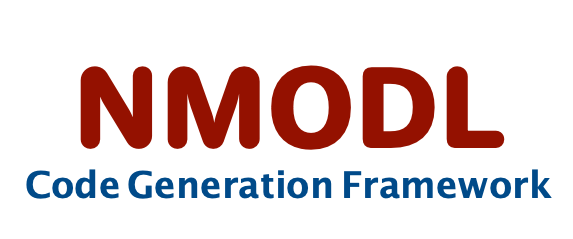 |
User Guide
|
Go to the documentation of this file.
41 std::shared_ptr<Name>
name;
43 std::shared_ptr<Expression>
from;
45 std::shared_ptr<Expression>
to;
51 std::shared_ptr<ModToken>
token;
115 return "FromStatement";
137 return std::static_pointer_cast<FromStatement>(shared_from_this());
144 return std::static_pointer_cast<const FromStatement>(shared_from_this());
186 std::shared_ptr<Expression>
get_from() const noexcept {
195 std::shared_ptr<Expression>
get_to() const noexcept {
250 void set_to(std::shared_ptr<Expression>&&
to);
255 void set_to(
const std::shared_ptr<Expression>&
to);
Abstract base class for all constant visitors implementation.
virtual ~FromStatement()=default
void set_to(std::shared_ptr< Expression > &&to)
Setter for member variable FromStatement::to (rvalue reference)
std::shared_ptr< StatementBlock > statement_block
TODO.
FromStatement * clone() const override
Return a copy of the current node.
std::shared_ptr< Expression > to
TODO.
std::shared_ptr< Ast > get_shared_ptr() override
Get std::shared_ptr from this pointer of the current ast node.
void set_statement_block(std::shared_ptr< StatementBlock > &&statement_block)
Setter for member variable FromStatement::statement_block (rvalue reference)
void set_token(const ModToken &tok)
Set token for the current ast node.
THIS FILE IS GENERATED AT BUILD TIME AND SHALL NOT BE EDITED.
void visit_children(visitor::Visitor &v) override
visit children i.e.
Abstract Syntax Tree (AST) related implementations.
void set_parent_in_children()
Set this object as parent for all the children.
std::shared_ptr< Expression > from
TODO.
std::shared_ptr< Expression > get_increment() const noexcept
Getter for member variable FromStatement::increment.
AstNodeType
Enum type for every AST node type.
bool is_from_statement() const noexcept override
Check if the ast node is an instance of ast::FromStatement.
Auto generated AST classes declaration.
std::shared_ptr< Expression > get_from() const noexcept
Getter for member variable FromStatement::from.
Abstract base class for all visitors implementation.
FromStatement(Name *name, Expression *from, Expression *to, Expression *increment, StatementBlock *statement_block)
std::string get_nmodl_name() const noexcept override
Return NMODL statement of ast node as std::string.
void set_increment(std::shared_ptr< Expression > &&increment)
Setter for member variable FromStatement::increment (rvalue reference)
const ModToken * get_token() const noexcept override
Return associated token for the current ast node.
std::shared_ptr< Name > get_name() const noexcept
Getter for member variable FromStatement::name.
std::string get_node_type_name() const noexcept override
Return type (ast::AstNodeType) of ast node as std::string.
Represents block encapsulating list of statements.
std::shared_ptr< Name > name
TODO.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
void set_name(std::shared_ptr< Name > &&name)
Setter for member variable FromStatement::name (rvalue reference)
std::shared_ptr< const Ast > get_shared_ptr() const override
Get std::shared_ptr from this pointer of the current ast node.
std::shared_ptr< StatementBlock > get_statement_block() const noexcept override
Getter for member variable FromStatement::statement_block.
AstNodeType get_node_type() const noexcept override
Return type (ast::AstNodeType) of ast node.
std::shared_ptr< Expression > get_to() const noexcept
Getter for member variable FromStatement::to.
Base class for all expressions in the NMODL.
std::shared_ptr< Expression > increment
TODO.
@ FROM_STATEMENT
type of ast::FromStatement
std::shared_ptr< ModToken > token
token with location information
Represent token returned by scanner.
void set_from(std::shared_ptr< Expression > &&from)
Setter for member variable FromStatement::from (rvalue reference)
std::string get_node_name() const override
Return name of the node.