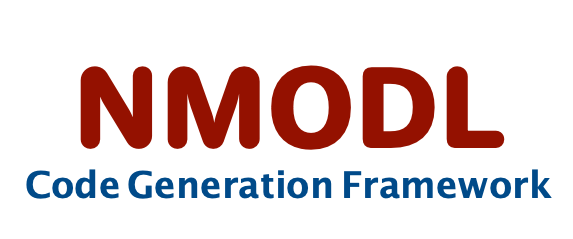 |
User Guide
|
Go to the documentation of this file.
44 std::shared_ptr<ModToken>
token;
107 return "LocalListStatement";
129 return std::static_pointer_cast<LocalListStatement>(shared_from_this());
136 return std::static_pointer_cast<const LocalListStatement>(shared_from_this());
165 LocalVarVector::const_iterator
erase_local_var(LocalVarVector::const_iterator first);
170 LocalVarVector::const_iterator
erase_local_var(LocalVarVector::const_iterator first, LocalVarVector::const_iterator last);
182 LocalVarVector::const_iterator
insert_local_var(LocalVarVector::const_iterator position,
const std::shared_ptr<LocalVar>& n);
187 template <
class NodeType,
class InputIterator>
188 void insert_local_var(LocalVarVector::const_iterator position, NodeType& to, InputIterator first, InputIterator last);
198 void reset_local_var(LocalVarVector::const_iterator position, std::shared_ptr<LocalVar> n);
291 template <
class NodeType,
class InputIterator>
294 for (
auto it = first; it != last; ++it) {
void set_variables(LocalVarVector &&variables)
Setter for member variable LocalListStatement::variables (rvalue reference)
Auto generated AST classes declaration.
void visit_children(visitor::Visitor &v) override
visit children i.e.
Abstract base class for all constant visitors implementation.
const LocalVarVector & get_variables() const noexcept
Getter for member variable LocalListStatement::variables.
void set_parent_in_children()
Set this object as parent for all the children.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
LocalVarVector::const_iterator erase_local_var(LocalVarVector::const_iterator first)
Erase member to variables.
std::string get_nmodl_name() const noexcept override
Return NMODL statement of ast node as std::string.
THIS FILE IS GENERATED AT BUILD TIME AND SHALL NOT BE EDITED.
Abstract Syntax Tree (AST) related implementations.
std::shared_ptr< const Ast > get_shared_ptr() const override
Get std::shared_ptr from this pointer of the current ast node.
std::vector< std::shared_ptr< LocalVar > > LocalVarVector
AstNodeType
Enum type for every AST node type.
Auto generated AST classes declaration.
void reset_local_var(LocalVarVector::const_iterator position, LocalVar *n)
Reset member to variables.
std::string get_node_type_name() const noexcept override
Return type (ast::AstNodeType) of ast node as std::string.
void emplace_back_local_var(LocalVar *n)
Add member to variables by raw pointer.
LocalListStatement * clone() const override
Return a copy of the current node.
Abstract base class for all visitors implementation.
LocalVarVector::const_iterator insert_local_var(LocalVarVector::const_iterator position, const std::shared_ptr< LocalVar > &n)
Insert member to variables.
LocalListStatement(const LocalVarVector &variables)
const ModToken * get_token() const noexcept override
Return associated token for the current ast node.
AstNodeType get_node_type() const noexcept override
Return type (ast::AstNodeType) of ast node.
LocalVarVector variables
TODO.
@ LOCAL_LIST_STATEMENT
type of ast::LocalListStatement
void set_token(const ModToken &tok)
Set token for the current ast node.
bool is_local_list_statement() const noexcept override
Check if the ast node is an instance of ast::LocalListStatement.
std::shared_ptr< Ast > get_shared_ptr() override
Get std::shared_ptr from this pointer of the current ast node.
std::shared_ptr< ModToken > token
token with location information
virtual ~LocalListStatement()=default
Represent token returned by scanner.