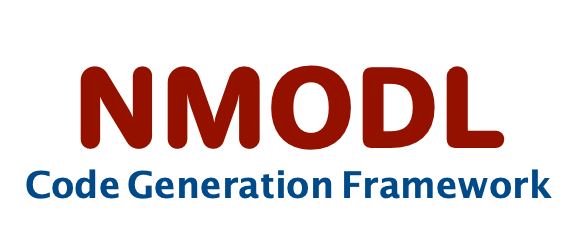 |
User Guide
|
Go to the documentation of this file.
11 #include "pybind/pybind_utils.hpp"
14 #include <pybind11/iostream.h>
15 #include <pybind11/pybind11.h>
16 #include <pybind11/stl.h>
29 namespace py = pybind11;
30 using namespace pybind11::literals;
37 static const char*
const driver = R
"(
38 This is the NmodlDriver class documentation
45 Instance of :py:class:`Program`
49 Parse NMODL provided as a string
52 input (str): C code as string
54 AST: ast root node if success, throws an exception otherwise
56 >>> ast = driver.parse_string("DEFINE NSTEP 6")
60 Parse NMODL provided as a file
63 filename (str): name of the C file
66 AST: ast root node if success, throws an exception otherwise
70 Parse NMODL file provided as istream
73 in (file): ifstream object
76 AST: ast root node if success, throws an exception otherwise
80 Given AST node, return the NMODL string representation
84 excludeTypes (set of AstNodeType): Excluded node types
87 str: NMODL string representation
89 >>> ast = driver.parse_string("NEURON{}")
90 >>> nmodl.to_nmodl(ast)
95 Given AST node, return the JSON string representation
99 compact (bool): Compact node
100 expand (bool): Expand node
103 str: JSON string representation
105 >>> ast = driver.parse_string("NEURON{}")
106 >>> nmodl.to_json(ast, True)
107 '{"Program":[{"NeuronBlock":[{"StatementBlock":[]}]}]}'
118 std::shared_ptr<nmodl::ast::Program>
parse_stream(py::object
const&
object) {
119 py::object tiob = py::module::import(
"io").attr(
"TextIOBase");
120 if (py::isinstance(
object, tiob)) {
121 py::detail::pythonibuf<py::str> buf(
object);
122 std::istream istr(&buf);
123 return NmodlDriver::parse_stream(istr);
125 py::detail::pythonibuf<py::bytes> buf(
object);
126 std::istream istr(&buf);
127 return NmodlDriver::parse_stream(istr);
140 m_nmodl.doc() =
"NMODL : Source-to-Source Code Generation Framework";
143 py::class_<nmodl::parser::NmodlDriver> _{m_nmodl,
"nmodl::parser::NmodlDriver"};
144 py::class_<nmodl::PyNmodlDriver, nmodl::parser::NmodlDriver> nmodl_driver(
146 nmodl_driver.def(py::init<>())
164 m_nmodl.def(
"to_nmodl",
166 const std::set<nmodl::ast::AstNodeType>&)
>(
169 "exclude_types"_a = std::set<nmodl::ast::AstNodeType>(),
171 m_nmodl.def(
"to_json",
176 "add_nmodl"_a =
false,
Class that binds all pieces together for parsing nmodl file.
std::string to_nmodl(const ast::Ast &node, const std::set< ast::AstNodeType > &exclude_types)
Given AST node, return the NMODL string representation.
static const char *const to_json
Base class for all Abstract Syntax Tree node types.
const std::shared_ptr< ast::Program > & get_ast() const noexcept
return previously parsed AST otherwise nullptr
void init_symtab_module(py::module &m)
static const char *const driver
encapsulates code generation backend implementations
std::string to_json(const ast::Ast &node, bool compact, bool expand, bool add_nmodl)
Given AST node, return the JSON string representation.
static const char *const to_nmodl
static const char *const driver_ast
std::shared_ptr< nmodl::ast::Program > parse_stream(py::object const &object)
Utility functions for visitors implementation.
Auto generated AST classes declaration.
nmodl::parser::UnitDriver driver
void init_ast_module(py::module &m)
PYBIND11_MODULE(_nmodl, m_nmodl)
static const char *const driver_parse_string
std::shared_ptr< ast::Program > parse_string(const std::string &input)
parser nmodl provided as string (used for testing)
static const std::string NMODL_VERSION
project tagged version in the cmake
static const char *const driver_parse_file
Version information and units file path.
Class to bridge C++ NmodlDriver with Python world using pybind11.
static const char *const driver_parse_stream
void init_visitor_module(py::module &m)
bool parse_file(const std::string &filename)
parse Units file