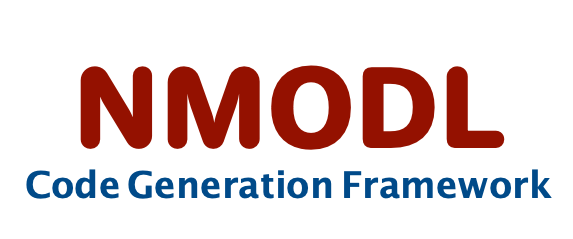 |
User Guide
|
Go to the documentation of this file.
56 std::shared_ptr<ModToken>
token;
129 return std::static_pointer_cast<Integer>(shared_from_this());
136 return std::static_pointer_cast<const Integer>(shared_from_this());
Abstract base class for all constant visitors implementation.
void set(int _value)
Set new value to the current ast node.
AstNodeType get_node_type() const noexcept override
Return type (ast::AstNodeType) of ast node.
void set_token(const ModToken &tok)
Set token for the current ast node.
int value
Value of integer.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
void set_parent_in_children()
Set this object as parent for all the children.
double to_double() override
Return value of the current ast node as double.
THIS FILE IS GENERATED AT BUILD TIME AND SHALL NOT BE EDITED.
int get_value() const noexcept
Getter for member variable Integer::value.
std::shared_ptr< Name > get_macro() const noexcept
Getter for member variable Integer::macro.
Auto generated AST classes declaration.
Represents an integer variable.
Abstract Syntax Tree (AST) related implementations.
std::shared_ptr< Ast > get_shared_ptr() override
Get std::shared_ptr from this pointer of the current ast node.
bool is_integer() const noexcept override
Check if the ast node is an instance of ast::Integer.
AstNodeType
Enum type for every AST node type.
std::string get_node_type_name() const noexcept override
Return type (ast::AstNodeType) of ast node as std::string.
std::shared_ptr< ModToken > token
token with location information
Abstract base class for all visitors implementation.
const ModToken * get_token() const noexcept override
Return associated token for the current ast node.
void visit_children(visitor::Visitor &v) override
visit children i.e.
Integer * clone() const override
Return a copy of the current node.
int eval() const
Return value of the ast node.
virtual ~Integer()=default
void set_macro(std::shared_ptr< Name > &¯o)
Setter for member variable Integer::macro (rvalue reference)
@ INTEGER
type of ast::Integer
Base class for all numbers.
std::shared_ptr< Name > macro
if integer is a macro then it's name
void negate() override
Negate the value of current ast node.
Represent token returned by scanner.
void set_value(int value)
Setter for member variable Integer::value.
std::shared_ptr< const Ast > get_shared_ptr() const override
Get std::shared_ptr from this pointer of the current ast node.