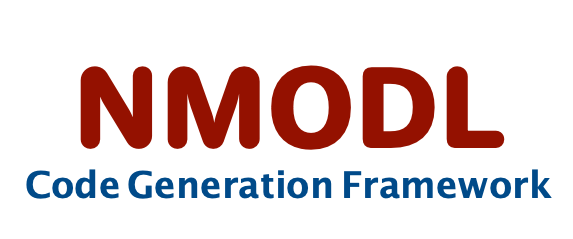 |
User Guide
|
Go to the documentation of this file.
24 solve_method = method ? method->get_value()->eval() :
"";
37 statement_block->emplace_back_statement(e);
51 const auto& lhs = node.
get_lhs();
59 auto name = std::dynamic_pointer_cast<ast::VarName>(lhs)->get_name();
61 if (name->is_prime_name()) {
68 auto expr_statement = std::dynamic_pointer_cast<ast::ExpressionStatement>(
70 const auto bin_expr = std::dynamic_pointer_cast<const ast::BinaryExpression>(
71 expr_statement->get_expression());
72 node.
set_lhs(std::shared_ptr<ast::Expression>(bin_expr->get_lhs()->clone()));
73 node.
set_rhs(std::shared_ptr<ast::Expression>(bin_expr->get_rhs()->clone()));
75 logger->warn(
"NeuronSolveVisitor :: cnexp solver not possible for {}",
83 auto expr_statement = std::dynamic_pointer_cast<ast::ExpressionStatement>(
85 const auto bin_expr = std::dynamic_pointer_cast<const ast::BinaryExpression>(
86 expr_statement->get_expression());
87 node.
set_lhs(std::shared_ptr<ast::Expression>(bin_expr->get_lhs()->clone()));
88 node.
set_rhs(std::shared_ptr<ast::Expression>(bin_expr->get_rhs()->clone()));
94 std::string n = name->get_node_name();
95 auto statement =
create_statement(fmt::format(
"{} = {} + dt * D{}", n, n, n));
99 auto varname =
"D" + name->get_node_name();
102 auto symbol = std::make_shared<symtab::Symbol>(varname,
ModToken());
103 symbol->set_original_name(name->get_node_name());
104 symbol->created_from_state();
108 logger->error(
"NeuronSolveVisitor :: solver method '{}' not supported",
solve_method);
std::string to_nmodl(const ast::Ast &node, const std::set< ast::AstNodeType > &exclude_types)
Given AST node, return the NMODL string representation.
void visit_binary_expression(ast::BinaryExpression &node) override
visit node of type ast::BinaryExpression
std::vector< std::shared_ptr< ast::Statement > > euler_solution_expressions
Implement class to represent a symbol in Symbol Table.
Represents differential equation in DERIVATIVE block.
void visit_solve_block(ast::SolveBlock &node) override
visit node of type ast::SolveBlock
static bool cnexp_possible(const std::string &equation, std::string &solution)
check if given equation can be solved using cnexp method
void visit_diff_eq_expression(ast::DiffEqExpression &node) override
visit node of type ast::DiffEqExpression
static constexpr char CNEXP_METHOD[]
cnexp method in nmodl
std::shared_ptr< Name > get_name() const noexcept
Getter for member variable DerivativeBlock::name.
bool derivative_block
visiting derivative block
encapsulates code generation backend implementations
bool differential_equation
true while visiting differential equation
symtab::SymbolTable * program_symtab
global symbol table
Utility functions for visitors implementation.
void set_rhs(std::shared_ptr< Expression > &&rhs)
Setter for member variable BinaryExpression::rhs (rvalue reference)
std::string derivative_block_name
the derivative name currently being visited
void visit_children(visitor::Visitor &v) override
visit children i.e.
void set_lhs(std::shared_ptr< Expression > &&lhs)
Setter for member variable BinaryExpression::lhs (rvalue reference)
std::string solve_method
method specified in solve block
std::shared_ptr< Name > get_block_name() const noexcept
Getter for member variable SolveBlock::block_name.
void visit_children(visitor::Visitor &v) override
visit children i.e.
std::shared_ptr< StatementBlock > get_statement_block() const noexcept override
Getter for member variable DerivativeBlock::statement_block.
static std::string solve(const std::string &equation, std::string method, bool debug=false)
solve equation using provided method
void visit_derivative_block(ast::DerivativeBlock &node) override
visit node of type ast::DerivativeBlock
std::map< std::string, std::string > solve_blocks
a map holding solve block names and methods
void visit_children(visitor::Visitor &v) override
visit children i.e.
Represents DERIVATIVE block in the NMODL.
std::shared_ptr< Name > get_method() const noexcept
Getter for member variable SolveBlock::method.
void insert(const std::shared_ptr< Symbol > &symbol)
std::shared_ptr< Statement > create_statement(const std::string &code_statement)
Convert given code statement (in string format) to corresponding ast node.
void visit_program(ast::Program &node) override
visit node of type ast::Program
static constexpr char DERIVIMPLICIT_METHOD[]
derivimplicit method in nmodl
Implement logger based on spdlog library.
static constexpr char EULER_METHOD[]
euler method in nmodl
symtab::SymbolTable * get_symbol_table() const override
Return associated symbol table for the current ast node.
Visitor that solves ODEs using old solvers of NEURON
std::shared_ptr< Expression > get_lhs() const noexcept
Getter for member variable BinaryExpression::lhs.
Represents top level AST node for whole NMODL input.
std::shared_ptr< Symbol > lookup(const std::string &name) const
check if symbol with given name exist in the current table (but not in parents)
Represents binary expression in the NMODL.
Represent token returned by scanner.
Auto generated AST classes declaration.