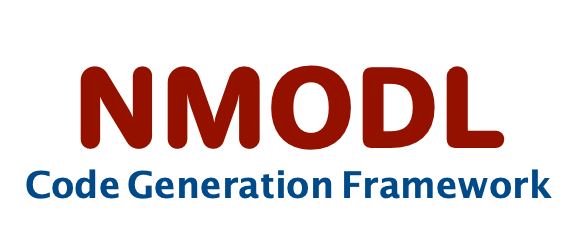 |
User Guide
|
Go to the documentation of this file.
43 std::shared_ptr<Name>
name;
53 std::shared_ptr<ModToken>
token;
139 return std::static_pointer_cast<Useion>(shared_from_this());
146 return std::static_pointer_cast<const Useion>(shared_from_this());
std::shared_ptr< String > ontology_id
Ontology to indicate the chemical ion.
Abstract base class for all constant visitors implementation.
Useion(Name *name, const ReadIonVarVector &readlist, const WriteIonVarVector &writelist, Valence *valence, String *ontology_id)
std::shared_ptr< Valence > valence
(TODO)
ReadIonVarVector readlist
Variables being read.
std::shared_ptr< String > get_ontology_id() const noexcept
Getter for member variable Useion::ontology_id.
Auto generated AST classes declaration.
THIS FILE IS GENERATED AT BUILD TIME AND SHALL NOT BE EDITED.
const WriteIonVarVector & get_writelist() const noexcept
Getter for member variable Useion::writelist.
void set_writelist(WriteIonVarVector &&writelist)
Setter for member variable Useion::writelist (rvalue reference)
Abstract Syntax Tree (AST) related implementations.
AstNodeType
Enum type for every AST node type.
Auto generated AST classes declaration.
std::shared_ptr< Valence > get_valence() const noexcept
Getter for member variable Useion::valence.
std::vector< std::shared_ptr< ReadIonVar > > ReadIonVarVector
AstNodeType get_node_type() const noexcept override
Return type (ast::AstNodeType) of ast node.
void set_readlist(ReadIonVarVector &&readlist)
Setter for member variable Useion::readlist (rvalue reference)
void set_token(const ModToken &tok)
Set token for the current ast node.
std::shared_ptr< ModToken > token
token with location information
const ReadIonVarVector & get_readlist() const noexcept
Getter for member variable Useion::readlist.
Abstract base class for all visitors implementation.
Represents USEION statement in NMODL.
std::shared_ptr< Name > name
Name of ion.
std::shared_ptr< const Ast > get_shared_ptr() const override
Get std::shared_ptr from this pointer of the current ast node.
WriteIonVarVector writelist
Variables being written.
void set_ontology_id(std::shared_ptr< String > &&ontology_id)
Setter for member variable Useion::ontology_id (rvalue reference)
std::string get_node_name() const override
Return name of the node.
const ModToken * get_token() const noexcept override
Return associated token for the current ast node.
std::shared_ptr< Name > get_name() const noexcept
Getter for member variable Useion::name.
void set_valence(std::shared_ptr< Valence > &&valence)
Setter for member variable Useion::valence (rvalue reference)
@ USEION
type of ast::Useion
Auto generated AST classes declaration.
void set_parent_in_children()
Set this object as parent for all the children.
void visit_children(visitor::Visitor &v) override
visit children i.e.
Useion * clone() const override
Return a copy of the current node.
virtual ~Useion()=default
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
std::string get_node_type_name() const noexcept override
Return type (ast::AstNodeType) of ast node as std::string.
std::vector< std::shared_ptr< WriteIonVar > > WriteIonVarVector
void set_name(std::shared_ptr< Name > &&name)
Setter for member variable Useion::name (rvalue reference)
std::string get_nmodl_name() const noexcept override
Return NMODL statement of ast node as std::string.
std::shared_ptr< Ast > get_shared_ptr() override
Get std::shared_ptr from this pointer of the current ast node.
Represent token returned by scanner.
bool is_useion() const noexcept override
Check if the ast node is an instance of ast::Useion.