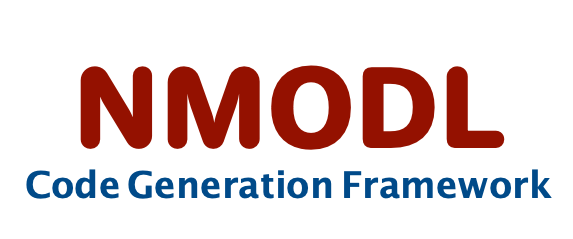 |
User Guide
|
Go to the documentation of this file.
19 using printer::JSONPrinter;
23 using utils::PerfStat;
35 bool assign_op =
false;
98 throw std::logic_error(
"Binary operator not handled in perf visitor");
119 const auto& values = perf.
values();
120 assert(keys.size() == values.size());
122 for (
size_t i = 0; i < keys.size(); i++) {
123 printer->add_node(values[i], keys[i]);
144 if (symtab ==
nullptr) {
145 throw std::runtime_error(
"Perfvisitor : symbol table not setup for " +
149 auto name = symtab->name();
158 perf.
title =
"Performance Statistics of " + name;
170 var_set.second.clear();
182 }
else if (name ==
"log") {
184 }
else if (name ==
"pow") {
190 auto method_property = NmodlType::procedure_block | NmodlType::function_block;
191 if (symbol !=
nullptr && symbol->has_any_property(method_property)) {
233 NmodlType property = NmodlType::range_var | NmodlType::assigned_definition |
234 NmodlType::state_var;
237 for (
auto& variable: variables) {
238 if (!variable->has_any_property(NmodlType::global_var)) {
240 if (variable->has_any_property(NmodlType::param_assign)) {
243 if (variable->has_any_status(Status::localized)) {
250 property = NmodlType::state_var;
255 property = NmodlType::pointer_var | NmodlType::bbcore_pointer_var;
260 property = NmodlType::random_var;
268 property = NmodlType::global_var | NmodlType::param_assign | NmodlType::bbcore_pointer_var |
269 NmodlType::pointer_var;
272 for (
auto& variable: variables) {
273 auto is_global = variable->has_any_property(NmodlType::global_var);
274 property = NmodlType::range_var | NmodlType::assigned_definition;
275 if (!variable->has_any_property(property) || is_global) {
277 if (variable->has_any_property(NmodlType::param_assign)) {
280 if (variable->has_any_status(Status::localized)) {
290 stream <<
"#VARIABLES :: ";
304 printer->push_block(
"MemoryInfo");
306 printer->push_block(
"Instance");
322 printer->push_block(
"Pointer");
336 printer->push_block(
"BlockPerf");
340 std::string title =
"Total Performance Statistics";
440 throw std::runtime_error(
"Perfvisitor : symbol table not setup for " +
482 throw std::logic_error(
"Unary operator not handled in perf visitor");
497 auto is_method = symbol->has_any_property(NmodlType::extern_method | NmodlType::function_block);
502 is_method = symbol->has_any_property(NmodlType::derivative_block | NmodlType::extern_method);
511 bool is_local =
false;
513 auto properties = NmodlType::local_var | NmodlType::argument | NmodlType::function_block;
514 if (symbol->has_any_property(properties)) {
521 bool is_constant =
false;
522 auto properties = NmodlType::param_assign;
523 if (symbol->has_any_property(properties)) {
std::string get_node_name() const override
Return name of the node.
void visit_children(visitor::Visitor &v) override
visit children i.e.
void visit_before_block(const ast::BeforeBlock &node) override
visit node of type ast::BeforeBlock
Represents a BEFORE block in NMODL.
bool under_function_call
whether function call is being visited
Represents a block to be executed before or after another block.
std::map< std::string, std::set< std::string > > var_usage
map of variables to count unique read-writes
void visit_unary_expression(const ast::UnaryExpression &node) override
visit node of type ast::UnaryExpression
void visit_children(visitor::Visitor &v) override
visit children i.e.
int n_ext_func_call
could be external math funcs
Visitor for measuring performance related information
Base class for all Abstract Syntax Tree node types.
void visit_prime_name(const ast::PrimeName &node) override
prime name derived from identifier and hence need to be handled here
int num_state_variables
count of state variables
std::vector< std::string > values() const
std::stack< utils::PerfStat > children_blocks_perf
performance of current all childrens
std::string global_memr_key
void visit_children(visitor::Visitor &v) override
visit children i.e.
static bool is_local_variable(const std::shared_ptr< symtab::Symbol > &symbol)
void visit_name(const ast::Name &node) override
every variable used is of type name, update counters
std::string global_memw_key
encapsulates code generation backend implementations
void visit_after_block(const ast::AfterBlock &node) override
visit node of type ast::AfterBlock
int num_constant_instance_variables
subset of instance variables which are constant
void visit_net_receive_block(const ast::NetReceiveBlock &node) override
visit node of type ast::NetReceiveBlock
static std::vector< std::string > keys()
std::vector< std::shared_ptr< Symbol > > get_variables_with_properties(syminfo::NmodlType properties, bool all=false) const
get variables with properties
void update_memory_ops(const std::string &name)
Find symbol in closest scope (up to parent) and update read/write count.
std::string const_memr_key
keys used in map to track var usage
void visit_binary_expression(const ast::BinaryExpression &node) override
count math operations from all binary expressions
Status
state during various compiler passes
void visit_non_linear_block(const ast::NonLinearBlock &node) override
visit node of type ast::NonLinearBlock
void visit_statement_block(const ast::StatementBlock &node) override
Blocks like function can have multiple statement blocks and blocks like net receive has nested initia...
symtab::SymbolTable * current_symtab
symbol table of current block being visited
void visit_function_call(const ast::FunctionCall &node) override
count function calls and "most useful" or "commonly used" math functions
int num_instance_variables
count of per channel instance variables
void visit_procedure_block(const ast::ProcedureBlock &node) override
visit node of type ast::ProcedureBlock
Helper class for printing AST in JSON form.
std::shared_ptr< Expression > get_rhs() const noexcept
Getter for member variable BinaryExpression::rhs.
int n_global_read
expensive : typically access to dynamically allocated memory
void visit_children(visitor::Visitor &v) override
visit children i.e.
void visit_children(visitor::Visitor &v) override
visit children i.e.
Represents a INITIAL block in the NMODL.
int n_unique_constant_write
void visit_destructor_block(const ast::DestructorBlock &node) override
visit node of type ast::DestructorBlock
bool visiting_lhs_expression
true while visiting lhs of binary expression (to count write operations)
void compact_json(bool flag)
std::unique_ptr< printer::JSONPrinter > printer
to print to json file
Helper class for printing AST in JSON form.
UnaryOp get_value() const noexcept
Getter for member variable UnaryOperator::value.
Represents a BREAKPOINT block in NMODL.
virtual std::string get_node_type_name() const =0
Return type (ast::AstNodeType) of ast node as std::string.
void visit_program(const ast::Program &node) override
visit node of type ast::Program
virtual symtab::SymbolTable * get_symbol_table() const
Return associated symbol table for the AST node.
bool is_method(const std::string &name)
Check if given name is an integration method in NMODL.
Represents a DESTRUCTOR block in the NMODL.
void visit_children(visitor::Visitor &v) override
visit children i.e.
bool start_measurement
whether to measure performance for current block
Represents a CONSTRUCTOR block in the NMODL.
symtab::SymbolTable * get_symbol_table() const override
Return associated symbol table for the current ast node.
void visit_solve_block(const ast::SolveBlock &node) override
solve is not a statement but could have associated block and hence could/should not be skipped comple...
void print(std::stringstream &stream) const
int n_local_read
cheap : typically local variables in mod file means registers
std::string to_string(const T &obj)
void visit_children(visitor::Visitor &v) override
visit children i.e.
static bool is_constant_variable(const std::shared_ptr< symtab::Symbol > &symbol)
int n_constant_read
could be optimized : access to variables that could be read-only in this case write counts are typica...
void visit_children(visitor::Visitor &v) override
visit children i.e.
const UnaryOperator & get_op() const noexcept
Getter for member variable UnaryExpression::op.
bool under_net_receive_block
whether net receive block is being visited
const BinaryOperator & get_op() const noexcept
Getter for member variable BinaryExpression::op.
int num_localized_global_variables
subset of global variables which are localized
Represents a prime variable (for ODE)
Represents DERIVATIVE block in the NMODL.
void visit_for_netcon(const ast::ForNetcon &node) override
visit node of type ast::ForNetcon
void visit_discrete_block(const ast::DiscreteBlock &node) override
visit node of type ast::DiscreteBlock
void visit_constructor_block(const ast::ConstructorBlock &node) override
visit node of type ast::ConstructorBlock
void visit_if_statement(const ast::IfStatement &node) override
visit node of type ast::IfStatement
Represents block encapsulating list of statements.
void measure_performance(const ast::Ast &node)
Helper function used by all ast nodes : visit all children recursively and performance stats get adde...
NmodlType
NMODL variable properties.
void visit_else_if_statement(const ast::ElseIfStatement &node) override
visit node of type ast::ElseIfStatement
int num_pointer_variables
count of pointer / bbcorepointer variables
std::stack< utils::PerfStat > blocks_perf
performance stats of all blocks being visited in recursive chain
Represents LINEAR block in the NMODL.
int num_global_variables
count of global variables
int num_localized_instance_variables
subset of instance variables which are localized
std::string get_node_name() const override
Return name of the node.
void visit_children(visitor::Visitor &v) override
visit children i.e.
std::string get_node_name() const override
Return name of the node.
BinaryOp get_value() const noexcept
Getter for member variable BinaryOperator::value.
Represents NONLINEAR block in the NMODL.
bool under_solve_block
whether solve block is being visited
std::stringstream stream
if not json, all goes to string
int n_int_func_call
mod functions (before/after inlining)
utils::PerfStat current_block_perf
performance of current block
void visit_ba_block(const ast::BABlock &node) override
visit node of type ast::BABlock
symtab::SymbolTable * get_symbol_table() const override
Return associated symbol table for the current ast node.
void visit_linear_block(const ast::LinearBlock &node) override
visit node of type ast::LinearBlock
bool symbol_to_skip(const std::shared_ptr< symtab::Symbol > &symbol) const
Certain statements / symbols needs extra check while measuring read/write operations.
void visit_breakpoint_block(const ast::BreakpointBlock &node) override
visit node of type ast::BreakpointBlock
std::shared_ptr< Expression > get_lhs() const noexcept
Getter for member variable BinaryExpression::lhs.
utils::PerfStat total_perf
total performance of mod file
std::string get_node_type_name() const noexcept override
Return type (ast::AstNodeType) of ast node as std::string.
Helper class to collect performance statistics.
Represents top level AST node for whole NMODL input.
void visit_function_table_block(const ast::FunctionTableBlock &node) override
visit node of type ast::FunctionTableBlock
int num_constant_global_variables
subset of global variables which are constant
std::string const_memw_key
Represents a AFTER block in NMODL.
int num_random_variables
count of RANDOM variables
void visit_kinetic_block(const ast::KineticBlock &node) override
visit node of type ast::KineticBlock
int n_unique_global_write
void print_memory_usage()
virtual void visit_children(visitor::Visitor &v)=0
Visit children i.e.
void add_perf_to_printer(const utils::PerfStat &perf) const
add performance stats to json printer
Represents binary expression in the NMODL.
void visit_initial_block(const ast::InitialBlock &node) override
skip initial block under net_receive block
std::string title
name for pretty-printing
virtual bool is_derivative_block() const noexcept
Check if the ast node is an instance of ast::DerivativeBlock.
int n_exp
expensive functions : commonly used functions in mod files
void visit_function_block(const ast::FunctionBlock &node) override
visit node of type ast::FunctionBlock
Auto generated AST classes declaration.
int n_unique_constant_read
std::shared_ptr< Symbol > lookup_in_scope(const std::string &name) const
check if symbol with given name exist in the current table (including all parents)
void visit_derivative_block(const ast::DerivativeBlock &node) override
visit node of type ast::DerivativeBlock
int n_add
basic ops (<= 1 cycle)