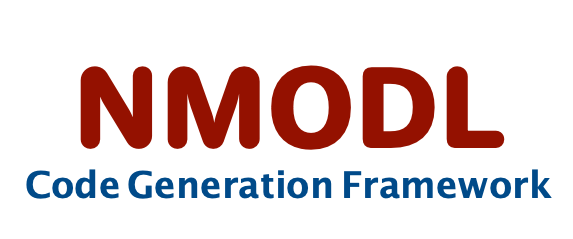 |
User Guide
|
Go to the documentation of this file.
55 std::shared_ptr<Expression>
lhs;
59 std::shared_ptr<Expression>
rhs;
61 std::shared_ptr<ModToken>
token;
125 return "BinaryExpression";
133 return std::static_pointer_cast<BinaryExpression>(shared_from_this());
140 return std::static_pointer_cast<const BinaryExpression>(shared_from_this());
161 std::shared_ptr<Expression>
get_lhs() const noexcept {
179 std::shared_ptr<Expression>
get_rhs() const noexcept {
194 void set_lhs(std::shared_ptr<Expression>&&
lhs);
199 void set_lhs(
const std::shared_ptr<Expression>&
lhs);
216 void set_rhs(std::shared_ptr<Expression>&&
rhs);
221 void set_rhs(
const std::shared_ptr<Expression>&
rhs);
BinaryOperator op
Operator.
Abstract base class for all constant visitors implementation.
std::shared_ptr< Expression > rhs
RHS of the binary expression.
std::shared_ptr< const Ast > get_shared_ptr() const override
Get std::shared_ptr from this pointer of the current ast node.
@ BINARY_EXPRESSION
type of ast::BinaryExpression
std::shared_ptr< Ast > get_shared_ptr() override
Get std::shared_ptr from this pointer of the current ast node.
void set_token(const ModToken &tok)
Set token for the current ast node.
bool is_binary_expression() const noexcept override
Check if the ast node is an instance of ast::BinaryExpression.
THIS FILE IS GENERATED AT BUILD TIME AND SHALL NOT BE EDITED.
Auto generated AST classes declaration.
void set_op(BinaryOperator &&op)
Setter for member variable BinaryExpression::op (rvalue reference)
Abstract Syntax Tree (AST) related implementations.
std::shared_ptr< Expression > get_rhs() const noexcept
Getter for member variable BinaryExpression::rhs.
AstNodeType
Enum type for every AST node type.
AstNodeType get_node_type() const noexcept override
Return type (ast::AstNodeType) of ast node.
void set_rhs(std::shared_ptr< Expression > &&rhs)
Setter for member variable BinaryExpression::rhs (rvalue reference)
void set_lhs(std::shared_ptr< Expression > &&lhs)
Setter for member variable BinaryExpression::lhs (rvalue reference)
Abstract base class for all visitors implementation.
void set_parent_in_children()
Set this object as parent for all the children.
const BinaryOperator & get_op() const noexcept
Getter for member variable BinaryExpression::op.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
std::shared_ptr< Expression > lhs
LHS of the binary expression.
std::shared_ptr< ModToken > token
token with location information
void visit_children(visitor::Visitor &v) override
visit children i.e.
virtual ~BinaryExpression()=default
BinaryExpression(Expression *lhs, const BinaryOperator &op, Expression *rhs)
Operator used in ast::BinaryExpression.
BinaryExpression * clone() const override
Return a copy of the current node.
std::shared_ptr< Expression > get_lhs() const noexcept
Getter for member variable BinaryExpression::lhs.
Auto generated AST classes declaration.
Base class for all expressions in the NMODL.
std::string get_node_type_name() const noexcept override
Return type (ast::AstNodeType) of ast node as std::string.
const ModToken * get_token() const noexcept override
Return associated token for the current ast node.
Represents binary expression in the NMODL.
Represent token returned by scanner.