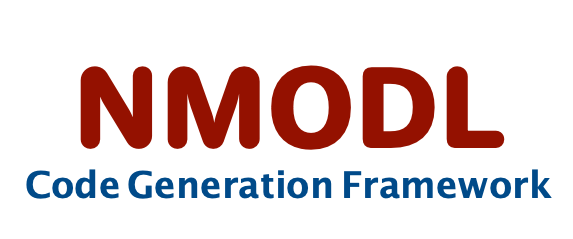 |
User Guide
|
Go to the documentation of this file.
24 #include <string_view>
113 const std::shared_ptr<symtab::Symbol>
symbol;
199 const std::string& output_dir,
202 size_t blame_line = 0)
227 std::ostream& stream,
230 size_t blame_line = 0)
250 using ParamVector = std::vector<std::tuple<std::string, std::string, std::string, std::string>>;
464 return std::string(
"mechanism_info");
499 template <
typename T>
752 bool open_brace =
true,
753 bool close_brace =
true);
835 template <
typename T>
837 const std::string& separator,
838 const std::string& prefix =
"");
911 return "\"" + text +
"\"";
931 return std::make_shared<symtab::Symbol>(name,
ModToken());
942 const std::string& concentration,
1003 const std::string& name,
1004 bool use_instance)
const = 0;
1015 bool use_instance =
true)
const = 0;
1027 bool use_instance =
true)
const = 0;
1121 const std::string& function_name =
"") = 0;
1349 template <
typename T>
1351 const std::string& separator,
1352 const std::string& prefix) {
1353 for (
auto iter = elements.begin(); iter != elements.end(); iter++) {
1355 (*iter)->accept(*
this);
virtual void print_nrn_constructor()=0
Print nrn_constructor function definition.
bool ion_variable_struct_required() const
Check if a structure for ion variables is required.
bool is_last(Iter iter, const Cont &cont)
Check if the iterator is pointing to last element in the container.
static bool need_semicolon(const ast::Statement &node)
Check if a semicolon is required at the end of given statement.
void update_index_semantics()
populate all index semantics needed for registration with coreneuron
virtual std::string register_mechanism_arguments() const =0
Arguments for register_mech or point_register_mech function.
virtual void print_g_unused() const =0
Set g_unused (conductance) for NRN_PRCELLSTATE feature.
bool is_index
if this is pure index (e.g.
Represents a C code block.
void visit_name(const ast::Name &node) override
visit node of type ast::Name
bool has_parameter_of_name(const T &node, const std::string &name)
Check if function or procedure node has parameter with given name.
int int_variables_size() const
Number of integer variables in the model.
virtual void print_sdlists_init(bool print_initializers)=0
void visit_if_statement(const ast::IfStatement &node) override
visit node of type ast::IfStatement
std::vector< ShadowUseStatement > ion_write_statements(BlockType type)
For a given output block type, return statements for writing back ion variables.
void visit_else_statement(const ast::ElseStatement &node) override
visit node of type ast::ElseStatement
Helper to represent information about index/int variables.
bool breakpoint_exist() const noexcept
Check if breakpoint node exist.
Concrete constant visitor for all AST classes.
virtual void print_nrn_current(const ast::BreakpointBlock &node)=0
Print the nrn_current kernel.
@ Destructor
destructor block
static constexpr char DEFAULT_LOCAL_VAR_TYPE[]
default local variable type
const std::shared_ptr< symtab::Symbol > symbol
symbol for the variable
codegen::CodegenInfo info
All ast information for code generation.
virtual void print_atomic_reduction_pragma()=0
Print atomic update pragma for reduction statements.
std::string global_struct_instance() const
Name of the (host-only) global instance of global_struct
Represents a double variable.
static std::pair< std::string, std::string > write_ion_variable_name(const std::string &name)
Return ion variable name and corresponding ion write variable name.
virtual int position_of_float_var(const std::string &name) const =0
Determine the position in the data array for a given float variable.
const char * operator_for_d() const noexcept
Operator for diagonal vector update (matrix update)
bool optimize_ionvar_copies
Flag to indicate if visitor should avoid ion variable copies.
bool net_receive_exist() const noexcept
Check if net_receive node exist.
std::shared_ptr< symtab::Symbol > SymbolType
virtual bool optimize_ion_variable_copies() const =0
Check if ion variable copies should be avoided.
std::string nmodl_version() const noexcept
Return Nmodl language version.
bool printing_top_verbatim_blocks
true if currently printing top level verbatim blocks
int float_variables_size() const
Number of float variables in the model.
bool is_constant
if the variable is qualified as constant (this is property of IndexVariable)
void visit_from_statement(const ast::FromStatement &node) override
visit node of type ast::FromStatement
virtual void print_global_var_struct_assertions() const
Print static assertions about the global variable struct.
bool printing_net_init
true if currently initial block of net_receive being printed
void print_mechanism_info()
Print backend code for byte array that has mechanism information (to be registered with NEURON/CoreNE...
virtual void print_global_function_common_code(BlockType type, const std::string &function_name="")=0
Print common code for global functions like nrn_init, nrn_cur and nrn_state.
virtual void print_standard_includes()=0
Print standard C/C++ includes.
void visit_prime_name(const ast::PrimeName &node) override
visit node of type ast::PrimeName
virtual void print_nrn_cur_non_conductance_kernel()=0
Print the nrn_cur kernel without NMODL conductance keyword provisions.
virtual void print_function_procedure_helper(const ast::Block &node)=0
Common helper function to help printing function or procedure blocks.
encapsulates code generation backend implementations
@ index
index / int variables
const std::string & float_data_type() const noexcept
Data type for floating point elements specified on command line.
virtual void print_function(const ast::FunctionBlock &node)=0
Print NMODL function in target backend code.
Implement classes for representing symbol table at block and file scope.
virtual std::string nrn_thread_arguments() const =0
Arguments for "_threadargs_" macro in neuron implementation.
bool range_variable_setup_required() const noexcept
Check if setup_range_variable function is required.
Represent MUTEXLOCK statement in NMODL.
void print_statement_block(const ast::StatementBlock &node, bool open_brace=true, bool close_brace=true)
Print any statement block in nmodl with option to (not) print braces.
virtual std::string global_variable_name(const SymbolType &symbol, bool use_instance=true) const =0
Determine the variable name for a global variable given its symbol.
void visit_binary_expression(const ast::BinaryExpression &node) override
visit node of type ast::BinaryExpression
Represents an integer variable.
@ BlockTypeEnd
fake ending block type for loops on the enums. Keep it at the end
std::vector< SymbolType > get_float_variables() const
Determine all float variables required during code generation.
virtual void print_net_move_call(const ast::FunctionCall &node)=0
Print call to net_move.
const char * default_int_data_type() const noexcept
Default data type for integer (offset) elements.
std::string mod_suffix
name of the suffix
parser::NmodlParser::symbol_type SymbolType
Represent information collected from AST for code generation.
void visit_paren_expression(const ast::ParenExpression &node) override
visit node of type ast::ParenExpression
const char * default_float_data_type() const noexcept
Default data type for floating point elements.
bool net_receive_buffering_required() const noexcept
Check if net receive/send buffering kernels required.
std::string update_if_ion_variable_name(const std::string &name) const
Determine the updated name if the ion variable has been optimized.
virtual void print_nrn_cur_conductance_kernel(const ast::BreakpointBlock &node)=0
Print the nrn_cur kernel with NMODL conductance keyword provisions.
void visit_mutex_unlock(const ast::MutexUnlock &node) override
visit node of type ast::MutexUnlock
bool is_integer
if this is an integer (e.g.
virtual std::string int_variable_name(const IndexVariableInfo &symbol, const std::string &name, bool use_instance) const =0
Determine the name of an int variable given its symbol.
void visit_unit(const ast::Unit &node) override
visit node of type ast::Unit
void visit_update_dt(const ast::UpdateDt &node) override
visit node of type ast::UpdateDt
void print_vector_elements(const std::vector< T > &elements, const std::string &separator, const std::string &prefix="")
Print the items in a vector as a list.
IndexVariableInfo(std::shared_ptr< symtab::Symbol > symbol, bool is_vdata=false, bool is_index=false, bool is_integer=false)
static constexpr char DEFAULT_FLOAT_TYPE[]
default float variable type
std::vector< IndexVariableInfo > get_int_variables()
Determine all int variables required during code generation.
virtual std::string nrn_thread_internal_arguments()=0
Arguments for "_threadargs_" macro in neuron implementation.
void visit_function_call(const ast::FunctionCall &node) override
visit node of type ast::FunctionCall
Base class for all block scoped nodes.
virtual int position_of_int_var(const std::string &name) const =0
Determine the position in the data array for a given int variable.
symtab::SymbolTable * program_symtab
Symbol table for the program.
@ Equation
breakpoint block
MemberType
Helper to represent various variables types.
bool is_net_move(const std::string &name) const noexcept
Checks if given function name is net_move.
Represents a float variable.
virtual void print_mechanism_global_var_structure(bool print_initializers)=0
Print the structure that wraps all global variables used in the NMODL.
Represents a BREAKPOINT block in NMODL.
virtual void print_compute_functions()=0
Print all compute functions for every backend.
std::vector< SymbolType > codegen_global_variables
All global variables for the model.
Represents specific element of an array variable.
virtual std::string simulator_name()=0
Name of the simulator the code was generated for.
void setup(const ast::Program &node)
Setup the target backend code generator.
void visit_unary_operator(const ast::UnaryOperator &node) override
visit node of type ast::UnaryOperator
void visit_mutex_lock(const ast::MutexLock &node) override
visit node of type ast::MutexLock
virtual void print_net_event_call(const ast::FunctionCall &node)=0
Print call to net_event.
void visit_boolean(const ast::Boolean &node) override
visit node of type ast::Boolean
bool nrn_cur_required() const noexcept
Check if nrn_cur function is required.
std::vector< std::string > ion_read_statements_optimized(BlockType type) const
For a given output block type, return minimal statements for all read ion variables.
virtual void print_net_send_call(const ast::FunctionCall &node)=0
Print call to net_send.
@ Constructor
constructor block
virtual void print_codegen_routines()=0
Print entry point to code generation.
Represent MUTEXUNLOCK statement in NMODL.
virtual std::string conc_write_statement(const std::string &ion_name, const std::string &concentration, int index)=0
Generate Function call statement for nrn_wrote_conc.
Helper class for printing C/C++ code.
void visit_double(const ast::Double &node) override
visit node of type ast::Double
bool net_send_buffer_required() const noexcept
Check if net_send_buffer is required.
static constexpr char NMODL_VERSION[]
nmodl language version
std::vector< IndexVariableInfo > codegen_int_variables
All int variables for the model.
void visit_float(const ast::Float &node) override
visit node of type ast::Float
void visit_string(const ast::String &node) override
visit node of type ast::String
@ NetReceive
net_receive block
void visit_solution_expression(const ast::SolutionExpression &node) override
visit node of type ast::SolutionExpression
const char * local_var_type() const noexcept
Data type for the local variables.
virtual void print_backend_info()=0
Print top file header printed in generated code.
virtual void print_global_var_struct_decl()
Instantiate global var instance.
std::string get_channel_info_var_name() const noexcept
Name of channel info variable.
virtual void print_namespace_begin()=0
Print start of namespaces.
void visit_var_name(const ast::VarName &node) override
void print_nmodl_constants()
Print the nmodl constants used in backend code.
Represents a prime variable (for ODE)
static std::pair< std::string, std::string > read_ion_variable_name(const std::string &name)
Return ion variable name and corresponding ion read variable name.
virtual void print_namespace_end()=0
Print end of namespaces.
Various types to store code generation specific information.
Represents a boolean variable.
static constexpr char DEFAULT_INTEGER_TYPE[]
default integer variable type
Represent symbol table for a NMODL block.
void visit_integer(const ast::Integer &node) override
visit node of type ast::Integer
bool printing_net_receive
true if currently net_receive block being printed
virtual std::string process_verbatim_text(std::string const &text)=0
Process a verbatim block for possible variable renaming.
Statement to indicate a change in timestep in a given block.
virtual void print_global_variables_for_hoc()=0
Print byte arrays that register scalar and vector variables for hoc interface.
std::string format_float_string(const std::string &value)
Convert a given float value to its string representation.
static std::string get_parameter_str(const ParamVector ¶ms)
Generate the string representing the procedure parameter declaration.
virtual void print_nrn_cur()=0
Print nrn_cur / current update function definition.
bool is_net_send(const std::string &name) const noexcept
Checks if given function name is net_send.
Represents block encapsulating list of statements.
virtual void print_nrn_cur_kernel(const ast::BreakpointBlock &node)=0
Print main body of nrn_cur function.
bool net_receive_required() const noexcept
Check if net_receive function is required.
virtual std::string float_variable_name(const SymbolType &symbol, bool use_instance) const =0
Determine the name of a float variable given its symbol.
virtual std::string internal_method_arguments()=0
Arguments for functions that are defined and used internally.
std::string global_struct() const
Name of structure that wraps global variables.
virtual const char * external_method_parameters(bool table=false) noexcept=0
Parameters for functions in generated code that are called back from external code.
bool electrode_current
if electrode current specified
void rename_function_arguments()
Rename function/procedure arguments that conflict with default arguments.
virtual ParamVector internal_method_parameters()=0
Parameters for internally defined functions.
virtual void print_nrn_destructor()=0
Print nrn_destructor function definition.
void visit_local_list_statement(const ast::LocalListStatement &node) override
visit node of type ast::LocalListStatement
void visit_protect_statement(const ast::ProtectStatement &node) override
visit node of type ast::ProtectStatement
Represents ion write statement during code generation.
CodegenCppVisitor(std::string mod_filename, std::ostream &stream, std::string float_type, const bool optimize_ionvar_copies, size_t blame_line=0)
Constructs the C++ code generator visitor.
virtual void print_mechanism_range_var_structure(bool print_initializers)=0
Print the structure that wraps all range and int variables required for the NMODL.
SymbolType make_symbol(const std::string &name) const
Creates a temporary symbol.
CodegenCppVisitor(std::string mod_filename, const std::string &output_dir, std::string float_type, const bool optimize_ionvar_copies, size_t blame_line=0)
Constructs the C++ code generator visitor.
std::string float_type
Data type of floating point variables.
Operator used in ast::BinaryExpression.
bool defined_method(const std::string &name) const
Check if given method is defined in this model.
static constexpr char NET_MOVE_METHOD[]
net_move function call in nmodl
Implement logger based on spdlog library.
std::string node_data_struct() const
Name of structure that wraps node variables.
bool is_vdata
if variable resides in vdata field of NrnThread typically true for bbcore pointer
virtual void print_function_call(const ast::FunctionCall &node)
Print call to internal or external function.
Represent solution of a block in the AST.
std::string process_shadow_update_statement(const ShadowUseStatement &statement, BlockType type)
Process shadow update statement.
bool enable_variable_name_lookup
Variable name should be converted to instance name (but not for function arguments)
BlockType
Helper to represent various block types.
int current_watch_statement
Index of watch statement being printed.
static constexpr char NET_EVENT_METHOD[]
net_event function call in nmodl
virtual void print_fast_imem_calculation()=0
Print fast membrane current calculation code.
virtual void print_mechanism_register()=0
Print the mechanism registration function.
std::string breakpoint_current(std::string current) const
Determine the variable name for the "current" used in breakpoint block taking into account intermedia...
void visit_binary_operator(const ast::BinaryOperator &node) override
visit node of type ast::BinaryOperator
void visit_else_if_statement(const ast::ElseIfStatement &node) override
visit node of type ast::ElseIfStatement
Visitor for printing C++ code compatible with legacy api of CoreNEURON
virtual void print_namespace_start()=0
Prints the start of the simulator namespace.
std::string instance_struct() const
Name of structure that wraps range variables.
std::string compute_method_name(BlockType type) const
virtual const char * external_method_arguments() noexcept=0
Arguments for external functions called from generated code.
void visit_program(const ast::Program &program) override
Main and only member function to call after creating an instance of this class.
std::string mod_filename
Name of mod file (without .mod suffix)
virtual std::string get_variable_name(const std::string &name, bool use_instance=true) const =0
Determine variable name in the structure of mechanism properties.
Represents top level AST node for whole NMODL input.
void visit_indexed_name(const ast::IndexedName &node) override
visit node of type ast::IndexedName
const char * operator_for_rhs() const noexcept
Operator for rhs vector update (matrix update)
void print_prcellstate_macros() const
Print declaration of macro NRN_PRCELLSTATE for debugging.
std::unique_ptr< CodePrinter > printer
Code printer object for target (C++)
virtual void print_namespace_stop()=0
Prints the end of the simulator namespace.
bool nrn_state_required() const noexcept
Check if nrn_state function is required.
std::string add_escape_quote(const std::string &text) const
Add quotes to string to be output.
bool internal_method_call_encountered
true if internal method call was encountered while processing verbatim block
virtual void print_v_unused() const =0
Set v_unused (voltage) for NRN_PRCELLSTATE feature.
std::string method_name(const std::string &name) const
Constructs the name of a function or procedure.
void visit_verbatim(const ast::Verbatim &node) override
visit node of type ast::Verbatim
bool is_net_event(const std::string &name) const noexcept
Checks if given function name is net_event.
Represents binary expression in the NMODL.
virtual void print_headers_include()=0
Print all includes.
std::vector< SymbolType > codegen_float_variables
All float variables for the model.
Helper class for printing C/C++ code.
static bool statement_to_skip(const ast::Statement &node)
Check if given statement should be skipped during code generation.
std::vector< std::string > ion_read_statements(BlockType type) const
For a given output block type, return statements for all read ion variables.
virtual void print_procedure(const ast::ProcedureBlock &node)=0
Print NMODL procedure in target backend code.
@ BeforeAfter
before / after block
virtual void print_data_structures(bool print_initializers)=0
Print all classes.
std::string format_double_string(const std::string &value)
Convert a given double value to its string representation.
virtual void print_function_or_procedure(const ast::Block &node, const std::string &name)=0
Print nmodl function or procedure (common code)
Represent token returned by scanner.
virtual void print_nrn_state()=0
Print nrn_state / state update function definition.
virtual std::string backend_name() const =0
Name of the code generation backend.
virtual void print_function_prototypes()=0
Print function and procedures prototype declaration.
static constexpr char NET_SEND_METHOD[]
net_send function call in nmodl
virtual void print_nrn_alloc()=0
Print nrn_alloc function definition.
void visit_while_statement(const ast::WhileStatement &node) override
visit node of type ast::WhileStatement
@ range
range / double variables
std::vector< std::tuple< std::string, std::string, std::string, std::string > > ParamVector
A vector of parameters represented by a 4-tuple of strings:
void visit_statement_block(const ast::StatementBlock &node) override
Concrete visitor for all AST classes.