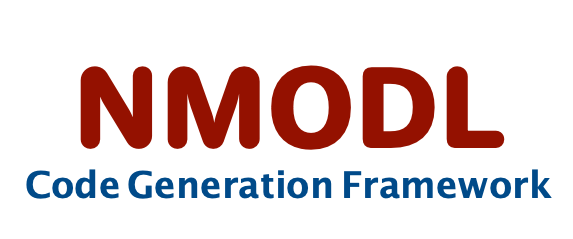 |
User Guide
|
Go to the documentation of this file.
15 #include <pybind11/embed.h>
16 #include <pybind11/stl.h>
17 #include <unordered_map>
18 #include <unordered_set>
78 const std::vector<std::string>& solutions,
94 static std::string&
replaceAll(std::string& context,
95 const std::string& from,
96 const std::string& to);
101 const std::vector<std::string>& original_vector,
102 const std::string& original_string,
103 const std::string& substitution_string);
static std::string to_nmodl_for_sympy(ast::Ast &node)
return NMODL string version of node, excluding any units
Base class for all AST node.
void visit_lin_equation(ast::LinEquation &node) override
visit node of type ast::LinEquation
std::string to_nmodl(const ast::Ast &node, const std::set< ast::AstNodeType > &exclude_types)
Given AST node, return the NMODL string representation.
void visit_linear_block(ast::LinearBlock &node) override
visit node of type ast::LinearBlock
Implement class to represent a symbol in Symbol Table.
Represents differential equation in DERIVATIVE block.
Base class for all Abstract Syntax Tree node types.
Visitor for systems of algebraic and differential equations
static std::string & replaceAll(std::string &context, const std::string &from, const std::string &to)
Function used by SympySolverVisitor::filter_X to replace the name X in a std::string to X_operator.
void visit_conserve(ast::Conserve &node) override
visit node of type ast::Conserve
void visit_non_linear_block(ast::NonLinearBlock &node) override
visit node of type ast::NonLinearBlock
Represent CONSERVE statement in NMODL.
std::string solve_method
method specified in solve block
std::vector< std::shared_ptr< Statement > > StatementVector
encapsulates code generation backend implementations
static std::vector< std::string > filter_string_vector(const std::vector< std::string > &original_vector, const std::string &original_string, const std::string &substitution_string)
Check original_vector for elements that contain a variable named original_string and rename it to sub...
std::vector< std::string > all_state_vars
vector of all state variables (in order specified in STATE block in mod file)
void init_block_data(ast::Node *node)
clear any data from previous block & get set of block local vars + global vars
std::unordered_map< std::string, std::string > derivative_block_solve_method
map between derivative block names and associated solver method
void solve_non_linear_system(const std::vector< std::string > &pre_solve_statements={})
solve non-linear system (for "derivimplicit", "sparse" and "NONLINEAR")
std::set< std::string > vars
local variables in current block + globals
std::set< std::string > global_vars
global variables
int SMALL_LINEAR_SYSTEM_MAX_STATES
max number of state vars allowed for small system linear solver
Utility functions for visitors implementation.
void visit_statement_block(ast::StatementBlock &node) override
visit node of type ast::StatementBlock
std::set< std::string > function_calls
custom function calls used in ODE block
ast::StatementBlock * block_with_expression_statements
block where expression statements appear (to check there is only one)
static void replace_diffeq_expression(ast::DiffEqExpression &expr, const std::string &new_expr)
replace binary expression with new expression provided as string
void visit_var_name(ast::VarName &node) override
visit node of type ast::VarName
void visit_expression_statement(ast::ExpressionStatement &node) override
visit node of type ast::ExpressionStatement
Concrete visitor for all AST classes.
std::set< std::string > state_vars_in_block
set of state variables used in block
@ UNIT_DEF
type of ast::UnitDef
ast::StatementVector::const_iterator get_solution_location_iterator(const ast::StatementVector &statements)
return iterator pointing to where solution should be inserted in statement block
Represents DERIVATIVE block in the NMODL.
bool use_pade_approx
optionally replace cnexp solution with (1,1) pade approx
void init_state_vars_vector()
construct vector from set of state vars in correct order
bool collect_state_vars
true for (non)linear eqs, to identify all state vars used in equations
Auto generated AST classes declaration.
std::unordered_map< std::string, std::string > conserve_equation
map from state vars to the algebraic equation from CONSERVE statement that should replace their ODE,...
std::vector< std::string > state_vars
vector of state vars used in block (in same order as all_state_vars)
void construct_eigen_solver_block(const std::vector< std::string > &pre_solve_statements, const std::vector< std::string > &solutions, bool linear)
construct solver block
Represents block encapsulating list of statements.
void visit_non_lin_equation(ast::NonLinEquation &node) override
visit node of type ast::NonLinEquation
bool elimination
optionally do CSE (common subexpression elimination) for sparse solver
Represents LINEAR block in the NMODL.
ast::ExpressionStatement * last_expression_statement
last expression statement visited (to know where to insert solutions in statement block)
Represents NONLINEAR block in the NMODL.
ast::StatementBlock * current_statement_block
current statement block being visited
SympySolverVisitor(bool use_pade_approx=false, bool elimination=true, int SMALL_LINEAR_SYSTEM_MAX_STATES=3)
void visit_derivative_block(ast::DerivativeBlock &node) override
visit node of type ast::DerivativeBlock
std::unordered_set< ast::Statement * > expression_statements
expression statements appearing in the block (these can be of type DiffEqExpression,...
void visit_program(ast::Program &node) override
visit node of type ast::Program
std::vector< std::string > eq_system
vector of {ODE, linear eq, non-linear eq} system to solve
Represents top level AST node for whole NMODL input.
bool eq_system_is_valid
only solve eq_system system of equations if this is true:
void solve_linear_system(const std::vector< std::string > &pre_solve_statements={})
solve linear system (for "LINEAR")
ast::ExpressionStatement * current_expression_statement
current expression statement being visited (to track ODEs / (non)lineqs)
void check_expr_statements_in_same_block()
raise error if kinetic/ode/(non)linear statements are spread over multiple blocks
void visit_diff_eq_expression(ast::DiffEqExpression &node) override
visit node of type ast::DiffEqExpression
Concrete visitor for all AST classes.