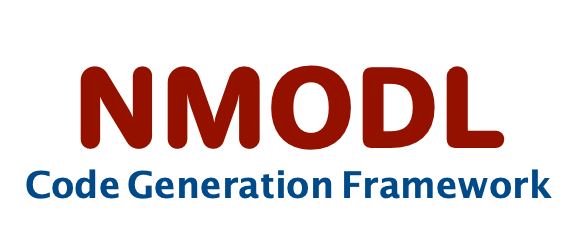 |
User Guide
|
Go to the documentation of this file.
8 #include <catch2/catch_test_macros.hpp>
20 using namespace nmodl;
21 using namespace visitor;
23 using namespace test_utils;
36 SymtabVisitor().visit_program(*ast);
37 ConstantFolderVisitor().visit_program(*ast);
38 LoopUnrollVisitor().visit_program(*ast);
39 ConstantFolderVisitor().visit_program(*ast);
42 CheckParentVisitor().check_ast(*ast);
44 return to_nmodl(ast, {AstNodeType::DEFINE});
47 SCENARIO(
"Perform loop unrolling of FROM construct",
"[visitor][unroll]") {
48 GIVEN(
"A loop with known iteration space") {
49 std::string input_nmodl = R
"(
56 FROM i=(0+(0+1)) TO (N+2-1) {
57 x[(i+0)] = x[i+1] + 11
62 ~ ca[i] <-> ca[i+1] (DFree*frat[i+1]*1(um), DFree*frat[i+1]*1(um))
66 std::string output_nmodl = R"(
83 ~ ca[1] <-> ca[2] (DFree*frat[2]*1(um), DFree*frat[2]*1(um))
84 ~ ca[2] <-> ca[3] (DFree*frat[3]*1(um), DFree*frat[3]*1(um))
85 ~ ca[3] <-> ca[4] (DFree*frat[4]*1(um), DFree*frat[4]*1(um))
89 THEN("Loop body gets correctly unrolled") {
95 GIVEN(
"A nested loop") {
96 std::string input_nmodl = R
"(
107 std::string output_nmodl = R"(
122 THEN("Loop get unrolled recursively") {
129 GIVEN(
"Loop with verbatim and unknown iteration space") {
130 std::string input_nmodl = R
"(
134 FROM i=((0+0)) TO (((N+0))) {
144 std::string output_nmodl = R"(
160 THEN("Only some loops get unrolled") {
Class that binds all pieces together for parsing nmodl file.
std::string to_nmodl(const ast::Ast &node, const std::set< ast::AstNodeType > &exclude_types)
Given AST node, return the NMODL string representation.
std::string reindent_text(const std::string &text, int indent_level)
Reindent nmodl text for text-to-text comparison.
encapsulates code generation backend implementations
std::string run_loop_unroll_visitor(const std::string &text)
Unroll for loop in the AST.
Perform constant folding of integer/float/double expressions.
AstNodeType
Enum type for every AST node type.
Utility functions for visitors implementation.
Auto generated AST classes declaration.
nmodl::parser::UnitDriver driver
bool parse_string(const std::string &input)
parser Units provided as string (used for testing)
Visitor for checking parents of ast nodes
THIS FILE IS GENERATED AT BUILD TIME AND SHALL NOT BE EDITED.
THIS FILE IS GENERATED AT BUILD TIME AND SHALL NOT BE EDITED.
SCENARIO("Perform loop unrolling of FROM construct", "[visitor][unroll]")