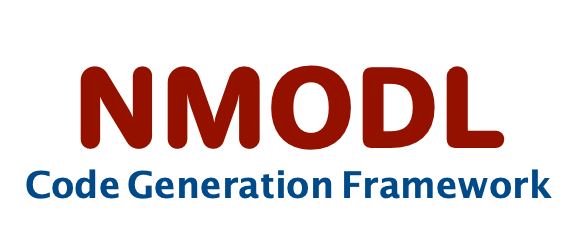 |
User Guide
|
Go to the documentation of this file.
8 #include <catch2/catch_test_macros.hpp>
21 using namespace nmodl;
22 using namespace visitor;
24 using namespace test_utils;
35 const std::string& text,
36 const std::vector<AstNodeType>& ret_nodetypes = {AstNodeType::SOLVE_BLOCK,
37 AstNodeType::DERIVATIVE_BLOCK}) {
38 std::vector<std::string> results;
44 SymtabVisitor().visit_program(*ast);
47 ConstantFolderVisitor().visit_program(*ast);
48 LoopUnrollVisitor().visit_program(*ast);
49 ConstantFolderVisitor().visit_program(*ast);
50 SymtabVisitor().visit_program(*ast);
54 KineticBlockVisitor().visit_program(*ast);
55 SymtabVisitor().visit_program(*ast);
58 SteadystateVisitor().visit_program(*ast);
62 results.reserve(res.size());
63 for (
const auto& r: res) {
68 CheckParentVisitor().check_ast(*ast);
73 SCENARIO(
"Solving ODEs with STEADYSTATE solve method",
"[visitor][steadystate]") {
74 GIVEN(
"STEADYSTATE sparse solve") {
75 std::string nmodl_text = R
"(
77 SOLVE states STEADYSTATE sparse
83 std::string expected_text1 = R"(
87 std::string expected_text2 = R"(
88 DERIVATIVE states_steadystate {
92 THEN("Construct DERIVATIVE block with steadystate solution & update SOLVE statement") {
94 REQUIRE(result.size() == 3);
95 REQUIRE(result[0] ==
"SOLVE states_steadystate METHOD sparse");
100 GIVEN(
"STEADYSTATE derivimplicit solve") {
101 std::string nmodl_text = R
"(
103 SOLVE states STEADYSTATE derivimplicit
109 std::string expected_text1 = R"(
113 std::string expected_text2 = R"(
114 DERIVATIVE states_steadystate {
118 THEN("Construct DERIVATIVE block with steadystate solution & update SOLVE statement") {
120 REQUIRE(result.size() == 3);
121 REQUIRE(result[0] ==
"SOLVE states_steadystate METHOD derivimplicit");
126 GIVEN(
"two STEADYSTATE solves") {
127 std::string nmodl_text = R
"(
133 SOLVE states0 STEADYSTATE derivimplicit
134 SOLVE states1 STEADYSTATE sparse
138 Z'[1] = Z[0] + 2*Z[2]
139 Z'[2] = Z[0]*Z[0] - 3.10
145 std::string expected_text1 = R"(
149 Z'[2] = Z[0]*Z[0]-3.10
151 std::string expected_text2 = R"(
155 std::string expected_text3 = R"(
156 DERIVATIVE states0_steadystate {
160 Z'[2] = Z[0]*Z[0]-3.10
162 std::string expected_text4 = R"(
163 DERIVATIVE states1_steadystate {
167 THEN("Construct DERIVATIVE blocks with steadystate solution & update SOLVE statements") {
169 REQUIRE(result.size() == 6);
170 REQUIRE(result[0] ==
"SOLVE states0_steadystate METHOD derivimplicit");
171 REQUIRE(result[1] ==
"SOLVE states1_steadystate METHOD sparse");
Class that binds all pieces together for parsing nmodl file.
std::string to_nmodl(const ast::Ast &node, const std::set< ast::AstNodeType > &exclude_types)
Given AST node, return the NMODL string representation.
std::vector< std::string > run_steadystate_visitor(const std::string &text, const std::vector< AstNodeType > &ret_nodetypes={AstNodeType::SOLVE_BLOCK, AstNodeType::DERIVATIVE_BLOCK})
std::string reindent_text(const std::string &text, int indent_level)
Reindent nmodl text for text-to-text comparison.
encapsulates code generation backend implementations
Unroll for loop in the AST.
Perform constant folding of integer/float/double expressions.
AstNodeType
Enum type for every AST node type.
Visitor for kinetic block statements
Visitor for STEADYSTATE solve statements
Utility functions for visitors implementation.
Auto generated AST classes declaration.
nmodl::parser::UnitDriver driver
bool parse_string(const std::string &input)
parser Units provided as string (used for testing)
std::vector< std::shared_ptr< const ast::Ast > > collect_nodes(const ast::Ast &node, const std::vector< ast::AstNodeType > &types)
traverse node recursively and collect nodes of given types
Visitor for checking parents of ast nodes
THIS FILE IS GENERATED AT BUILD TIME AND SHALL NOT BE EDITED.
SCENARIO("Solving ODEs with STEADYSTATE solve method", "[visitor][steadystate]")