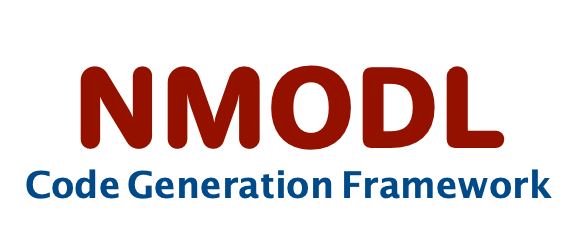 |
User Guide
|
Go to the documentation of this file.
8 #include <catch2/catch_test_macros.hpp>
15 using namespace nmodl;
16 using namespace visitor;
17 using namespace test_utils;
26 SCENARIO(
"Searching for ast nodes using AstLookupVisitor",
"[visitor][lookup]") {
27 auto to_ast = [](
const std::string& text) {
32 GIVEN(
"A mod file with nodes of type NEURON, RANGE, BinaryExpression") {
33 std::string nmodl_text = R
"(
47 auto ast = to_ast(nmodl_text);
49 WHEN(
"Looking for existing nodes") {
50 THEN(
"Can find RANGE variables") {
51 const auto& result =
collect_nodes(*ast, {AstNodeType::RANGE_VAR});
52 REQUIRE(result.size() == 2);
53 REQUIRE(
to_nmodl(result[0]) ==
"tau");
57 THEN(
"Can find NEURON block") {
58 const auto& nodes =
collect_nodes(*ast, {AstNodeType::NEURON_BLOCK});
59 REQUIRE(nodes.size() == 1);
61 const std::string neuron_block = R
"(
67 REQUIRE(result == expected);
70 THEN(
"Can find Binary Expressions and function call") {
73 {AstNodeType::BINARY_EXPRESSION, AstNodeType::FUNCTION_CALL});
74 REQUIRE(result.size() == 4);
78 WHEN(
"Looking for missing nodes") {
79 THEN(
"Can not find BREAKPOINT block") {
80 const auto& result =
collect_nodes(*ast, {AstNodeType::BREAKPOINT_BLOCK});
81 REQUIRE(result.empty());
Class that binds all pieces together for parsing nmodl file.
std::string to_nmodl(const ast::Ast &node, const std::set< ast::AstNodeType > &exclude_types)
Given AST node, return the NMODL string representation.
std::string reindent_text(const std::string &text, int indent_level)
Reindent nmodl text for text-to-text comparison.
encapsulates code generation backend implementations
AstNodeType
Enum type for every AST node type.
Utility functions for visitors implementation.
Auto generated AST classes declaration.
nmodl::parser::UnitDriver driver
SCENARIO("Searching for ast nodes using AstLookupVisitor", "[visitor][lookup]")
bool parse_string(const std::string &input)
parser Units provided as string (used for testing)
std::vector< std::shared_ptr< const ast::Ast > > collect_nodes(const ast::Ast &node, const std::vector< ast::AstNodeType > &types)
traverse node recursively and collect nodes of given types