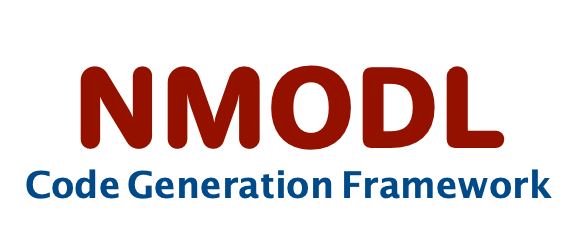 |
User Guide
|
Go to the documentation of this file.
42 return collect_nodes(node, {AstNodeType::IF_STATEMENT, AstNodeType::VERBATIM}).empty();
50 std::vector<std::string> statements;
55 const auto& names_in_block =
collect_nodes(node, {AstNodeType::NAME});
57 for (
const auto& name: names_in_block) {
58 auto varname = name->get_node_name();
60 used_names_in_block.insert(varname);
67 auto it =
i_name.find(lhs_str);
69 std::string i_name_str = it->second;
73 static_cast<std::ptrdiff_t
>(
78 auto [dIdV, exception_message] = analytic_diff(expressions, used_names_in_block);
79 if (!exception_message.empty()) {
80 logger->warn(
"SympyConductance :: python exception: {}", exception_message);
84 "SympyConductance :: analytic differentiation of ionic current "
87 std::string g_var = dIdV;
92 std::map<std::string, int> var_map;
100 std::string statement_str = g_var;
101 statement_str.append(
" = ").append(dIdV);
102 statements.insert(statements.begin(), statement_str);
103 logger->debug(
"SympyConductance :: Adding BREAKPOINT statement: {}",
106 std::string statement_str =
"CONDUCTANCE " + g_var;
107 if (!i_name_str.empty()) {
108 statement_str +=
" USEION " + i_name_str;
110 statements.push_back(statement_str);
111 logger->debug(
"SympyConductance :: Adding BREAKPOINT statement: {}", statement_str);
126 std::dynamic_pointer_cast<ast::VarName>(node.
get_lhs())->get_name()->get_node_name();
138 logger->debug(
"SympyConductance :: Found NONSPECIFIC_CURRENT statement");
139 for (
const auto& write_name:
140 std::dynamic_pointer_cast<const ast::Nonspecific>(ns_curr_ast)->get_currents()) {
141 const std::string& curr_write = write_name->get_node_name();
142 logger->debug(
"SympyConductance :: -> Adding non-specific current write name: {}",
158 const auto& ion = std::dynamic_pointer_cast<const ast::Useion>(useion_ast);
159 const std::string& ion_name = ion->get_node_name();
162 logger->debug(
"SympyConductance :: -> Ignoring ion current name: {}", ion_name);
164 for (
const auto& w: ion->get_writelist()) {
165 std::string ion_write = w->get_node_name();
167 "SympyConductance :: -> Adding ion write name: {} for ion current name: {}",
170 i_name[ion_write] = ion_name;
179 logger->debug(
"SympyConductance :: Found existing CONDUCTANCE statement: {}",
181 if (
const auto& ion = node.
get_ion()) {
182 logger->debug(
"SympyConductance :: -> Ignoring ion current name: {}", ion->get_node_name());
183 i_ignore.insert(ion->get_node_name());
185 logger->debug(
"SympyConductance :: -> Ignoring all non-specific currents");
193 logger->warn(
"SympyConductance :: Unsafe to insert CONDUCTANCE statement");
199 for (
const auto& localvar: symtab->get_variables_with_properties(NmodlType::local_var)) {
200 all_vars.insert(localvar->get_name());
214 if (!new_statements.empty()) {
219 auto insertion_point = brkpnt_statements.begin();
220 while ((*insertion_point)->is_local_list_statement()) {
223 for (
const auto& statement_str: new_statements) {
224 insertion_point = brkpnt_statements.insert(insertion_point,
234 const auto& program = node;
BinaryOp
enum Type for binary operators in NMODL
static EmbeddedPythonLoader & get_instance()
Construct (if not already done) and get the only instance of this class.
std::string to_nmodl(const ast::Ast &node, const std::set< ast::AstNodeType > &exclude_types)
Given AST node, return the NMODL string representation.
Implement class to represent a symbol in Symbol Table.
Base class for all Abstract Syntax Tree node types.
std::shared_ptr< Name > get_ion() const noexcept
Getter for member variable ConductanceHint::ion.
std::vector< std::string > generate_statement_strings(ast::BreakpointBlock &node)
Represents CONDUCTANCE statement in NMODL.
string_map i_name
map between current write names and ion names
bool under_breakpoint_block
true while visiting breakpoint block
encapsulates code generation backend implementations
string_set all_vars
set of all variables for SymPy
void visit_binary_expression(ast::BinaryExpression &node) override
visit node of type ast::BinaryExpression
std::vector< std::string > ordered_binary_exprs
list in order of binary expressions in breakpoint
static std::string to_nmodl_for_sympy(const ast::Ast &node)
void visit_program(ast::Program &node) override
visit node of type ast::Program
std::shared_ptr< StatementBlock > get_statement_block() const noexcept override
Getter for member variable BreakpointBlock::statement_block.
std::vector< std::shared_ptr< const ast::Ast > > use_ion_nodes
use ion ast nodes
AstNodeType
Enum type for every AST node type.
void visit_breakpoint_block(ast::BreakpointBlock &node) override
visit node of type ast::BreakpointBlock
void lookup_nonspecific_statements()
std::set< std::string > string_set
Utility functions for visitors implementation.
Visitor for generating CONDUCTANCE statements for ions
Represents a BREAKPOINT block in NMODL.
std::map< std::string, std::size_t > binary_expr_index
map from lhs of binary expression to index of expression in above vector
const pybind_wrap_api & api()
Get a pointer to the pybind_wrap_api struct.
void visit_children(visitor::Visitor &v) override
visit children i.e.
@ UNIT_DEF
type of ast::UnitDef
const BinaryOperator & get_op() const noexcept
Getter for member variable BinaryExpression::op.
std::shared_ptr< Statement > create_statement(const std::string &code_statement)
Convert given code statement (in string format) to corresponding ast node.
std::vector< std::shared_ptr< const ast::Ast > > collect_nodes(const ast::Ast &node, const std::vector< ast::AstNodeType > &types)
traverse node recursively and collect nodes of given types
std::string get_new_name(const std::string &name, const std::string &suffix, std::map< std::string, int > &variables)
Return new name variable by appending _suffix_COUNT where COUNT is number of times the given variable...
NmodlType
NMODL variable properties.
decltype(&call_analytic_diff) analytic_diff
void visit_children(visitor::Visitor &v) override
visit children i.e.
static bool conductance_statement_possible(const ast::BreakpointBlock &node)
Analyse breakpoint block to check if it is safe to insert CONDUCTANCE statements.
BinaryOp get_value() const noexcept
Getter for member variable BinaryOperator::value.
Implement logger based on spdlog library.
std::vector< std::string > ordered_binary_exprs_lhs
ditto but for LHS of expression only
bool NONSPECIFIC_CONDUCTANCE_ALREADY_EXISTS
LocalVar * add_local_variable(StatementBlock &node, Identifier *varname)
std::shared_ptr< Expression > get_lhs() const noexcept
Getter for member variable BinaryExpression::lhs.
Represents top level AST node for whole NMODL input.
string_set i_ignore
set of currents to ignore
std::vector< std::shared_ptr< const ast::Ast > > nonspecific_nodes
non specific currents
void visit_conductance_hint(ast::ConductanceHint &node) override
visit node of type ast::ConductanceHint
Represents binary expression in the NMODL.
void lookup_useion_statements()
std::set< std::string > get_global_vars(const Program &node)
Return set of strings with the names of all global variables.
Auto generated AST classes declaration.