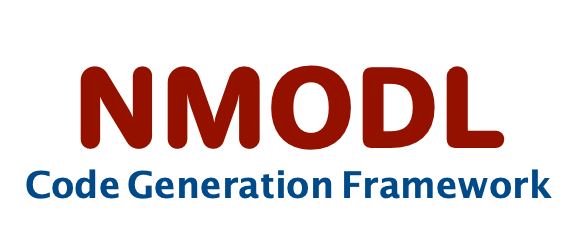 |
User Guide
|
Go to the documentation of this file.
11 #include <catch2/catch_test_macros.hpp>
17 TEST_CASE(
"JSON printer converting object to string form",
"[printer][json]") {
18 SECTION(
"Stringstream test 1") {
28 auto result = R
"({"A":[{"name":"B"}]})";
29 REQUIRE(ss.str() == result);
32 SECTION("Stringstream test 2") {
46 auto result = R
"({"A":[{"name":"B"},{"name":"C"},{"D":[{"name":"E"}]}]})";
47 REQUIRE(ss.str() == result);
50 SECTION("Test with nodes as separate tags") {
64 R
"({"children":[{"name":"B"},{"children":[{"name":"E"}],"name":"D"}],"name":"A"})";
65 REQUIRE(ss.str() == result);
Helper class for printing AST in JSON form.
void add_node(std::string value, const std::string &key="name")
Add node to json (typically basic type)
Helper class for printing AST in JSON form.
void compact_json(bool flag)
print json in compact mode
void push_block(const std::string &value, const std::string &key="name")
Add new json object (typically start of new block) name here is type of new block encountered.
void pop_block()
We finished processing a block, add processed block to it's parent block.
void flush()
Dump json object to stream (typically at the end) nspaces is number of spaces used for indentation.
void expand_keys(bool flag)
TEST_CASE("JSON printer converting object to string form", "[printer][json]")