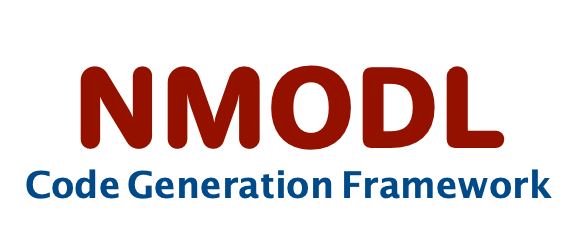 |
User Guide
|
Go to the documentation of this file.
46 std::shared_ptr<Expression>
from;
48 std::shared_ptr<Expression>
to;
50 std::shared_ptr<Integer>
with;
52 std::shared_ptr<ModToken>
token;
116 return "TableStatement";
138 return std::static_pointer_cast<TableStatement>(shared_from_this());
145 return std::static_pointer_cast<const TableStatement>(shared_from_this());
184 std::shared_ptr<Expression>
get_from() const noexcept {
193 std::shared_ptr<Expression>
get_to() const noexcept {
202 std::shared_ptr<Integer>
get_with() const noexcept {
250 void set_to(std::shared_ptr<Expression>&&
to);
255 void set_to(
const std::shared_ptr<Expression>&
to);
const NameVector & get_depend_vars() const noexcept
Getter for member variable TableStatement::depend_vars.
Abstract base class for all constant visitors implementation.
void set_table_vars(NameVector &&table_vars)
Setter for member variable TableStatement::table_vars (rvalue reference)
NameVector table_vars
Variables in the table.
std::shared_ptr< Integer > with
an increment factor
std::shared_ptr< Integer > get_with() const noexcept
Getter for member variable TableStatement::with.
void set_to(std::shared_ptr< Expression > &&to)
Setter for member variable TableStatement::to (rvalue reference)
void set_parent_in_children()
Set this object as parent for all the children.
THIS FILE IS GENERATED AT BUILD TIME AND SHALL NOT BE EDITED.
Auto generated AST classes declaration.
std::shared_ptr< ModToken > token
token with location information
Represents TABLE statement in NMODL.
Represents an integer variable.
Abstract Syntax Tree (AST) related implementations.
NameVector depend_vars
dependent variables
void set_depend_vars(NameVector &&depend_vars)
Setter for member variable TableStatement::depend_vars (rvalue reference)
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
TableStatement * clone() const override
Return a copy of the current node.
const ModToken * get_token() const noexcept override
Return associated token for the current ast node.
AstNodeType
Enum type for every AST node type.
Auto generated AST classes declaration.
void set_with(std::shared_ptr< Integer > &&with)
Setter for member variable TableStatement::with (rvalue reference)
virtual ~TableStatement()=default
std::shared_ptr< Expression > get_from() const noexcept
Getter for member variable TableStatement::from.
bool is_table_statement() const noexcept override
Check if the ast node is an instance of ast::TableStatement.
std::shared_ptr< Expression > get_to() const noexcept
Getter for member variable TableStatement::to.
std::vector< std::shared_ptr< Name > > NameVector
void set_from(std::shared_ptr< Expression > &&from)
Setter for member variable TableStatement::from (rvalue reference)
std::string get_nmodl_name() const noexcept override
Return NMODL statement of ast node as std::string.
Abstract base class for all visitors implementation.
void set_token(const ModToken &tok)
Set token for the current ast node.
TableStatement(const NameVector &table_vars, const NameVector &depend_vars, Expression *from, Expression *to, Integer *with)
std::shared_ptr< const Ast > get_shared_ptr() const override
Get std::shared_ptr from this pointer of the current ast node.
std::shared_ptr< Expression > to
to values
std::shared_ptr< Ast > get_shared_ptr() override
Get std::shared_ptr from this pointer of the current ast node.
const NameVector & get_table_vars() const noexcept
Getter for member variable TableStatement::table_vars.
void visit_children(visitor::Visitor &v) override
visit children i.e.
Base class for all expressions in the NMODL.
std::string get_node_type_name() const noexcept override
Return type (ast::AstNodeType) of ast node as std::string.
@ TABLE_STATEMENT
type of ast::TableStatement
std::shared_ptr< Expression > from
from value
AstNodeType get_node_type() const noexcept override
Return type (ast::AstNodeType) of ast node.
Represent token returned by scanner.