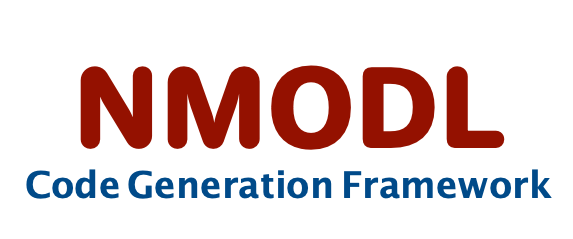 |
User Guide
|
Go to the documentation of this file.
11 #include <fmt/format.h>
44 const auto& block_name = solve_block.
get_block_name()->get_node_name();
45 const auto& solve_node_symbol =
symtab->
lookup(block_name);
46 if (solve_node_symbol ==
nullptr) {
47 throw std::runtime_error(
48 fmt::format(
"SolveBlockVisitor :: cannot find the block '{}' to solve it", block_name));
50 auto node_to_solve = solve_node_symbol->get_nodes().front();
55 std::string solve_method = method ? method->get_node_name() :
"";
65 auto block_to_solve = node_to_solve->get_statement_block();
81 node.
set_expression(std::shared_ptr<ast::SolutionExpression>(sol_expr));
std::shared_ptr< Expression > get_expression() const noexcept
Getter for member variable ExpressionStatement::expression.
@ DERIVATIVE_BLOCK
type of ast::DerivativeBlock
symtab::SymbolTable * symtab
Base class for all Abstract Syntax Tree node types.
Replace solve block statements with actual solution node in the AST.
@ EIGEN_NEWTON_SOLVER_BLOCK
type of ast::EigenNewtonSolverBlock
ast::SolutionExpression * create_solution_expression(ast::SolveBlock &solve_block)
Create solution expression node that will be used for solve block.
encapsulates code generation backend implementations
Utility functions for visitors implementation.
Represents a BREAKPOINT block in NMODL.
std::shared_ptr< Name > get_block_name() const noexcept
Getter for member variable SolveBlock::block_name.
void visit_children(visitor::Visitor &v) override
visit children i.e.
void visit_breakpoint_block(ast::BreakpointBlock &node) override
visit node of type ast::BreakpointBlock
static bool has_sympy_solution(const ast::Ast &node)
check if given node contains sympy solution
Represents DERIVATIVE block in the NMODL.
std::shared_ptr< Name > get_method() const noexcept
Getter for member variable SolveBlock::method.
std::vector< std::shared_ptr< const ast::Ast > > collect_nodes(const ast::Ast &node, const std::vector< ast::AstNodeType > &types)
traverse node recursively and collect nodes of given types
void visit_program(ast::Program &node) override
visit node of type ast::Program
void visit_children(visitor::Visitor &v) override
visit children i.e.
Represents the coreneuron nrn_state callback function.
void visit_children(visitor::Visitor &v) override
visit children i.e.
Represent a callback to NEURON's derivimplicit solver.
SolveBlock * clone() const override
Return a copy of the current node.
static constexpr char DERIVIMPLICIT_METHOD[]
derivimplicit method in nmodl
Represent solution of a block in the AST.
symtab::SymbolTable * get_symbol_table() const override
Return associated symbol table for the current ast node.
Represents top level AST node for whole NMODL input.
void emplace_back_node(Node *n)
Add member to blocks by raw pointer.
std::shared_ptr< Symbol > lookup(const std::string &name) const
check if symbol with given name exist in the current table (but not in parents)
ast::StatementVector nrn_state_solve_statements
solve expression statements for NrnState block
void visit_expression_statement(ast::ExpressionStatement &node) override
Replace solve blocks with solution expression.
Auto generated AST classes declaration.
void set_expression(std::shared_ptr< Expression > &&expression)
Setter for member variable ExpressionStatement::expression (rvalue reference)