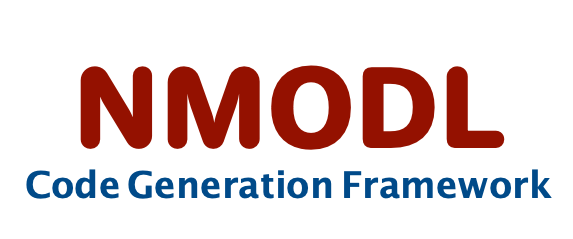 |
User Guide
|
Go to the documentation of this file.
58 std::shared_ptr<ModToken>
token;
130 return std::static_pointer_cast<Double>(shared_from_this());
137 return std::static_pointer_cast<const Double>(shared_from_this());
173 void set(std::string _value) {
244 return std::stod(
value);
Abstract base class for all constant visitors implementation.
std::shared_ptr< ModToken > token
token with location information
bool is_double() const noexcept override
Check if the ast node is an instance of ast::Double.
std::string eval() const
Return value of the ast node.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
Represents a double variable.
virtual ~Double()=default
THIS FILE IS GENERATED AT BUILD TIME AND SHALL NOT BE EDITED.
std::string get_node_type_name() const noexcept override
Return type (ast::AstNodeType) of ast node as std::string.
const ModToken * get_token() const noexcept override
Return associated token for the current ast node.
double to_double() override
Return value of the current ast node as double.
std::shared_ptr< Ast > get_shared_ptr() override
Get std::shared_ptr from this pointer of the current ast node.
const std::string & get_value() const noexcept
Getter for member variable Double::value.
Auto generated AST classes declaration.
Abstract Syntax Tree (AST) related implementations.
void set_value(std::string value)
Setter for member variable Double::value.
void visit_children(visitor::Visitor &v) override
visit children i.e.
AstNodeType
Enum type for every AST node type.
@ DOUBLE
type of ast::Double
Abstract base class for all visitors implementation.
void set(std::string _value)
Set new value to the current ast node.
std::shared_ptr< const Ast > get_shared_ptr() const override
Get std::shared_ptr from this pointer of the current ast node.
void negate() override
Negate the value of current ast node.
AstNodeType get_node_type() const noexcept override
Return type (ast::AstNodeType) of ast node.
Double * clone() const override
Return a copy of the current node.
std::string value
Value of double.
void set_parent_in_children()
Set this object as parent for all the children.
Base class for all numbers.
void set_token(const ModToken &tok)
Set token for the current ast node.
Represent token returned by scanner.