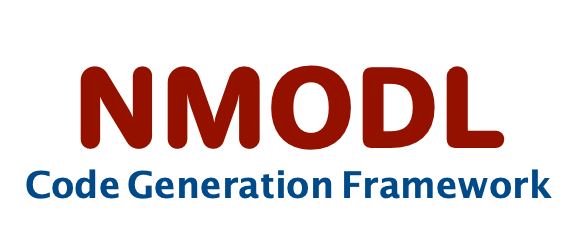 |
User Guide
|
Go to the documentation of this file.
46 std::shared_ptr<ModToken>
token;
132 return std::static_pointer_cast<Include>(shared_from_this());
139 return std::static_pointer_cast<const Include>(shared_from_this());
Abstract base class for all constant visitors implementation.
Represents an INCLUDE statement in NMODL.
std::shared_ptr< ModToken > token
token with location information
std::string get_nmodl_name() const noexcept override
Return NMODL statement of ast node as std::string.
std::shared_ptr< const Ast > get_shared_ptr() const override
Get std::shared_ptr from this pointer of the current ast node.
void set_token(const ModToken &tok)
Set token for the current ast node.
Auto generated AST classes declaration.
THIS FILE IS GENERATED AT BUILD TIME AND SHALL NOT BE EDITED.
std::vector< std::shared_ptr< Node > > NodeVector
std::string get_node_type_name() const noexcept override
Return type (ast::AstNodeType) of ast node as std::string.
NodeVector blocks
AST of the included file.
void set_parent_in_children()
Set this object as parent for all the children.
bool is_include() const noexcept override
Check if the ast node is an instance of ast::Include.
Abstract Syntax Tree (AST) related implementations.
virtual ~Include()=default
AstNodeType
Enum type for every AST node type.
Auto generated AST classes declaration.
AstNodeType get_node_type() const noexcept override
Return type (ast::AstNodeType) of ast node.
Include(String *filename, const NodeVector &blocks)
Abstract base class for all visitors implementation.
const NodeVector & get_blocks() const noexcept
Getter for member variable Include::blocks.
void set_blocks(NodeVector &&blocks)
Setter for member variable Include::blocks (rvalue reference)
void visit_children(visitor::Visitor &v) override
visit children i.e.
const ModToken * get_token() const noexcept override
Return associated token for the current ast node.
std::shared_ptr< String > filename
path to the file to include
std::shared_ptr< Ast > get_shared_ptr() override
Get std::shared_ptr from this pointer of the current ast node.
void set_filename(std::shared_ptr< String > &&filename)
Setter for member variable Include::filename (rvalue reference)
Include * clone() const override
Return a copy of the current node.
@ INCLUDE
type of ast::Include
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
std::shared_ptr< String > get_filename() const noexcept
Getter for member variable Include::filename.
Represent token returned by scanner.