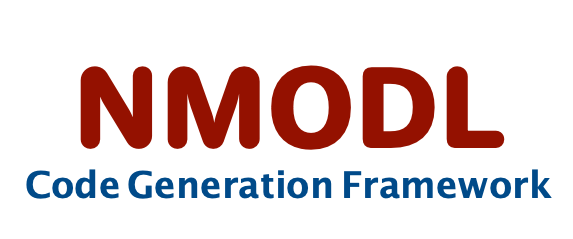 |
User Guide
|
Go to the documentation of this file.
48 std::shared_ptr<ElseStatement>
elses;
50 std::shared_ptr<ModToken>
token;
114 return "IfStatement";
136 return std::static_pointer_cast<IfStatement>(shared_from_this());
143 return std::static_pointer_cast<const IfStatement>(shared_from_this());
191 std::shared_ptr<ElseStatement>
get_elses() const noexcept {
std::shared_ptr< ModToken > token
token with location information
void visit_children(visitor::Visitor &v) override
visit children i.e.
Abstract base class for all constant visitors implementation.
std::string get_nmodl_name() const noexcept override
Return NMODL statement of ast node as std::string.
void set_elses(std::shared_ptr< ElseStatement > &&elses)
Setter for member variable IfStatement::elses (rvalue reference)
void set_statement_block(std::shared_ptr< StatementBlock > &&statement_block)
Setter for member variable IfStatement::statement_block (rvalue reference)
THIS FILE IS GENERATED AT BUILD TIME AND SHALL NOT BE EDITED.
std::shared_ptr< ElseStatement > elses
TODO.
std::shared_ptr< StatementBlock > statement_block
TODO.
void set_token(const ModToken &tok)
Set token for the current ast node.
Abstract Syntax Tree (AST) related implementations.
IfStatement(Expression *condition, StatementBlock *statement_block, const ElseIfStatementVector &elseifs, ElseStatement *elses)
const ModToken * get_token() const noexcept override
Return associated token for the current ast node.
AstNodeType
Enum type for every AST node type.
Auto generated AST classes declaration.
Auto generated AST classes declaration.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
const ElseIfStatementVector & get_elseifs() const noexcept
Getter for member variable IfStatement::elseifs.
virtual ~IfStatement()=default
std::vector< std::shared_ptr< ElseIfStatement > > ElseIfStatementVector
std::shared_ptr< Expression > get_condition() const noexcept
Getter for member variable IfStatement::condition.
void set_condition(std::shared_ptr< Expression > &&condition)
Setter for member variable IfStatement::condition (rvalue reference)
Abstract base class for all visitors implementation.
ElseIfStatementVector elseifs
TODO.
std::shared_ptr< Expression > condition
TODO.
std::shared_ptr< Ast > get_shared_ptr() override
Get std::shared_ptr from this pointer of the current ast node.
void set_elseifs(ElseIfStatementVector &&elseifs)
Setter for member variable IfStatement::elseifs (rvalue reference)
std::shared_ptr< StatementBlock > get_statement_block() const noexcept override
Getter for member variable IfStatement::statement_block.
Represents block encapsulating list of statements.
std::shared_ptr< const Ast > get_shared_ptr() const override
Get std::shared_ptr from this pointer of the current ast node.
std::string get_node_type_name() const noexcept override
Return type (ast::AstNodeType) of ast node as std::string.
bool is_if_statement() const noexcept override
Check if the ast node is an instance of ast::IfStatement.
IfStatement * clone() const override
Return a copy of the current node.
std::shared_ptr< ElseStatement > get_elses() const noexcept
Getter for member variable IfStatement::elses.
AstNodeType get_node_type() const noexcept override
Return type (ast::AstNodeType) of ast node.
Base class for all expressions in the NMODL.
Represent token returned by scanner.
void set_parent_in_children()
Set this object as parent for all the children.
@ IF_STATEMENT
type of ast::IfStatement