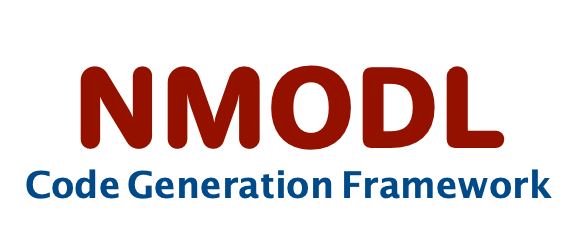 |
User Guide
|
Go to the documentation of this file.
8 #include <catch2/catch_test_macros.hpp>
19 using namespace nmodl;
20 using namespace visitor;
22 using namespace test_utils;
38 CheckParentVisitor v4(
true);
39 v1.visit_program(*ast);
40 v2.visit_program(*ast);
41 v3.visit_program(*ast);
44 v1.visit_program(*ast);
45 v1.visit_program(*ast);
47 v2.visit_program(*ast);
48 v3.visit_program(*ast);
49 v2.visit_program(*ast);
55 SCENARIO(
"Running visitor passes multiple times",
"[visitor]") {
57 std::string nmodl_text = R
"(
68 THEN("Passes can run multiple times") {
79 SCENARIO(
"Sympy specific AST to NMODL conversion") {
80 GIVEN(
"NMODL block with unit usage") {
81 static const std::string
nmodl = R
"(
83 Pf_NMDA = (1/1.38) * 120 (mM) * 0.6
84 VDCC = gca_bar_VDCC * 4(um2)*PI*3(1/um3)
85 gca_bar_VDCC = 0.0372 (nS/um2)
89 static const std::string expected = R
"(
91 Pf_NMDA = (1/1.38)*120*0.6
92 VDCC = gca_bar_VDCC*4*PI*3
97 THEN("to_nmodl can ignore all units") {
101 const auto& result =
to_nmodl(ast, {AstNodeType::UNIT});
Class that binds all pieces together for parsing nmodl file.
Visitor to transform global variable usage to local
std::string to_nmodl(const ast::Ast &node, const std::set< ast::AstNodeType > &exclude_types)
Given AST node, return the NMODL string representation.
std::string reindent_text(const std::string &text, int indent_level)
Reindent nmodl text for text-to-text comparison.
encapsulates code generation backend implementations
AstNodeType
Enum type for every AST node type.
Utility functions for visitors implementation.
Auto generated AST classes declaration.
SCENARIO("Running visitor passes multiple times", "[visitor]")
nmodl::parser::UnitDriver driver
void run_visitor_passes(const std::string &text)
bool parse_string(const std::string &input)
parser Units provided as string (used for testing)
Visitor for checking parents of ast nodes
Visitor to inline local procedure and function calls
THIS FILE IS GENERATED AT BUILD TIME AND SHALL NOT BE EDITED.