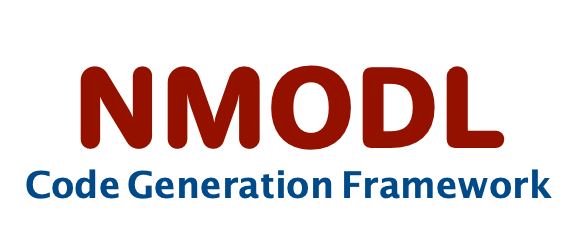 |
User Guide
|
Go to the documentation of this file.
33 const std::vector<ast::AstNodeType> blocks_to_analyze = {
49 auto it = std::find(blocks_to_analyze.begin(), blocks_to_analyze.end(), type);
50 auto node_to_analyze = !(it == blocks_to_analyze.end());
52 return node_to_analyze || solve_procedure;
62 if (symbol && symbol->has_any_property(NmodlType::to_solve)) {
70 std::vector<std::string> result;
72 const NmodlType excluded_var_properties = NmodlType::extern_var
73 | NmodlType::extern_neuron_variable
74 | NmodlType::read_ion_var
75 | NmodlType::write_ion_var
76 | NmodlType::prime_name
77 | NmodlType::nonspecific_cur_var
78 | NmodlType::pointer_var
79 | NmodlType::bbcore_pointer_var
80 | NmodlType::electrode_cur_var
81 | NmodlType::state_var;
83 const NmodlType global_var_properties = NmodlType::range_var
84 | NmodlType::assigned_definition
85 | NmodlType::param_assign;
95 for (
const auto& variable: variables) {
96 if (!variable->has_any_property(excluded_var_properties)) {
97 result.push_back(variable->get_name());
107 logger->warn(
"LocalizeVisitor :: symbol table is not setup, returning");
112 for (
const auto& varname: variables) {
114 std::map<DUState, std::vector<std::shared_ptr<ast::Node>>> block_usage;
116 logger->debug(
"LocalizeVisitor: Checking DU chains for {}", varname);
119 for (
const auto& block: blocks) {
122 const auto& usages = v.
analyze(*block, varname);
123 auto result = usages.
eval();
124 block_usage[result].push_back(block);
125 logger->debug(
"\tDU chain in block {} is {}",
126 block->get_node_type_name(),
134 if (it == block_usage.end()) {
135 logger->debug(
"LocalizeVisitor : localized variable {}", varname);
140 for (
auto& block: block_usage[state]) {
141 auto block_ptr =
dynamic_cast<ast::Block*
>(block.get());
146 if (symbol->is_array()) {
154 symbol->mark_localized();
157 const auto& symtab = statement_block->get_symbol_table();
158 auto new_symbol = std::make_shared<Symbol>(varname, variable);
159 new_symbol->add_property(NmodlType::local_var);
160 new_symbol->mark_created();
161 symtab->insert(new_symbol);
@ DERIVATIVE_BLOCK
type of ast::DerivativeBlock
Base class for all AST node.
Visitor to transform global variable usage to local
@ FOR_NETCON
type of ast::ForNetcon
std::vector< std::string > variables_to_optimize() const
@ INITIAL_BLOCK
type of ast::InitialBlock
encapsulates code generation backend implementations
@ CONSTRUCTOR_BLOCK
type of ast::ConstructorBlock
DUState eval() const
return "effective" usage of a variable
std::vector< std::shared_ptr< Symbol > > get_variables_with_properties(syminfo::NmodlType properties, bool all=false) const
get variables with properties
virtual bool is_procedure_block() const noexcept
Check if the ast node is an instance of ast::ProcedureBlock.
Implement various classes to represent various Symbol properties.
@ DESTRUCTOR_BLOCK
type of ast::DestructorBlock
Visitor to return Def-Use chain for a given variable in the block/node
@ D
global variable is defined
DUChain analyze(const ast::Ast &node, const std::string &name)
compute def-use chain for a variable within the node
@ U
global variable is used
bool ignore_verbatim
ignore verbatim blocks while localizing
Base class for all block scoped nodes.
@ AFTER_BLOCK
type of ast::AfterBlock
bool is_solve_procedure(const ast::Node &node) const
@ NON_LINEAR_BLOCK
type of ast::NonLinearBlock
void visit_program(const ast::Program &node) override
visit node of type ast::Program
Visitor to return Def-Use chain for a given variable in the block/node
bool node_for_def_use_analysis(const ast::Node &node) const
NmodlType
NMODL variable properties.
const NodeVector & get_blocks() const noexcept
Getter for member variable Program::blocks.
Implement logger based on spdlog library.
LocalVar * add_local_variable(StatementBlock &node, Identifier *varname)
symtab::SymbolTable * get_symbol_table() const override
Return associated symbol table for the current ast node.
@ CD
global or local variable is conditionally defined
@ DISCRETE_BLOCK
type of ast::DiscreteBlock
virtual std::shared_ptr< StatementBlock > get_statement_block() const
Return associated statement block for the AST node.
Represents top level AST node for whole NMODL input.
@ BEFORE_BLOCK
type of ast::BeforeBlock
symtab::SymbolTable * program_symtab
std::shared_ptr< Symbol > lookup(const std::string &name) const
check if symbol with given name exist in the current table (but not in parents)
@ BREAKPOINT_BLOCK
type of ast::BreakpointBlock
@ BA_BLOCK
type of ast::BABlock
@ NET_RECEIVE_BLOCK
type of ast::NetReceiveBlock
virtual std::string get_node_name() const
Return name of of the node.
Auto generated AST classes declaration.
AstNodeType get_node_type() const noexcept override
Return type (ast::AstNodeType) of ast node.
@ LINEAR_BLOCK
type of ast::LinearBlock