::name (rvalue reference)
std::shared_ptr< ModToken > token
token with location information
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
void set_name(std::shared_ptr< Identifier > &&name)
Setter for member variable AssignedDefinition::name (rvalue reference)
void set_lhs(std::shared_ptr< Expression > &&lhs)
Setter for member variable LinEquation::lhs (rvalue reference)
virtual void visit_extern_var(ast::ExternVar &node)=0
visit node of type ast::ExternVar
WriteIonVarVector writelist
Variables being written.
std::shared_ptr< ModToken > token
token with location information
std::shared_ptr< ModToken > token
token with location information
void visit_children(visitor::Visitor &v) override
visit children i.e.
void visit_children(visitor::Visitor &v) override
visit children i.e.
virtual void visit_compartment(const ast::Compartment &node)=0
visit node of type ast::Compartment
void set_parent_in_children()
Set this object as parent for all the children.
void set_parent_in_children()
Set this object as parent for all the children.
NonspecificCurVar(Name *name)
void set_unit(std::shared_ptr< Unit > &&unit)
Setter for member variable ProcedureBlock::unit (rvalue reference)
void visit_children(visitor::Visitor &v) override
visit children i.e.
std::shared_ptr< Number > value
TODO.
virtual void visit_limits(ast::Limits &node)=0
visit node of type ast::Limits
void set_increment(std::shared_ptr< Expression > &&increment)
Setter for member variable FromStatement::increment (rvalue reference)
void set_parent_in_children()
Set this object as parent for all the children.
void set_longitudinal_diffusion_statements(std::shared_ptr< StatementBlock > &&longitudinal_diffusion_statements)
Setter for member variable LongitudinalDiffusionBlock::longitudinal_diffusion_statements (rvalue refe...
std::shared_ptr< Name > block_name
Name of the block to solve.
Represents GLOBAL statement in NMODL.
virtual void visit_name(ast::Name &node)=0
visit node of type ast::Name
std::shared_ptr< Double > value
TODO.
void set_ontology_id(std::shared_ptr< String > &&ontology_id)
Setter for member variable Useion::ontology_id (rvalue reference)
ElectrodeCurVar(Name *name)
void set_expression(std::shared_ptr< Expression > &&expression)
Setter for member variable WrappedExpression::expression (rvalue reference)
virtual bool is_electrode_cur_var() const noexcept
Check if the ast node is an instance of ast::ElectrodeCurVar.
virtual void visit_argument(const ast::Argument &node)=0
visit node of type ast::Argument
WatchVector::const_iterator insert_watch(WatchVector::const_iterator position, const std::shared_ptr< Watch > &n)
Insert member to statements.
virtual bool is_watch_statement() const noexcept
Check if the ast node is an instance of ast::WatchStatement.
void visit_children(visitor::Visitor &v) override
visit children i.e.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
std::shared_ptr< Expression > rhs
Right-hand-side of the equation.
void set_parent_in_children()
Set this object as parent for all the children.
virtual bool is_random_var() const noexcept
Check if the ast node is an instance of ast::RandomVar.
void set_parent_in_children()
Set this object as parent for all the children.
virtual void visit_watch(const ast::Watch &node)=0
visit node of type ast::Watch
void set_variable_block(std::shared_ptr< StatementBlock > &&variable_block)
Setter for member variable EigenLinearSolverBlock::variable_block (rvalue reference)
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
void visit_children(visitor::Visitor &v) override
visit children i.e.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
std::shared_ptr< ModToken > token
token with location information
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
virtual void visit_limits(const ast::Limits &node)=0
visit node of type ast::Limits
virtual bool is_constant_statement() const noexcept
Check if the ast node is an instance of ast::ConstantStatement.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
void visit_children(visitor::Visitor &v) override
visit children i.e.
virtual bool is_from_statement() const noexcept
Check if the ast node is an instance of ast::FromStatement.
void visit_children(visitor::Visitor &v) override
visit children i.e.
virtual void visit_program(ast::Program &node)=0
visit node of type ast::Program
std::shared_ptr< ModToken > token
token with location information
Represent CONSTANT block in the mod file.
std::string get_node_name() const override
Return name of the node.
std::shared_ptr< StatementBlock > statement_block
Block with statements vector.
std::shared_ptr< SolveBlock > solve_block
std::string value
Value of float.
void visit_children(visitor::Visitor &v) override
visit children i.e.
std::shared_ptr< StatementBlock > finalize_block
Statement block to be executed after calling newton solver.
virtual void visit_unit_state(ast::UnitState &node)=0
visit node of type ast::UnitState
Represents a prime variable (for ODE)
void visit_children(visitor::Visitor &v) override
visit children i.e.
void emplace_back_statement(Statement *n)
Add member to statements by raw pointer.
GlobalVarVector variables
Vector of global variables.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
void visit_children(visitor::Visitor &v) override
visit children i.e.
virtual bool is_state_block() const noexcept
Check if the ast node is an instance of ast::StateBlock.
virtual void visit_procedure_block(const ast::ProcedureBlock &node)=0
visit node of type ast::ProcedureBlock
Represents DERIVATIVE block in the NMODL.
virtual const ModToken * get_token() const
Return associated token for the AST node.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
void set_name(std::shared_ptr< Name > &&name)
Setter for member variable RandomVar::name (rvalue reference)
std::shared_ptr< BinaryExpression > expression
Differential Expression.
void set_value(std::shared_ptr< Double > &&value)
Setter for member variable DoubleUnit::value (rvalue reference)
virtual void visit_constant_statement(const ast::ConstantStatement &node)=0
visit node of type ast::ConstantStatement
std::shared_ptr< ModToken > token
token with location information
virtual void visit_from_statement(ast::FromStatement &node)=0
visit node of type ast::FromStatement
std::shared_ptr< Integer > at
Value specified with @
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
virtual bool is_neuron_block() const noexcept
Check if the ast node is an instance of ast::NeuronBlock.
std::shared_ptr< Name > index_name
Name of the index variable in volume expression.
DerivativeBlock(Name *name, StatementBlock *statement_block)
NonspecificCurVarVector currents
Vector of non specific variables.
virtual void visit_non_lin_equation(const ast::NonLinEquation &node)=0
visit node of type ast::NonLinEquation
void set_parent_in_children()
Set this object as parent for all the children.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
std::shared_ptr< ModToken > token
token with location information
Represents a boolean variable.
void set_setup_x_block(std::shared_ptr< StatementBlock > &&setup_x_block)
Setter for member variable EigenLinearSolverBlock::setup_x_block (rvalue reference)
virtual void visit_node(const ast::Node &node)=0
visit node of type ast::Node
Limits(Number *min, Number *max)
std::shared_ptr< ModToken > token
token with location information
virtual void visit_nonspecific(const ast::Nonspecific &node)=0
visit node of type ast::Nonspecific
virtual bool is_watch() const noexcept
Check if the ast node is an instance of ast::Watch.
std::shared_ptr< Expression > reaction1
TODO.
Represent symbol table for a NMODL block.
std::vector< std::shared_ptr< ElectrodeCurVar > > ElectrodeCurVarVector
std::shared_ptr< Number > value
Value of the constant.
void set_index_name(std::shared_ptr< Name > &&index_name)
Setter for member variable Compartment::index_name (rvalue reference)
void set_variables(RandomVarVector &&variables)
Setter for member variable RandomVarList::variables (rvalue reference)
void set_statement(std::shared_ptr< String > &&statement)
Setter for member variable Verbatim::statement (rvalue reference)
Conserve(Expression *react, Expression *expr)
virtual bool is_mutex_unlock() const noexcept
Check if the ast node is an instance of ast::MutexUnlock.
virtual void visit_compartment(ast::Compartment &node)=0
visit node of type ast::Compartment
void set_parent_in_children()
Set this object as parent for all the children.
virtual void visit_mutex_lock(const ast::MutexLock &node)=0
visit node of type ast::MutexLock
void set_conductance(std::shared_ptr< Name > &&conductance)
Setter for member variable ConductanceHint::conductance (rvalue reference)
void set_parent_in_children()
Set this object as parent for all the children.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
void visit_children(visitor::Visitor &v) override
visit children i.e.
virtual void visit_block_comment(ast::BlockComment &node)=0
visit node of type ast::BlockComment
virtual void visit_derivimplicit_callback(const ast::DerivimplicitCallback &node)=0
visit node of type ast::DerivimplicitCallback
virtual void visit_suffix(ast::Suffix &node)=0
visit node of type ast::Suffix
virtual void visit_neuron_block(ast::NeuronBlock &node)=0
visit node of type ast::NeuronBlock
void set_name(std::shared_ptr< Name > &&name)
Setter for member variable NonLinearBlock::name (rvalue reference)
void set_name(std::shared_ptr< Identifier > &&name)
Setter for member variable LagStatement::name (rvalue reference)
void visit_children(visitor::Visitor &v) override
visit children i.e.
Represent linear solver solution block based on Eigen.
std::shared_ptr< StatementBlock > initialize_block
Statement block to be executed before calling newton solver.
void set_stiff_block(std::shared_ptr< StatementBlock > &&stiff_block)
Setter for member variable CvodeBlock::stiff_block (rvalue reference)
virtual bool is_breakpoint_block() const noexcept
Check if the ast node is an instance of ast::BreakpointBlock.
std::shared_ptr< ModToken > token
token with location information
std::shared_ptr< Expression > condition
TODO.
virtual void visit_global_var(ast::GlobalVar &node)=0
visit node of type ast::GlobalVar
virtual bool is_wrapped_expression() const noexcept
Check if the ast node is an instance of ast::WrappedExpression.
virtual bool is_binary_expression() const noexcept
Check if the ast node is an instance of ast::BinaryExpression.
virtual bool is_expression() const noexcept
Check if the ast node is an instance of ast::Expression.
void visit_children(visitor::Visitor &v) override
visit children i.e.
std::shared_ptr< ModToken > token
token with location information
void set_solve_statements(StatementVector &&solve_statements)
Setter for member variable NrnStateBlock::solve_statements (rvalue reference)
void set_volume(std::shared_ptr< Expression > &&volume)
Setter for member variable Compartment::volume (rvalue reference)
Define(Name *name, Integer *value)
std::string get_node_name() const override
Return name of the node.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
Represents a LAG statement in the mod file.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
Represent COMPARTMENT statement in NMODL.
std::shared_ptr< String > filename
path to the file to include
virtual void visit_number_range(ast::NumberRange &node)=0
visit node of type ast::NumberRange
virtual void visit_constant_var(ast::ConstantVar &node)=0
visit node of type ast::ConstantVar
void visit_children(visitor::Visitor &v) override
visit children i.e.
std::string get_node_name() const override
Return name of the node.
void set_parent_in_children()
Set this object as parent for all the children.
Statement to indicate a change in timestep in a given block.
ArgumentVector parameters
Vector of the parameters.
std::shared_ptr< ModToken > token
token with location information
LocalVarVector variables
TODO.
virtual void visit_param_assign(ast::ParamAssign &node)=0
visit node of type ast::ParamAssign
std::shared_ptr< Expression > lhs
LHS of the binary expression.
virtual void visit_neuron_block(const ast::NeuronBlock &node)=0
visit node of type ast::NeuronBlock
void visit_children(visitor::Visitor &v) override
visit children i.e.
virtual void visit_bbcore_pointer(const ast::BbcorePointer &node)=0
visit node of type ast::BbcorePointer
void visit_children(visitor::Visitor &v) override
visit children i.e.
virtual void visit_prime_name(ast::PrimeName &node)=0
visit node of type ast::PrimeName
virtual bool is_react_var_name() const noexcept
Check if the ast node is an instance of ast::ReactVarName.
std::shared_ptr< Block > node_to_solve
Block to be solved (typically derivative)
FunctionBlock(Name *name, const ArgumentVector ¶meters, Unit *unit, StatementBlock *statement_block)
std::shared_ptr< ModToken > token
token with location information
LinEquation(Expression *lhs, Expression *rhs)
void set_op(UnaryOperator &&op)
Setter for member variable UnaryExpression::op (rvalue reference)
virtual void visit_constructor_block(const ast::ConstructorBlock &node)=0
visit node of type ast::ConstructorBlock
void set_parent_in_children()
Set this object as parent for all the children.
std::shared_ptr< ModToken > token
token with location information
Represent LONGITUDINAL_DIFFUSION statement in NMODL.
std::shared_ptr< Expression > volume
The volume of the compartment.
std::shared_ptr< Identifier > name
Name of the argument.
void set_name(std::shared_ptr< Name > &&name)
Setter for member variable RangeVar::name (rvalue reference)
virtual bool is_compartment() const noexcept
Check if the ast node is an instance of ast::Compartment.
virtual bool is_factor_def() const noexcept
Check if the ast node is an instance of ast::FactorDef.
std::shared_ptr< Double > value
TODO.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
void visit_children(visitor::Visitor &v) override
visit children i.e.
virtual void visit_function_table_block(ast::FunctionTableBlock &node)=0
visit node of type ast::FunctionTableBlock
SolveBlock(Name *block_name, Name *method, Name *steadystate)
virtual void visit_lon_diffuse(const ast::LonDiffuse &node)=0
visit node of type ast::LonDiffuse
void set_parent_in_children()
Set this object as parent for all the children.
void reset_assigned_definition(AssignedDefinitionVector::const_iterator position, AssignedDefinition *n)
Reset member to definitions.
virtual void visit_solution_expression(const ast::SolutionExpression &node)=0
visit node of type ast::SolutionExpression
Represents a STATE block in the NMODL.
void set_expression(std::shared_ptr< BinaryExpression > &&expression)
Setter for member variable DiffEqExpression::expression (rvalue reference)
void set_macro(std::shared_ptr< Name > &¯o)
Setter for member variable Integer::macro (rvalue reference)
void set_parent_in_children()
Set this object as parent for all the children.
void set_name(std::shared_ptr< String > &&name)
Setter for member variable Unit::name (rvalue reference)
std::shared_ptr< ModToken > token
token with location information
std::string get_node_name() const override
Return name of the node.
virtual void visit_local_list_statement(ast::LocalListStatement &node)=0
visit node of type ast::LocalListStatement
std::shared_ptr< Number > start
TODO.
virtual void visit_watch(ast::Watch &node)=0
visit node of type ast::Watch
void visit_children(visitor::Visitor &v) override
visit children i.e.
virtual bool is_reaction_operator() const noexcept
Check if the ast node is an instance of ast::ReactionOperator.
void visit_children(visitor::Visitor &v) override
visit children i.e.
virtual void set_symbol_table(symtab::SymbolTable *symtab)
Set symbol table for the AST node.
void set_statement_block(std::shared_ptr< StatementBlock > &&statement_block)
Setter for member variable ElseStatement::statement_block (rvalue reference)
void set_valence(std::shared_ptr< Valence > &&valence)
Setter for member variable Useion::valence (rvalue reference)
void set_elseifs(ElseIfStatementVector &&elseifs)
Setter for member variable IfStatement::elseifs (rvalue reference)
void set_value(UnaryOp value)
Setter for member variable UnaryOperator::value.
void set_parent_in_children()
Set this object as parent for all the children.
std::shared_ptr< Unit > unit1
TODO.
std::string get_node_name() const override
Return name of the node.
virtual bool is_lin_equation() const noexcept
Check if the ast node is an instance of ast::LinEquation.
virtual void visit_unary_expression(const ast::UnaryExpression &node)=0
visit node of type ast::UnaryExpression
void set_unit(std::shared_ptr< Unit > &&unit)
Setter for member variable FunctionBlock::unit (rvalue reference)
virtual void visit_binary_expression(const ast::BinaryExpression &node)=0
visit node of type ast::BinaryExpression
virtual bool is_define() const noexcept
Check if the ast node is an instance of ast::Define.
void set_parent_in_children()
Set this object as parent for all the children.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
virtual bool is_statement_block() const noexcept
Check if the ast node is an instance of ast::StatementBlock.
UnitState(UnitStateType value)
void visit_children(visitor::Visitor &v) override
visit children i.e.
virtual void visit_model(ast::Model &node)=0
visit node of type ast::Model
void visit_children(visitor::Visitor &v) override
visit children i.e.
std::shared_ptr< ModToken > token
token with location information
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
std::shared_ptr< Expression > length
length of an array or index position
Represents the coreneuron nrn_state callback function.
ElectrodeCurVarVector currents
Vector of electrode current variables.
Argument(Identifier *name, Unit *unit)
void set_parent_in_children()
Set this object as parent for all the children.
virtual bool is_non_lin_equation() const noexcept
Check if the ast node is an instance of ast::NonLinEquation.
virtual bool is_indexed_name() const noexcept
Check if the ast node is an instance of ast::IndexedName.
Represents block encapsulating list of statements.
virtual std::shared_ptr< Ast > get_shared_ptr()
get std::shared_ptr from this pointer of the AST node
void set_statement_block(std::shared_ptr< StatementBlock > &&statement_block)
Setter for member variable LinearBlock::statement_block (rvalue reference)
std::shared_ptr< ModToken > token
token with location information
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
void visit_children(visitor::Visitor &v) override
visit children i.e.
virtual void visit_float(const ast::Float &node)=0
visit node of type ast::Float
WatchVector statements
Vector of watch statements.
std::shared_ptr< Integer > length
Length in case of array.
std::shared_ptr< ModToken > token
token with location information
std::shared_ptr< ModToken > token
token with location information
void set_react(std::shared_ptr< Expression > &&react)
Setter for member variable Conserve::react (rvalue reference)
void set_type(std::shared_ptr< Name > &&type)
Setter for member variable Valence::type (rvalue reference)
virtual void visit_range_var(ast::RangeVar &node)=0
visit node of type ast::RangeVar
ConstantStatementVector statements
Vector of constant statements.
std::vector< std::shared_ptr< Expression > > ExpressionVector
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
virtual bool is_unit_state() const noexcept
Check if the ast node is an instance of ast::UnitState.
virtual void visit_nonspecific_cur_var(const ast::NonspecificCurVar &node)=0
visit node of type ast::NonspecificCurVar
Represents CURIE information in NMODL.
This construct is deprecated and no longer supported in the NMODL.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
Represents an argument to functions and procedures.
std::shared_ptr< Expression > expression
TODO.
void set_filename(std::shared_ptr< String > &&filename)
Setter for member variable Include::filename (rvalue reference)
virtual void visit_ontology_statement(const ast::OntologyStatement &node)=0
visit node of type ast::OntologyStatement
std::shared_ptr< StatementBlock > statement_block
Block with statements vector.
std::vector< std::shared_ptr< AssignedDefinition > > AssignedDefinitionVector
std::vector< std::shared_ptr< NonspecificCurVar > > NonspecificCurVarVector
void set_name(std::shared_ptr< Name > &&name)
Setter for member variable FunctionCall::name (rvalue reference)
std::shared_ptr< ModToken > token
token with location information
std::shared_ptr< Name > name
TODO.
void set_parent_in_children()
Set this object as parent for all the children.
NetReceiveBlock(const ArgumentVector ¶meters, StatementBlock *statement_block)
void set_parent_in_children()
Set this object as parent for all the children.
virtual bool is_include() const noexcept
Check if the ast node is an instance of ast::Include.
void set_statement_block(std::shared_ptr< StatementBlock > &&statement_block)
Setter for member variable DestructorBlock::statement_block (rvalue reference)
void set_parent_in_children()
Set this object as parent for all the children.
std::shared_ptr< Expression > expression
TODO.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
std::shared_ptr< String > name
TODO.
BinaryExpression(Expression *lhs, const BinaryOperator &op, Expression *rhs)
std::shared_ptr< ModToken > token
token with location information
void visit_children(visitor::Visitor &v) override
visit children i.e.
void set_reaction2(std::shared_ptr< Expression > &&reaction2)
Setter for member variable ReactionStatement::reaction2 (rvalue reference)
std::string get_node_name() const override
Return name of the node.
void reset_node(NodeVector::const_iterator position, Node *n)
Reset member to blocks.
std::shared_ptr< Name > name
TODO.
void reset_statement(StatementVector::const_iterator position, Statement *n)
Reset member to statements.
ReactionOperator()=default
virtual bool is_param_assign() const noexcept
Check if the ast node is an instance of ast::ParamAssign.
void set_parent_in_children()
Set this object as parent for all the children.
Represents LINEAR block in the NMODL.
UnaryExpression(const UnaryOperator &op, Expression *expression)
std::shared_ptr< ModToken > token
token with location information
std::shared_ptr< ModToken > token
token with location information
void visit_children(visitor::Visitor &v) override
visit children i.e.
virtual void visit_pointer_var(ast::PointerVar &node)=0
visit node of type ast::PointerVar
virtual void visit_protect_statement(ast::ProtectStatement &node)=0
visit node of type ast::ProtectStatement
std::shared_ptr< ModToken > token
token with location information
virtual void visit_define(const ast::Define &node)=0
visit node of type ast::Define
void set_rhs(std::shared_ptr< Expression > &&rhs)
Setter for member variable NonLinEquation::rhs (rvalue reference)
virtual bool is_node() const noexcept
Check if the ast node is an instance of ast::Node.
Represent a callback to NEURON's derivimplicit solver.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
void visit_children(visitor::Visitor &v) override
visit children i.e.
std::shared_ptr< StatementBlock > statement_block
TODO.
void set_ion(std::shared_ptr< Name > &&ion)
Setter for member variable ConductanceHint::ion (rvalue reference)
std::shared_ptr< ModToken > token
token with location information
std::shared_ptr< Number > to
TODO.
Ast * parent
Generic pointer to the parent.
std::shared_ptr< Name > name
TODO.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
virtual void visit_string(const ast::String &node)=0
visit node of type ast::String
virtual bool is_lon_diffuse() const noexcept
Check if the ast node is an instance of ast::LonDiffuse.
void set_parent_in_children()
Set this object as parent for all the children.
virtual bool is_ba_block() const noexcept
Check if the ast node is an instance of ast::BABlock.
void set_initialize_block(std::shared_ptr< StatementBlock > &&initialize_block)
Setter for member variable EigenLinearSolverBlock::initialize_block (rvalue reference)
FunctionTableBlock(Name *name, const ArgumentVector ¶meters, Unit *unit)
std::shared_ptr< Limits > limit
TODO.
void set_definitions(AssignedDefinitionVector &&definitions)
Setter for member variable AssignedBlock::definitions (rvalue reference)
void emplace_back_watch(Watch *n)
Add member to statements by raw pointer.
void set_parent_in_children()
Set this object as parent for all the children.
void set_parent_in_children()
Set this object as parent for all the children.
std::shared_ptr< ModToken > token
token with location information
std::shared_ptr< Double > abstol
TODO.
virtual bool is_number_range() const noexcept
Check if the ast node is an instance of ast::NumberRange.
void visit_children(visitor::Visitor &v) override
visit children i.e.
void visit_children(visitor::Visitor &v) override
visit children i.e.
std::string get_node_name() const override
Return name of the node.
virtual void visit_paren_expression(ast::ParenExpression &node)=0
visit node of type ast::ParenExpression
virtual bool is_unary_expression() const noexcept
Check if the ast node is an instance of ast::UnaryExpression.
void set_parent_in_children()
Set this object as parent for all the children.
std::shared_ptr< ModToken > token
token with location information
virtual bool is_unit_def() const noexcept
Check if the ast node is an instance of ast::UnitDef.
void visit_children(visitor::Visitor &v) override
visit children i.e.
Represent statement in CONSTANT block of NMODL.
std::string get_node_name() const override
Return name of the node.
void set_statements(ParamAssignVector &&statements)
Setter for member variable ParamBlock::statements (rvalue reference)
Operator used in ast::BinaryExpression.
UnitDef(Unit *unit1, Unit *unit2)
void visit_children(visitor::Visitor &v) override
visit children i.e.
std::shared_ptr< Expression > rate
Diffusion coefficient/rate.
Type to represent different block types for before/after block.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
std::string get_node_name() const override
Return name of the node.
void set_parent_in_children()
Set this object as parent for all the children.
virtual void visit_kinetic_block(ast::KineticBlock &node)=0
visit node of type ast::KineticBlock
Represents NONLINEAR block in the NMODL.
void set_parent_in_children()
Set this object as parent for all the children.
void set_name(std::shared_ptr< Name > &&name)
Setter for member variable ConstantVar::name (rvalue reference)
void set_parent_in_children()
Set this object as parent for all the children.
std::shared_ptr< BABlockType > type
Type of NMODL block.
std::shared_ptr< Name > name
Name of the function table block.
std::shared_ptr< Unit > unit
Unit for the variable.
void set_parent_in_children()
Set this object as parent for all the children.
std::shared_ptr< ModToken > token
token with location information
std::shared_ptr< ModToken > token
token with location information
void visit_children(visitor::Visitor &v) override
visit children i.e.
std::vector< std::shared_ptr< Watch > > WatchVector
void set_parent_in_children()
Set this object as parent for all the children.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
void set_constant(std::shared_ptr< ConstantVar > &&constant)
Setter for member variable ConstantStatement::constant (rvalue reference)
void set_parent_in_children()
Set this object as parent for all the children.
virtual void visit_boolean(const ast::Boolean &node)=0
visit node of type ast::Boolean
virtual void visit_kinetic_block(const ast::KineticBlock &node)=0
visit node of type ast::KineticBlock
std::shared_ptr< ModToken > token
token with location information
void visit_children(visitor::Visitor &v) override
visit children i.e.
Represent solution of a block in the AST.
void set_value(std::shared_ptr< Integer > &&value)
Setter for member variable ReactVarName::value (rvalue reference)
void set_name(std::shared_ptr< Name > &&name)
Setter for member variable FunctionBlock::name (rvalue reference)
NodeVector::const_iterator insert_node(NodeVector::const_iterator position, const std::shared_ptr< Node > &n)
Insert member to blocks.
virtual void visit_constructor_block(ast::ConstructorBlock &node)=0
visit node of type ast::ConstructorBlock
virtual bool is_linear_block() const noexcept
Check if the ast node is an instance of ast::LinearBlock.
virtual void visit_reaction_statement(ast::ReactionStatement &node)=0
visit node of type ast::ReactionStatement
void visit_children(visitor::Visitor &v) override
visit children i.e.
virtual void visit_discrete_block(const ast::DiscreteBlock &node)=0
visit node of type ast::DiscreteBlock
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
virtual void visit_procedure_block(ast::ProcedureBlock &node)=0
visit node of type ast::ProcedureBlock
void visit_children(visitor::Visitor &v) override
visit children i.e.
void visit_children(visitor::Visitor &v) override
visit children i.e.
virtual bool is_param_block() const noexcept
Check if the ast node is an instance of ast::ParamBlock.
std::shared_ptr< StatementBlock > statement_block
Block with statements vector.
virtual void visit_reaction_operator(const ast::ReactionOperator &node)=0
visit node of type ast::ReactionOperator
std::shared_ptr< VarName > name
TODO.
void set_name(std::shared_ptr< Name > &&name)
Setter for member variable FromStatement::name (rvalue reference)
void set_value(std::shared_ptr< Double > &&value)
Setter for member variable Valence::value (rvalue reference)
void visit_children(visitor::Visitor &v) override
visit children i.e.
std::shared_ptr< ModToken > token
token with location information
void set_parent_in_children()
Set this object as parent for all the children.
One equation in a system of equations tha collectively form a LINEAR block.
std::string value
Value of double.
void set_statement_block(std::shared_ptr< StatementBlock > &&statement_block)
Setter for member variable WhileStatement::statement_block (rvalue reference)
virtual void visit_unary_operator(ast::UnaryOperator &node)=0
visit node of type ast::UnaryOperator
EigenNewtonSolverBlock(Integer *n_state_vars, StatementBlock *variable_block, StatementBlock *initialize_block, StatementBlock *setup_x_block, StatementBlock *functor_block, StatementBlock *update_states_block, StatementBlock *finalize_block)
std::shared_ptr< Name > type
TODO.
virtual bool is_prime_name() const noexcept
Check if the ast node is an instance of ast::PrimeName.
std::shared_ptr< Name > name
Name of the non-linear block.
virtual void visit_unit(ast::Unit &node)=0
visit node of type ast::Unit
std::string get_node_name() const override
Return name of the node.
virtual void visit_ba_block_type(ast::BABlockType &node)=0
visit node of type ast::BABlockType
void set_parent_in_children()
Set this object as parent for all the children.
void set_condition(std::shared_ptr< Expression > &&condition)
Setter for member variable ElseIfStatement::condition (rvalue reference)
virtual bool is_solution_expression() const noexcept
Check if the ast node is an instance of ast::SolutionExpression.
virtual void visit_before_block(ast::BeforeBlock &node)=0
visit node of type ast::BeforeBlock
LocalVar(Identifier *name)
virtual bool is_limits() const noexcept
Check if the ast node is an instance of ast::Limits.
virtual bool is_eigen_linear_solver_block() const noexcept
Check if the ast node is an instance of ast::EigenLinearSolverBlock.
Represents a INDEPENDENT block in the NMODL.
ArgumentVector parameters
Vector of the parameters.
virtual bool is_program() const noexcept
Check if the ast node is an instance of ast::Program.
virtual void visit_block_comment(const ast::BlockComment &node)=0
visit node of type ast::BlockComment
void set_parent_in_children()
Set this object as parent for all the children.
void set_node_to_solve(std::shared_ptr< Expression > &&node_to_solve)
Setter for member variable SolutionExpression::node_to_solve (rvalue reference)
virtual void visit_double_unit(ast::DoubleUnit &node)=0
visit node of type ast::DoubleUnit
virtual bool is_cvode_block() const noexcept
Check if the ast node is an instance of ast::CvodeBlock.
void set_parent_in_children()
Set this object as parent for all the children.
void set_name(std::shared_ptr< Name > &&name)
Setter for member variable Define::name (rvalue reference)
virtual void visit_constant_statement(ast::ConstantStatement &node)=0
visit node of type ast::ConstantStatement
void set_parent_in_children()
Set this object as parent for all the children.
void set_value(int value)
Setter for member variable Boolean::value.
virtual bool is_independent_block() const noexcept
Check if the ast node is an instance of ast::IndependentBlock.
ExpressionVector definitions
Vector of unit statements.
void visit_children(visitor::Visitor &v) override
visit children i.e.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
virtual bool is_solve_block() const noexcept
Check if the ast node is an instance of ast::SolveBlock.
std::shared_ptr< ModToken > token
token with location information
UnitBlock(const ExpressionVector &definitions)
virtual void visit_unit_def(const ast::UnitDef &node)=0
visit node of type ast::UnitDef
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
std::shared_ptr< Expression > index
index value in case of array
OntologyStatement(String *ontology_id)
Represents BBCOREPOINTER statement in NMODL.
void set_parent_in_children()
Set this object as parent for all the children.
std::shared_ptr< ModToken > token
token with location information
Represents NONSPECIFIC_CURRENT variables statement in NMODL.
std::shared_ptr< ConstantVar > constant
single constant variable
std::shared_ptr< Unit > unit1
TODO.
virtual bool is_identifier() const noexcept
Check if the ast node is an instance of ast::Identifier.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
void visit_children(visitor::Visitor &v) override
visit children i.e.
ParamAssignVector statements
Vector of parameters.
ElectrodeCurrent(const ElectrodeCurVarVector ¤ts)
void set_parent_in_children()
Set this object as parent for all the children.
void set_parent_in_children()
Set this object as parent for all the children.
BreakpointBlock(StatementBlock *statement_block)
virtual void visit_useion(const ast::Useion &node)=0
visit node of type ast::Useion
virtual bool is_line_comment() const noexcept
Check if the ast node is an instance of ast::LineComment.
void set_parent_in_children()
Set this object as parent for all the children.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
std::shared_ptr< ModToken > token
token with location information
virtual void visit_linear_block(ast::LinearBlock &node)=0
visit node of type ast::LinearBlock
void visit_children(visitor::Visitor &v) override
visit children i.e.
virtual bool is_while_statement() const noexcept
Check if the ast node is an instance of ast::WhileStatement.
void set_parent_in_children()
Set this object as parent for all the children.
std::shared_ptr< Name > name
Name of the derivative block.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
std::shared_ptr< String > statement
C code as a string.
virtual std::shared_ptr< StatementBlock > get_statement_block() const
Return associated statement block for the AST node.
void set_parent_in_children()
Set this object as parent for all the children.
std::shared_ptr< ModToken > token
token with location information
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
std::shared_ptr< Name > name
TODO.
virtual bool is_else_statement() const noexcept
Check if the ast node is an instance of ast::ElseStatement.
virtual void visit_update_dt(const ast::UpdateDt &node)=0
visit node of type ast::UpdateDt
std::shared_ptr< Identifier > name
Name of variable.
void set_steadystate(std::shared_ptr< Name > &&steadystate)
Setter for member variable SolveBlock::steadystate (rvalue reference)
void set_parent_in_children()
Set this object as parent for all the children.
std::shared_ptr< ModToken > token
token with location information
Represents top level AST node for whole NMODL input.
virtual void visit_prime_name(const ast::PrimeName &node)=0
visit node of type ast::PrimeName
void visit_children(visitor::Visitor &v) override
visit children i.e.
void set_name(std::shared_ptr< Name > &&name)
Setter for member variable FactorDef::name (rvalue reference)
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
std::shared_ptr< Name > name
Name of the kinetic block.
void set_parent_in_children()
Set this object as parent for all the children.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
std::shared_ptr< ModToken > token
token with location information
virtual void visit_update_dt(ast::UpdateDt &node)=0
visit node of type ast::UpdateDt
std::shared_ptr< StatementBlock > statement_block
Block with statements vector.
void visit_children(visitor::Visitor &v) override
visit children i.e.
virtual bool is_nonspecific_cur_var() const noexcept
Check if the ast node is an instance of ast::NonspecificCurVar.
InitialBlock(StatementBlock *statement_block)
Base class for all numbers.
Represents a AFTER block in NMODL.
UnitStateType
enum type used for UNIT_ON or UNIT_OFF state
std::shared_ptr< ModToken > token
token with location information
virtual void visit_longitudinal_diffusion_block(ast::LongitudinalDiffusionBlock &node)=0
visit node of type ast::LongitudinalDiffusionBlock
void set_parent_in_children()
Set this object as parent for all the children.
virtual bool is_derivimplicit_callback() const noexcept
Check if the ast node is an instance of ast::DerivimplicitCallback.
Base class for all expressions in the NMODL.
virtual bool is_before_block() const noexcept
Check if the ast node is an instance of ast::BeforeBlock.
Represents POINTER statement in NMODL.
void set_statement_block(std::shared_ptr< StatementBlock > &&statement_block)
Setter for member variable DerivativeBlock::statement_block (rvalue reference)
NonLinearBlock(Name *name, const NameVector &solvefor, StatementBlock *statement_block)
void set_statement_block(std::shared_ptr< StatementBlock > &&statement_block)
Setter for member variable NeuronBlock::statement_block (rvalue reference)
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
std::shared_ptr< StatementBlock > compartment_statements
All (required) COMPARTMENT statements in the KINETIC block.
virtual void visit_state_block(const ast::StateBlock &node)=0
visit node of type ast::StateBlock
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
void visit_children(visitor::Visitor &v) override
visit children i.e.
virtual void visit_nonspecific(ast::Nonspecific &node)=0
visit node of type ast::Nonspecific
std::shared_ptr< StatementBlock > non_stiff_block
Block with statements of the form Dvar = f(var), used for updating non-stiff systems.
virtual void visit_from_statement(const ast::FromStatement &node)=0
visit node of type ast::FromStatement
DiscreteBlock(Name *name, StatementBlock *statement_block)
void set_parent_in_children()
Set this object as parent for all the children.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
void set_parent_in_children()
Set this object as parent for all the children.
void set_parent_in_children()
Set this object as parent for all the children.
void visit_children(visitor::Visitor &v) override
visit children i.e.
void visit_children(visitor::Visitor &v) override
visit children i.e.
std::shared_ptr< Expression > condition
TODO.
void visit_children(visitor::Visitor &v) override
visit children i.e.
void set_parent_in_children()
Set this object as parent for all the children.
void set_variables(RangeVarVector &&variables)
Setter for member variable Range::variables (rvalue reference)
void set_statement_block(std::shared_ptr< StatementBlock > &&statement_block)
Setter for member variable NetReceiveBlock::statement_block (rvalue reference)
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
Represents ELECTRODE_CURRENT variables statement in NMODL.
Represent NEURON block in the mod file.
std::shared_ptr< Expression > increment
TODO.
Range(const RangeVarVector &variables)
void set_variables(BbcorePointerVarVector &&variables)
Setter for member variable BbcorePointer::variables (rvalue reference)
std::shared_ptr< Name > macro
if integer is a macro then it's name
DestructorBlock(StatementBlock *statement_block)
std::shared_ptr< Name > name
Name of the variable.
std::string get_node_name() const override
Return name of the node.
void set_parent_in_children()
Set this object as parent for all the children.
std::string get_node_name() const override
Return name of the node.
std::shared_ptr< StatementBlock > update_states_block
update back states from X
std::shared_ptr< ModToken > token
token with location information
virtual void visit_local_list_statement(const ast::LocalListStatement &node)=0
visit node of type ast::LocalListStatement
void set_solvefor(NameVector &&solvefor)
Setter for member variable KineticBlock::solvefor (rvalue reference)
AssignedDefinition(Identifier *name, Integer *length, Number *from, Number *to, Number *start, Unit *unit, Double *abstol)
std::shared_ptr< Name > name
Name of the channel.
virtual void visit_initial_block(ast::InitialBlock &node)=0
visit node of type ast::InitialBlock
virtual bool is_electrode_current() const noexcept
Check if the ast node is an instance of ast::ElectrodeCurrent.
virtual void visit_integer(ast::Integer &node)=0
visit node of type ast::Integer
virtual void visit_indexed_name(const ast::IndexedName &node)=0
visit node of type ast::IndexedName
std::shared_ptr< ModToken > token
token with location information
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
std::shared_ptr< StatementBlock > setup_x_block
update X from states
void emplace_back_node(Node *n)
Add member to blocks by raw pointer.
virtual void visit_range_var(const ast::RangeVar &node)=0
visit node of type ast::RangeVar
std::shared_ptr< ModToken > token
token with location information
std::shared_ptr< Double > value
TODO.
void set_value(BinaryOp value)
Setter for member variable BinaryOperator::value.
void set_parent_in_children()
Set this object as parent for all the children.
virtual bool is_binary_operator() const noexcept
Check if the ast node is an instance of ast::BinaryOperator.
virtual bool is_constant_block() const noexcept
Check if the ast node is an instance of ast::ConstantBlock.
void visit_children(visitor::Visitor &v) override
visit children i.e.
void set_compartment_statements(std::shared_ptr< StatementBlock > &&compartment_statements)
Setter for member variable LongitudinalDiffusionBlock::compartment_statements (rvalue reference)
void set_parent_in_children()
Set this object as parent for all the children.
void set_statement_block(std::shared_ptr< StatementBlock > &&statement_block)
Setter for member variable NonLinearBlock::statement_block (rvalue reference)
virtual void visit_thread_safe(ast::ThreadSafe &node)=0
visit node of type ast::ThreadSafe
virtual bool is_nonspecific() const noexcept
Check if the ast node is an instance of ast::Nonspecific.
NameVector solvefor
Name of the integration method.
Represents binary expression in the NMODL.
void set_parent_in_children()
Set this object as parent for all the children.
std::shared_ptr< Expression > lhs
Left-hand-side of the equation.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
Represents a PARAMETER block in the NMODL.
std::vector< std::shared_ptr< WriteIonVar > > WriteIonVarVector
virtual void visit_random_var_list(ast::RandomVarList &node)=0
visit node of type ast::RandomVarList
std::string get_node_name() const override
Return name of the node.
void set_variables(PointerVarVector &&variables)
Setter for member variable Pointer::variables (rvalue reference)
void set_name(std::shared_ptr< VarName > &&name)
Setter for member variable ReactVarName::name (rvalue reference)
void reset_pointer_var(PointerVarVector::const_iterator position, PointerVar *n)
Reset member to variables.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
void set_currents(NonspecificCurVarVector &¤ts)
Setter for member variable Nonspecific::currents (rvalue reference)
std::shared_ptr< Expression > react
TODO.
virtual void visit_function_call(ast::FunctionCall &node)=0
visit node of type ast::FunctionCall
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
Compartment(Name *index_name, Expression *volume, const NameVector &species)
std::shared_ptr< Expression > from
from value
std::shared_ptr< Integer > n_state_vars
number of state vars used in solve
void set_blocks(NodeVector &&blocks)
Setter for member variable Program::blocks (rvalue reference)
void set_name(std::shared_ptr< Name > &&name)
Setter for member variable Useion::name (rvalue reference)
virtual void visit_global(ast::Global &node)=0
visit node of type ast::Global
virtual void visit_ba_block(const ast::BABlock &node)=0
visit node of type ast::BABlock
virtual void visit_number(const ast::Number &node)=0
visit node of type ast::Number
virtual bool is_after_block() const noexcept
Check if the ast node is an instance of ast::AfterBlock.
void set_statement_block(std::shared_ptr< StatementBlock > &&statement_block)
Setter for member variable InitialBlock::statement_block (rvalue reference)
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
NodeVector blocks
Vector of top level blocks in the mod file.
void set_variables(GlobalVarVector &&variables)
Setter for member variable Global::variables (rvalue reference)
void set_parameters(ArgumentVector &¶meters)
Setter for member variable FunctionTableBlock::parameters (rvalue reference)
std::shared_ptr< StatementBlock > statement_block
Block with statements vector.
void set_parent_in_children()
Set this object as parent for all the children.
std::shared_ptr< Name > name
Variable name.
void set_title(std::shared_ptr< String > &&title)
Setter for member variable Model::title (rvalue reference)
void set_name(std::shared_ptr< Name > &&name)
Setter for member variable GlobalVar::name (rvalue reference)
void set_reaction1(std::shared_ptr< Expression > &&reaction1)
Setter for member variable ReactionStatement::reaction1 (rvalue reference)
void emplace_back_global_var(GlobalVar *n)
Add member to variables by raw pointer.
std::string get_node_name() const override
Return name of the node.
Valence(Name *type, Double *value)
BbcorePointerVar(Name *name)
virtual bool is_for_netcon() const noexcept
Check if the ast node is an instance of ast::ForNetcon.
WatchVector::const_iterator erase_watch(WatchVector::const_iterator first)
Erase member to statements.
void set_parent_in_children()
Set this object as parent for all the children.
virtual bool is_float() const noexcept
Check if the ast node is an instance of ast::Float.
std::vector< std::shared_ptr< ExternVar > > ExternVarVector
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
ParamAssign(Identifier *name, Number *value, Unit *unit, Limits *limit)
void visit_children(visitor::Visitor &v) override
visit children i.e.
void set_type(std::shared_ptr< Name > &&type)
Setter for member variable Suffix::type (rvalue reference)
void set_value(std::shared_ptr< Number > &&value)
Setter for member variable ConstantVar::value (rvalue reference)
IndexedName(Identifier *name, Expression *length)
virtual void visit_wrapped_expression(const ast::WrappedExpression &node)=0
visit node of type ast::WrappedExpression
WhileStatement(Expression *condition, StatementBlock *statement_block)
virtual void visit_else_statement(const ast::ElseStatement &node)=0
visit node of type ast::ElseStatement
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
void set_expression1(std::shared_ptr< Expression > &&expression1)
Setter for member variable ReactionStatement::expression1 (rvalue reference)
void set_parent_in_children()
Set this object as parent for all the children.
std::shared_ptr< ModToken > token
token with location information
std::shared_ptr< String > value
Value of name.
Represent token returned by scanner.
void set_value(std::shared_ptr< Double > &&value)
Setter for member variable FactorDef::value (rvalue reference)
void set_parent_in_children()
Set this object as parent for all the children.
virtual bool is_derivative_block() const noexcept
Check if the ast node is an instance of ast::DerivativeBlock.
virtual bool is_boolean() const noexcept
Check if the ast node is an instance of ast::Boolean.
virtual Ast * get_parent() const
Parent getter.
std::shared_ptr< ModToken > token
token with location information
std::string get_node_name() const override
Return name of the node.
GlobalVarVector::const_iterator insert_global_var(GlobalVarVector::const_iterator position, const std::shared_ptr< GlobalVar > &n)
Insert member to variables.
virtual bool is_unary_operator() const noexcept
Check if the ast node is an instance of ast::UnaryOperator.
virtual std::string get_node_name() const
Return name of of the node.
void set_order(std::shared_ptr< Integer > &&order)
Setter for member variable PrimeName::order (rvalue reference)
virtual bool is_range_var() const noexcept
Check if the ast node is an instance of ast::RangeVar.
AssignedDefinitionVector definitions
Vector of assigned variables.
void visit_children(visitor::Visitor &v) override
visit children i.e.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
virtual void visit_range(ast::Range &node)=0
visit node of type ast::Range
void set_parent_in_children()
Set this object as parent for all the children.
void set_byname(std::shared_ptr< Name > &&byname)
Setter for member variable LagStatement::byname (rvalue reference)
void set_name(std::shared_ptr< Identifier > &&name)
Setter for member variable LocalVar::name (rvalue reference)
virtual void visit_if_statement(const ast::IfStatement &node)=0
visit node of type ast::IfStatement
std::shared_ptr< Name > name
TODO.
void visit_children(visitor::Visitor &v) override
visit children i.e.
std::shared_ptr< ModToken > token
token with location information
Auto generated AST classes declaration.
Wrap any other expression type.
virtual void visit_longitudinal_diffusion_block(const ast::LongitudinalDiffusionBlock &node)=0
visit node of type ast::LongitudinalDiffusionBlock
void set_unit(std::shared_ptr< Unit > &&unit)
Setter for member variable ParamAssign::unit (rvalue reference)
ElseIfStatement(Expression *condition, StatementBlock *statement_block)
virtual void visit_lon_diffuse(ast::LonDiffuse &node)=0
visit node of type ast::LonDiffuse
void set_min(std::shared_ptr< Number > &&min)
Setter for member variable Limits::min (rvalue reference)
virtual void visit_nrn_state_block(const ast::NrnStateBlock &node)=0
visit node of type ast::NrnStateBlock
void set_parent_in_children()
Set this object as parent for all the children.
void visit_children(visitor::Visitor &v) override
visit children i.e.
virtual void visit_extern_var(const ast::ExternVar &node)=0
visit node of type ast::ExternVar
virtual bool is_useion() const noexcept
Check if the ast node is an instance of ast::Useion.
std::string get_node_name() const override
Return name of the node.
void set_expression(std::shared_ptr< Expression > &&expression)
Setter for member variable ExpressionStatement::expression (rvalue reference)
std::shared_ptr< ModToken > token
token with location information
void set_from(std::shared_ptr< Expression > &&from)
Setter for member variable FromStatement::from (rvalue reference)
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
void set_parent_in_children()
Set this object as parent for all the children.
void set_length(std::shared_ptr< Expression > &&length)
Setter for member variable IndexedName::length (rvalue reference)
std::shared_ptr< ModToken > token
token with location information
std::shared_ptr< Name > name
TODO.
virtual void visit_constant_var(const ast::ConstantVar &node)=0
visit node of type ast::ConstantVar
void set_name(std::shared_ptr< Name > &&name)
Setter for member variable DerivativeBlock::name (rvalue reference)
virtual void visit_external(ast::External &node)=0
visit node of type ast::External
Suffix(Name *type, Name *name)
std::shared_ptr< ModToken > token
token with location information
void set_parent_in_children()
Set this object as parent for all the children.
void visit_children(visitor::Visitor &v) override
visit children i.e.
std::shared_ptr< ModToken > token
token with location information
ExpressionStatement(Expression *expression)
void set_value(int value)
Setter for member variable Integer::value.
virtual bool is_read_ion_var() const noexcept
Check if the ast node is an instance of ast::ReadIonVar.
void set_unit1(std::shared_ptr< Unit > &&unit1)
Setter for member variable FactorDef::unit1 (rvalue reference)
BbcorePointer(const BbcorePointerVarVector &variables)
std::string get_node_name() const override
Return name of the node.
void visit_children(visitor::Visitor &v) override
visit children i.e.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
virtual void visit_lin_equation(ast::LinEquation &node)=0
visit node of type ast::LinEquation
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
std::shared_ptr< ModToken > token
token with location information
void set_max(std::shared_ptr< Number > &&max)
Setter for member variable Limits::max (rvalue reference)
void set_max(std::shared_ptr< Number > &&max)
Setter for member variable NumberRange::max (rvalue reference)
std::shared_ptr< ModToken > token
token with location information
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
void visit_children(visitor::Visitor &v) override
visit children i.e.
void visit_children(visitor::Visitor &v) override
visit children i.e.
virtual void visit_var_name(ast::VarName &node)=0
visit node of type ast::VarName
void visit_children(visitor::Visitor &v) override
visit children i.e.
std::shared_ptr< Name > byname
Name of the variable (TODO)
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
void set_name(std::shared_ptr< Name > &&name)
Setter for member variable PointerVar::name (rvalue reference)
void set_parameters(ArgumentVector &¶meters)
Setter for member variable FunctionBlock::parameters (rvalue reference)
virtual bool is_constructor_block() const noexcept
Check if the ast node is an instance of ast::ConstructorBlock.
void reset_watch(WatchVector::const_iterator position, Watch *n)
Reset member to statements.
virtual void visit_cvode_block(const ast::CvodeBlock &node)=0
visit node of type ast::CvodeBlock
void set_expression(std::shared_ptr< Expression > &&expression)
Setter for member variable ParenExpression::expression (rvalue reference)
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
void set_parent_in_children()
Set this object as parent for all the children.
void set_species(NameVector &&species)
Setter for member variable Compartment::species (rvalue reference)