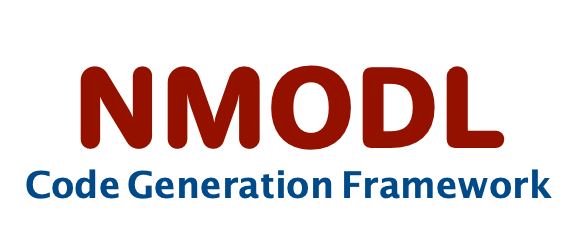 |
User Guide
|
Go to the documentation of this file.
119 nodes.push_back(node);
129 nodes.push_back(node);
176 if (new_order >
order) {
186 value = std::make_shared<double>(val);
219 const std::shared_ptr<double>&
get_value() const noexcept {
244 nodes.push_back(node);
247 const std::vector<ast::Ast*>&
get_nodes() const noexcept {
252 std::initializer_list<ast::AstNodeType> l)
const noexcept;
290 return static_cast<bool>(
properties & new_properties);
295 return ((
properties & new_properties) == new_properties);
300 return static_cast<bool>(
status & new_status);
305 return ((
status & new_status) == new_status);
int definition_order
order in which symbol appears in the mod file Different variables appear in different blocks (NEURON,...
void set_as_array(int len) noexcept
@ from_state
derived from state
@ write_ion_var
Write Ion.
std::shared_ptr< double > value
associated value in case of parameters, constant variable
void set_id(int i) noexcept
const std::vector< ast::Ast * > & get_nodes() const noexcept
@ extern_method
neuron solver methods and math functions
std::string renamed_from
original name of the symbol if renamed
@ thread_safe
variable marked as thread safe
Base class for all Abstract Syntax Tree node types.
bool has_all_status(syminfo::Status new_status) const noexcept
check if symbol has all of the status
Symbol(std::string name, ModToken token)
Represent symbol in symbol table.
void set_value(double val)
THIS FILE IS GENERATED AT BUILD TIME AND SHALL NOT BE EDITED.
encapsulates code generation backend implementations
int get_num_values() const noexcept
int num_values
number of values that variable can take in case of table variable
bool is_writable() const noexcept
bool array
true if symbol represent array variable
Implement various classes to represent various Symbol properties.
Status
state during various compiler passes
void add_node(ast::Ast *node) noexcept
int get_length() const noexcept
@ range_var
Range Variable.
int get_read_count() const noexcept
void mark_created() noexcept
mark symbol as newly created (in case of new variable)
ModToken token
token associated with symbol (from node)
Symbol(std::string name, ast::Ast *node)
const syminfo::Status & get_status() const noexcept
std::string scope
scope of the symbol (nmodl block name where it appears)
const syminfo::NmodlType & get_properties() const noexcept
int id
unique id or index position when symbol is inserted into specific table
bool is_array() const noexcept
std::vector< ast::Ast * > nodes
All given AST nodes for this symbol.
int read_count
number of times symbol is read
void mark_renamed() noexcept
bool has_any_property(syminfo::NmodlType new_properties) const noexcept
check if symbol has any of the given property
int get_id() const noexcept
void set_num_values(int n) noexcept
void set_scope(const std::string &s)
const std::string & get_name() const noexcept
void set_definition_order(int order) noexcept
const ModToken & get_token() const noexcept
void add_property(syminfo::NmodlType property) noexcept
add new property to symbol
const std::string & get_scope() const noexcept
bool is_external_variable() const noexcept
Check if symbol represent an external variable.
syminfo::Status status
status of symbol after processing through various passes
void mark_inlined() noexcept
mark symbol as inlined (in case of procedure/function)
@ assigned_definition
Assigned Definition.
std::string name
name of the symbol
void read() noexcept
increment read count
NmodlType
NMODL variable properties.
int length
dimension/length in case of array variable
void add_properties(syminfo::NmodlType new_properties) noexcept
add new properties to symbol
int get_write_count() const noexcept
void remove_property(syminfo::NmodlType property)
remove property from symbol
int get_definition_order() const noexcept
bool has_any_status(syminfo::Status new_status) const noexcept
check if symbol has any of the status
@ state_var
state variable
std::vector< ast::Ast * > get_nodes_by_type(std::initializer_list< ast::AstNodeType > l) const noexcept
const std::shared_ptr< double > & get_value() const noexcept
Symbol(std::string name, ast::Ast *node, ModToken token)
void created_from_state() noexcept
mark symbol as newly created variable for the STATE variable this is used with legacy euler/derivimpl...
void write() noexcept
increment write count
void set_original_name(const std::string &new_name)
void mark_thread_safe() noexcept
int write_count
number of times symbol is written
bool is_variable() const noexcept
check if symbol is a variable in nmodl
const std::string & get_original_name() const noexcept
bool has_all_properties(syminfo::NmodlType new_properties) const noexcept
check if symbol has all of the given properties
int order
order in case of state / prime variable
Represent token returned by scanner.
@ localized
converted to local
syminfo::NmodlType properties
properties of symbol as a result of usage across whole mod file
void set_name(const std::string &new_name)
Set new name for the symbol.
void mark_localized() noexcept
mark symbol as localized (e.g. from RANGE to LOCAL conversion)
void set_order(int new_order) noexcept
Set order in case of prime/state variable.
std::string to_string() const
@ extern_neuron_variable
neuron variable accessible in mod file