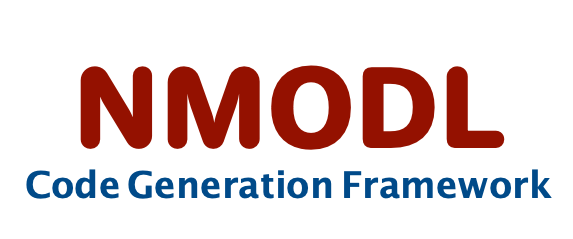 |
User Guide
|
Go to the documentation of this file.
49 const std::shared_ptr<ast::Expression>& node)
const {
50 if (node->is_name()) {
51 auto name = std::dynamic_pointer_cast<ast::Name>(node);
52 if (name->get_node_name() ==
index) {
53 return std::make_shared<ast::Integer>(
value,
nullptr);
83 static std::shared_ptr<ast::Expression>
unwrap(
const std::shared_ptr<ast::Expression>& expr) {
84 if (expr && expr->is_wrapped_expression()) {
85 const auto& e = std::dynamic_pointer_cast<ast::WrappedExpression>(expr);
86 return e->get_expression();
99 const std::shared_ptr<ast::FromStatement>& node) {
102 const auto& from =
unwrap(node->get_from());
103 const auto& to =
unwrap(node->get_to());
104 const auto& increment =
unwrap(node->get_increment());
108 if (!from->is_integer() || !to->is_integer() ||
109 (increment !=
nullptr && !increment->is_integer())) {
113 int start = std::dynamic_pointer_cast<ast::Integer>(from)->eval();
114 int end = std::dynamic_pointer_cast<ast::Integer>(to)->eval();
116 if (increment !=
nullptr) {
117 step = std::dynamic_pointer_cast<ast::Integer>(increment)->eval();
121 std::string index_var = node->get_node_name();
122 for (
int i = start; i <= end; i += step) {
124 const auto new_block = std::unique_ptr<ast::StatementBlock>(
125 node->get_statement_block()->clone());
127 statements.insert(statements.end(),
128 new_block->get_statements().begin(),
129 new_block->get_statements().end());
134 return std::make_shared<ast::ExpressionStatement>(block);
146 for (
auto iter = statements.begin(); iter != statements.end(); ++iter) {
147 if ((*iter)->is_from_statement()) {
148 const auto& statement = std::dynamic_pointer_cast<ast::FromStatement>((*iter));
152 if (!verbatim_blocks.empty()) {
153 logger->debug(
"LoopUnrollVisitor : can not unroll because of verbatim block");
159 if (new_statement !=
nullptr) {
162 const auto& before =
to_nmodl(statement);
163 const auto& after =
to_nmodl(new_statement);
164 logger->debug(
"LoopUnrollVisitor : \n {} \n unrolled to \n {}", before, after);
std::shared_ptr< Expression > get_length() const noexcept
Getter for member variable IndexedName::length.
std::string to_nmodl(const ast::Ast &node, const std::set< ast::AstNodeType > &exclude_types)
Given AST node, return the NMODL string representation.
std::string index
index variable name
void visit_children(visitor::Visitor &v) override
visit children i.e.
bool under_indexed_name
true if we are visiting index variable
std::vector< std::shared_ptr< Statement > > StatementVector
encapsulates code generation backend implementations
Unroll for loop in the AST.
Helper visitor to replace index of array variable with integer.
std::shared_ptr< Expression > get_rhs() const noexcept
Getter for member variable BinaryExpression::rhs.
void visit_statement_block(ast::StatementBlock &node) override
Parse verbatim blocks and rename variable if it is used.
const StatementVector & get_statements() const noexcept
Getter for member variable StatementBlock::statements.
std::shared_ptr< ast::Expression > replace_for_name(const std::shared_ptr< ast::Expression > &node) const
if expression we are visiting is Name then return new Integer node
Utility functions for visitors implementation.
void set_rhs(std::shared_ptr< Expression > &&rhs)
Setter for member variable BinaryExpression::rhs (rvalue reference)
Represents specific element of an array variable.
void set_lhs(std::shared_ptr< Expression > &&lhs)
Setter for member variable BinaryExpression::lhs (rvalue reference)
void visit_statement_block(ast::StatementBlock &node) override
visit node of type ast::StatementBlock
Concrete visitor for all AST classes.
void visit_binary_expression(ast::BinaryExpression &node) override
visit node of type ast::BinaryExpression
static std::shared_ptr< ast::ExpressionStatement > unroll_for_loop(const std::shared_ptr< ast::FromStatement > &node)
Unroll given for loop.
void visit_children(visitor::Visitor &v) override
visit children i.e.
std::vector< std::shared_ptr< const ast::Ast > > collect_nodes(const ast::Ast &node, const std::vector< ast::AstNodeType > &types)
traverse node recursively and collect nodes of given types
int value
integer value of index variable
void visit_children(visitor::Visitor &v) override
visit children i.e.
Represents block encapsulating list of statements.
void reset_statement(StatementVector::const_iterator position, Statement *n)
Reset member to statements.
Implement logger based on spdlog library.
@ VERBATIM
type of ast::Verbatim
void visit_indexed_name(ast::IndexedName &node) override
visit node of type ast::IndexedName
static std::shared_ptr< ast::Expression > unwrap(const std::shared_ptr< ast::Expression > &expr)
return underlying expression wrapped by WrappedExpression
std::shared_ptr< Expression > get_lhs() const noexcept
Getter for member variable BinaryExpression::lhs.
Represents binary expression in the NMODL.
Auto generated AST classes declaration.
void set_length(std::shared_ptr< Expression > &&length)
Setter for member variable IndexedName::length (rvalue reference)
IndexRemover(std::string index, int value)