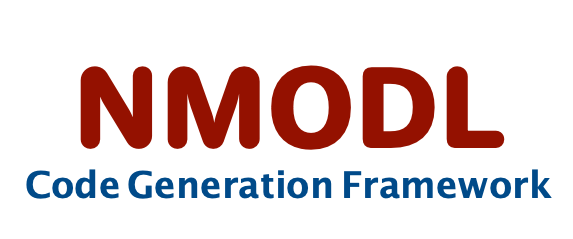 |
User Guide
|
Go to the documentation of this file.
46 std::shared_ptr<Identifier>
name;
48 std::shared_ptr<Integer>
at;
50 std::shared_ptr<Expression>
index;
52 std::shared_ptr<ModToken>
token;
58 explicit VarName(std::shared_ptr<Identifier>
name, std::shared_ptr<Integer>
at, std::shared_ptr<Expression>
index);
124 return std::static_pointer_cast<VarName>(shared_from_this());
131 return std::static_pointer_cast<const VarName>(shared_from_this());
164 std::shared_ptr<Identifier>
get_name() const noexcept {
173 std::shared_ptr<Integer>
get_at() const noexcept {
182 std::shared_ptr<Expression>
get_index() const noexcept {
208 void set_at(std::shared_ptr<Integer>&&
at);
213 void set_at(
const std::shared_ptr<Integer>&
at);
Abstract base class for all constant visitors implementation.
Base class for all identifiers.
std::shared_ptr< ModToken > token
token with location information
void set_parent_in_children()
Set this object as parent for all the children.
bool is_var_name() const noexcept override
Check if the ast node is an instance of ast::VarName.
VarName(Identifier *name, Integer *at, Expression *index)
THIS FILE IS GENERATED AT BUILD TIME AND SHALL NOT BE EDITED.
Represents an integer variable.
Abstract Syntax Tree (AST) related implementations.
std::shared_ptr< Expression > get_index() const noexcept
Getter for member variable VarName::index.
AstNodeType
Enum type for every AST node type.
std::shared_ptr< Integer > get_at() const noexcept
Getter for member variable VarName::at.
void set_name(std::shared_ptr< Identifier > &&name)
Setter for member variable VarName::name (rvalue reference)
VarName * clone() const override
Return a copy of the current node.
std::shared_ptr< Ast > get_shared_ptr() override
Get std::shared_ptr from this pointer of the current ast node.
Abstract base class for all visitors implementation.
void set_index(std::shared_ptr< Expression > &&index)
Setter for member variable VarName::index (rvalue reference)
void set_token(const ModToken &tok)
Set token for the current ast node.
const ModToken * get_token() const noexcept override
Return associated token for the current ast node.
void set_at(std::shared_ptr< Integer > &&at)
Setter for member variable VarName::at (rvalue reference)
void visit_children(visitor::Visitor &v) override
visit children i.e.
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
virtual ~VarName()=default
std::shared_ptr< Integer > at
Value specified with @
std::string get_node_type_name() const noexcept override
Return type (ast::AstNodeType) of ast node as std::string.
std::shared_ptr< Identifier > get_name() const noexcept
Getter for member variable VarName::name.
std::shared_ptr< Expression > index
index value in case of array
std::shared_ptr< const Ast > get_shared_ptr() const override
Get std::shared_ptr from this pointer of the current ast node.
std::shared_ptr< Identifier > name
Name of variable.
Auto generated AST classes declaration.
Base class for all expressions in the NMODL.
AstNodeType get_node_type() const noexcept override
Return type (ast::AstNodeType) of ast node.
Represent token returned by scanner.
std::string get_node_name() const override
Return name of the node.
@ VAR_NAME
type of ast::VarName