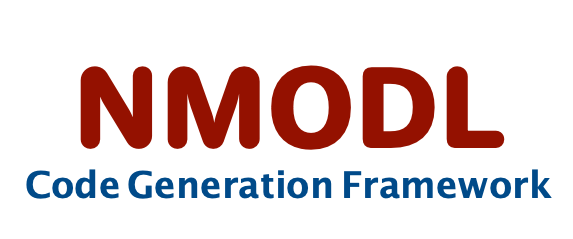 |
User Guide
|
Go to the documentation of this file.
24 #include <string_view>
41 using printer::CodePrinter;
329 std::vector<std::string>
const& ) {}
523 const std::
string& concentration,
626 const std::
string& name,
627 bool use_instance) const override;
638 bool use_instance = true) const override;
649 std::
string get_variable_name(const std::
string& name,
bool use_instance = true) const override;
762 const std::
string& function_name = "") override;
1099 template <typename T>
1131 template <typename T>
1133 const std::
string& name) {
1139 const auto& params = node.get_parameters();
1140 for (
const auto& param: params) {
1141 internal_params.emplace_back(
"", type,
"", param.get()->get_node_name());
1145 const char* return_type =
"int";
1146 if (node.is_function_block()) {
1152 printer->fmt_text(
"inline {} {}({})",
virtual void print_procedure(const ast::ProcedureBlock &node) override
Print NMODL procedure in target backend code.
virtual void print_device_method_annotation()
Print the backend specific device method annotation.
void print_coreneuron_includes()
Print includes from coreneuron.
virtual std::string net_receive_buffering_declaration()
Generate the target backend code for the net_receive_buffering function delcaration.
virtual bool nrn_cur_reduction_loop_required()
Check if reduction block in nrn_cur required.
virtual void print_backend_namespace_start()
Prints the start of namespace for the backend-specific code.
void print_net_move_call(const ast::FunctionCall &node) override
Print call to net_move.
void print_mechanism_range_var_structure(bool print_initializers) override
Print the structure that wraps all range and int variables required for the NMODL.
virtual void print_net_send_buf_count_update_to_host() const
Print the code to update NetSendBuffer_t count from device to host.
Visitor for printing C++ code compatible with legacy api of CoreNEURON
void print_function_prototypes() override
Print function and procedures prototype declaration.
void print_functors_definitions()
Go through the map of EigenNewtonSolverBlock s and their corresponding functor names and print the fu...
void print_namespace_stop() override
Prints the end of the coreneuron namespace.
Helper to represent information about index/int variables.
void print_v_unused() const override
Set v_unused (voltage) for NRN_PRCELLSTATE feature.
virtual void print_ion_variable()
Print the ion variable struct.
void print_derivimplicit_kernel(const ast::Block &block)
Print derivative kernel when derivimplicit method is used.
virtual void print_device_stream_wait() const
Print the code to synchronise/wait on stream specific to NrnThread.
virtual void print_instance_struct_delete_from_device()
Delete the instance struct from the device.
virtual void print_net_send_buf_count_update_to_device() const
Print the code to update NetSendBuffer_t count from host to device.
codegen::CodegenInfo info
All ast information for code generation.
void print_functor_definition(const ast::EigenNewtonSolverBlock &node)
Based on the EigenNewtonSolverBlock passed print the definition needed for its functor.
std::string global_variable_name(const SymbolType &symbol, bool use_instance=true) const override
Determine the variable name for a global variable given its symbol.
void visit_eigen_linear_solver_block(const ast::EigenLinearSolverBlock &node) override
visit node of type ast::EigenLinearSolverBlock
virtual void print_newtonspace_transfer_to_device() const
Print code block to transfer newtonspace structure to device.
int thread_data_index
thread_data_index indicates number of threads being allocated.
void print_namespace_end() override
Print end of namespaces.
void print_net_send_call(const ast::FunctionCall &node) override
Print call to net_send.
virtual void print_net_receive_loop_begin()
Print the code for the main net_receive loop.
virtual void print_kernel_data_present_annotation_block_begin()
Print accelerator annotations indicating data presence on device.
void print_backend_info() override
Print top file header printed in generated code.
void print_g_unused() const override
Set g_unused (conductance) for NRN_PRCELLSTATE feature.
void print_common_getters()
Print common getters.
std::string int_variable_name(const IndexVariableInfo &symbol, const std::string &name, bool use_instance) const override
Determine the name of an int variable given its symbol.
std::tuple< bool, int > check_if_var_is_array(const std::string &name)
Check if the given name exist in the symbol.
void print_initial_block(const ast::InitialBlock *node)
Print initial block statements.
void print_net_receive_kernel()
Print net_receive kernel function definition.
void print_thread_getters()
Print the getter method for thread variables and ids.
std::string simulator_name() override
Name of the simulator the code was generated for.
void print_net_send_buffering()
Print kernel for buffering net_send events.
encapsulates code generation backend implementations
int num_thread_objects() const noexcept
Determine the number of threads to allocate.
virtual void print_fast_imem_calculation() override
Print fast membrane current calculation code.
void print_function_declaration(const T &node, const std::string &name)
Print prototype declarations of functions or procedures.
virtual void print_dt_update_to_device() const
Print the code to update dt from host to device.
virtual void print_net_init_acc_serial_annotation_block_end()
Print accelerator kernels end annotation for net_init kernel.
void print_net_init()
Print initial block in the net receive block.
void print_net_event_call(const ast::FunctionCall &node) override
Print call to net_event.
void print_nrn_destructor() override
Print nrn_destructor function definition.
void print_sdlists_init(bool print_initializers) override
Implement classes for representing symbol table at block and file scope.
void print_headers_include() override
Print all includes.
void print_send_event_move()
Print send event move block used in net receive as well as watch.
void print_table_replacement_function(const ast::Block &node)
Print replacement function for function or procedure using table.
virtual void print_atomic_reduction_pragma() override
Print atomic update pragma for reduction statements.
virtual void print_instance_struct_copy_to_device()
Transfer the instance struct to the device.
void print_global_variables_for_hoc() override
Print byte arrays that register scalar and vector variables for hoc interface.
parser::NmodlParser::symbol_type SymbolType
void print_function_or_procedure(const ast::Block &node, const std::string &name) override
Print nmodl function or procedure (common code)
void print_net_receive_common_code(const ast::Block &node, bool need_mech_inst=true)
Print the common code section for net receive related methods.
void print_nrn_current(const ast::BreakpointBlock &node) override
Print the nrn_current kernel.
const char * default_float_data_type() const noexcept
Default data type for floating point elements.
std::string conc_write_statement(const std::string &ion_name, const std::string &concentration, int index) override
Generate Function call statement for nrn_wrote_conc.
void print_first_pointer_var_index_getter()
Print the getter method for index position of first pointer variable.
void print_setup_range_variable()
Print the function that initialize range variable with different data type.
std::string internal_method_arguments() override
Arguments for functions that are defined and used internally.
virtual void print_instance_struct_transfer_routine_declarations()
Print declarations of the functions used by print_instance_struct_copy_to_device and print_instance_s...
std::string nrn_thread_arguments() const override
Arguments for "_threadargs_" macro in neuron implementation.
Represent newton solver solution block based on Eigen.
void print_net_receive_arg_size_getter()
Print the getter method for getting number of arguments for net_receive.
bool is_functor_const(const ast::StatementBlock &variable_block, const ast::StatementBlock &functor_block)
Checks whether the functor_block generated by sympy solver modifies any variable outside its scope.
Visitor for printing C++ code compatible with legacy api of CoreNEURON
Base class for all block scoped nodes.
Represents a INITIAL block in the NMODL.
virtual void print_nrn_cur_matrix_shadow_update()
Print the update to matrix elements with/without shadow vectors.
std::string register_mechanism_arguments() const override
Arguments for register_mech or point_register_mech function.
virtual void print_net_init_acc_serial_annotation_block_begin()
Print accelerator kernels begin annotation for net_init kernel.
virtual void print_deriv_advance_flag_transfer_to_device() const
Print the code to copy derivative advance flag to device.
void print_first_random_var_index_getter()
Print the getter method for index position of first RANDOM variable.
Represent WATCH statement in NMODL.
virtual void print_eigen_linear_solver(const std::string &float_type, int N)
virtual void print_before_after_block(const ast::Block *node, size_t block_id)
Print NMODL before / after block in target backend code.
Represents a BREAKPOINT block in NMODL.
Helper class for printing C/C++ code.
void print_ion_var_structure()
Print structure of ion variables used for local copies.
ParamVector internal_method_parameters() override
Parameters for internally defined functions.
int position_of_int_var(const std::string &name) const override
Determine the position in the data array for a given int variable.
virtual void print_channel_iteration_block_parallel_hint(BlockType type, const ast::Block *block)
Print pragma annotations for channel iterations.
virtual bool is_constant_variable(const std::string &name) const
Check if variable is qualified as constant.
void print_function_procedure_helper(const ast::Block &node) override
Common helper function to help printing function or procedure blocks.
const char * external_method_parameters(bool table=false) noexcept override
Parameters for functions in generated code that are called back from external code.
void visit_for_netcon(const ast::ForNetcon &node) override
visit node of type ast::ForNetcon
virtual void print_compute_functions() override
Print all compute functions for every backend.
void print_nrn_cur_conductance_kernel(const ast::BreakpointBlock &node) override
Print the nrn_cur kernel with NMODL conductance keyword provisions.
virtual void print_memory_allocation_routine() const
Print memory allocation routine.
void print_check_table_thread_function()
Print check_table functions.
void print_namespace_begin() override
Print start of namespaces.
virtual std::string backend_name() const override
Name of the code generation backend.
virtual void print_rhs_d_shadow_variables()
Print the setup method for setting matrix shadow vectors.
std::string process_verbatim_text(std::string const &text) override
Process a verbatim block for possible variable renaming.
void print_net_receive()
Print net_receive function definition.
bool vectorize
true if mod file is vectorizable (which should be always true for coreneuron) But there are some bloc...
bool optimize_ion_variable_copies() const override
Check if ion variable copies should be avoided.
void print_function(const ast::FunctionBlock &node) override
Print NMODL function in target backend code.
void print_top_verbatim_blocks()
Print top level (global scope) verbatim blocks.
Various types to store code generation specific information.
Represent linear solver solution block based on Eigen.
void print_mechanism_global_var_structure(bool print_initializers) override
Print the structure that wraps all global variables used in the NMODL.
void visit_eigen_newton_solver_block(const ast::EigenNewtonSolverBlock &node) override
visit node of type ast::EigenNewtonSolverBlock
void print_nrn_constructor() override
Print nrn_constructor function definition.
virtual void print_kernel_data_present_annotation_block_end()
Print matching block end of accelerator annotations for data presence on device.
virtual void print_instance_struct_transfer_routines(std::vector< std::string > const &)
Print the definitions of the functions used by print_instance_struct_copy_to_device and print_instanc...
void print_nrn_alloc() override
Print nrn_alloc function definition.
virtual void print_nrn_cur_matrix_shadow_reduction()
Print the reduction to matrix elements from shadow vectors.
void print_memb_list_getter()
Print the getter method for returning membrane list from NrnThread.
static std::string get_parameter_str(const ParamVector ¶ms)
Generate the string representing the procedure parameter declaration.
void print_watch_check()
Print watch activate function.
const char * external_method_arguments() noexcept override
Arguments for external functions called from generated code.
void print_instance_variable_setup()
Print the function that initialize instance structure.
void print_nrn_init(bool skip_init_check=true)
Print the nrn_init function definition.
Represents block encapsulating list of statements.
std::string get_variable_name(const std::string &name, bool use_instance=true) const override
Determine variable name in the structure of mechanism properties.
void print_namespace_start() override
Prints the start of the coreneuron namespace.
virtual void print_abort_routine() const
Print backend specific abort routine.
void print_table_check_function(const ast::Block &node)
Print check_function() for functions or procedure using table.
void print_nrn_cur() override
Print nrn_cur / current update function definition.
std::string get_range_var_float_type(const SymbolType &symbol)
Returns floating point type for given range variable symbol.
virtual void print_net_send_buffering_cnt_update() const
Print the code related to the update of NetSendBuffer_t cnt.
void print_thread_memory_callbacks()
Print thread related memory allocation and deallocation callbacks.
Represent a callback to NEURON's derivimplicit solver.
void print_net_receive_buffering(bool need_mech_inst=true)
Print kernel for buffering net_receive events.
virtual void print_global_function_common_code(BlockType type, const std::string &function_name="") override
Print common code for global functions like nrn_init, nrn_cur and nrn_state.
CodegenCppVisitor(std::string mod_filename, const std::string &output_dir, std::string float_type, const bool optimize_ionvar_copies, size_t blame_line=0)
Constructs the C++ code generator visitor.
std::string float_type
Data type of floating point variables.
Implement logger based on spdlog library.
bool enable_variable_name_lookup
Variable name should be converted to instance name (but not for function arguments)
BlockType
Helper to represent various block types.
void print_mechanism_register() override
Print the mechanism registration function.
virtual void print_global_method_annotation()
Print backend specific global method annotation.
void print_nrn_cur_kernel(const ast::BreakpointBlock &node) override
Print main body of nrn_cur function.
std::string nrn_thread_internal_arguments() override
Arguments for "_threadargs_" macro in neuron implementation.
virtual void print_device_atomic_capture_annotation() const
Print pragma annotation for increase and capture of variable in automatic way.
void visit_derivimplicit_callback(const ast::DerivimplicitCallback &node) override
visit node of type ast::DerivimplicitCallback
Visitor for printing C++ code compatible with legacy api of CoreNEURON
virtual void print_get_memb_list()
Print the target backend code for defining and checking a local Memb_list variable.
void print_num_variable_getter()
Print the getter methods for float and integer variables count.
void print_standard_includes() override
Print standard C/C++ includes.
virtual void print_net_receive_loop_end()
Print the code for closing the main net_receive loop.
void print_mech_type_getter()
Print the getter method for returning mechtype.
std::string float_variable_name(const SymbolType &symbol, bool use_instance) const override
Determine the name of a float variable given its symbol.
virtual void print_global_variable_device_update_annotation()
Print the pragma annotation to update global variables from host to the device.
std::unique_ptr< CodePrinter > printer
Code printer object for target (C++)
void print_function_tables(const ast::FunctionTableBlock &node)
Print NMODL function_table in target backend code.
std::string method_name(const std::string &name) const
Constructs the name of a function or procedure.
virtual void visit_watch_statement(const ast::WatchStatement &node) override
visit node of type ast::WatchStatement
void print_nrn_cur_non_conductance_kernel() override
Print the nrn_cur kernel without NMODL conductance keyword provisions.
virtual void print_backend_includes()
Print backend specific includes (none needed for C++ backend)
std::string process_verbatim_token(const std::string &token)
Process a token in a verbatim block for possible variable renaming.
void print_nrn_state() override
Print nrn_state / state update function definition.
virtual void print_codegen_routines() override
Print entry point to code generation.
void print_watch_activate()
Print watch activate function.
virtual void print_net_send_buffering_grow()
Print statement that grows NetSendBuffering_t structure if needed.
virtual void print_backend_namespace_stop()
Prints the end of namespace for the backend-specific code.
virtual void print_net_send_buf_update_to_host() const
Print the code to update NetSendBuffer_t from device to host.
void print_data_structures(bool print_initializers) override
Print all classes.
std::string replace_if_verbatim_variable(std::string name)
Replace commonly used verbatim variables.
virtual void print_ion_var_constructor(const std::vector< std::string > &members)
Print constructor of ion variables.
int position_of_float_var(const std::string &name) const override
Determine the position in the data array for a given float variable.
std::vector< std::tuple< std::string, std::string, std::string, std::string > > ParamVector
A vector of parameters represented by a 4-tuple of strings:
Concrete visitor for all AST classes.