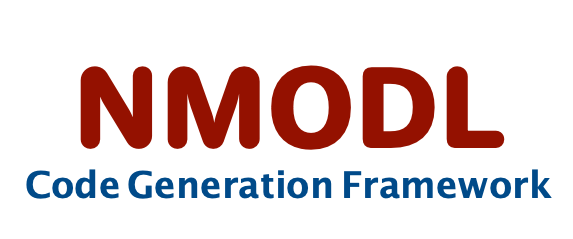 |
User Guide
|
Go to the documentation of this file.
43 std::shared_ptr<ModToken>
token;
107 return "DerivimplicitCallback";
115 return std::static_pointer_cast<DerivimplicitCallback>(shared_from_this());
122 return std::static_pointer_cast<const DerivimplicitCallback>(shared_from_this());
Abstract base class for all constant visitors implementation.
std::shared_ptr< Block > get_node_to_solve() const noexcept
Getter for member variable DerivimplicitCallback::node_to_solve.
AstNodeType get_node_type() const noexcept override
Return type (ast::AstNodeType) of ast node.
@ DERIVIMPLICIT_CALLBACK
type of ast::DerivimplicitCallback
void set_node_to_solve(std::shared_ptr< Block > &&node_to_solve)
Setter for member variable DerivimplicitCallback::node_to_solve (rvalue reference)
void set_parent_in_children()
Set this object as parent for all the children.
virtual ~DerivimplicitCallback()=default
THIS FILE IS GENERATED AT BUILD TIME AND SHALL NOT BE EDITED.
Abstract Syntax Tree (AST) related implementations.
std::shared_ptr< const Ast > get_shared_ptr() const override
Get std::shared_ptr from this pointer of the current ast node.
AstNodeType
Enum type for every AST node type.
Base class for all block scoped nodes.
const ModToken * get_token() const noexcept override
Return associated token for the current ast node.
DerivimplicitCallback(Block *node_to_solve)
Abstract base class for all visitors implementation.
void visit_children(visitor::Visitor &v) override
visit children i.e.
void set_token(const ModToken &tok)
Set token for the current ast node.
bool is_derivimplicit_callback() const noexcept override
Check if the ast node is an instance of ast::DerivimplicitCallback.
std::shared_ptr< Block > node_to_solve
Block to be solved (typically derivative)
Represent a callback to NEURON's derivimplicit solver.
std::string get_node_type_name() const noexcept override
Return type (ast::AstNodeType) of ast node as std::string.
DerivimplicitCallback * clone() const override
Return a copy of the current node.
std::shared_ptr< ModToken > token
token with location information
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
std::shared_ptr< Ast > get_shared_ptr() override
Get std::shared_ptr from this pointer of the current ast node.
Auto generated AST classes declaration.
Base class for all expressions in the NMODL.
Represent token returned by scanner.