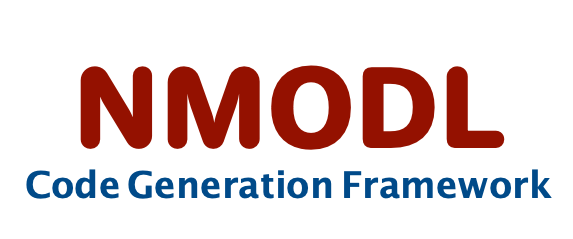 |
User Guide
|
Go to the documentation of this file.
93 std::unique_ptr<printer::JSONPrinter>
printer;
139 bool symbol_to_skip(
const std::shared_ptr<symtab::Symbol>& symbol)
const;
252 void print(std::ostream& ss)
const {
void visit_conductance_hint(const ast::ConductanceHint &) override
certain constructs needs to be excluded from usage counting and hence need to provide empty implement...
void visit_before_block(const ast::BeforeBlock &node) override
visit node of type ast::BeforeBlock
Represents a BEFORE block in NMODL.
bool under_function_call
whether function call is being visited
int get_const_instance_variable_count() const noexcept
Represents a block to be executed before or after another block.
std::map< std::string, std::set< std::string > > var_usage
map of variables to count unique read-writes
void visit_unary_expression(const ast::UnaryExpression &node) override
visit node of type ast::UnaryExpression
void visit_useion(const ast::Useion &) override
visit node of type ast::Useion
int get_global_variable_count() const noexcept
Concrete constant visitor for all AST classes.
Base class for all Abstract Syntax Tree node types.
void visit_prime_name(const ast::PrimeName &node) override
prime name derived from identifier and hence need to be handled here
int num_state_variables
count of state variables
std::stack< utils::PerfStat > children_blocks_perf
performance of current all childrens
std::string global_memr_key
int get_instance_variable_count() const noexcept
static bool is_local_variable(const std::shared_ptr< symtab::Symbol > &symbol)
void visit_name(const ast::Name &node) override
every variable used is of type name, update counters
std::string global_memw_key
Represents CONDUCTANCE statement in NMODL.
void print(std::ostream &ss) const
encapsulates code generation backend implementations
void visit_after_block(const ast::AfterBlock &node) override
visit node of type ast::AfterBlock
void visit_valence(const ast::Valence &) override
visit node of type ast::Valence
int num_constant_instance_variables
subset of instance variables which are constant
void visit_net_receive_block(const ast::NetReceiveBlock &node) override
visit node of type ast::NetReceiveBlock
void update_memory_ops(const std::string &name)
Find symbol in closest scope (up to parent) and update read/write count.
std::string const_memr_key
keys used in map to track var usage
void visit_binary_expression(const ast::BinaryExpression &node) override
count math operations from all binary expressions
Represents SUFFIX statement in NMODL.
void visit_non_linear_block(const ast::NonLinearBlock &node) override
visit node of type ast::NonLinearBlock
void visit_statement_block(const ast::StatementBlock &node) override
Blocks like function can have multiple statement blocks and blocks like net receive has nested initia...
symtab::SymbolTable * current_symtab
symbol table of current block being visited
void visit_function_call(const ast::FunctionCall &node) override
count function calls and "most useful" or "commonly used" math functions
int num_instance_variables
count of per channel instance variables
void visit_procedure_block(const ast::ProcedureBlock &node) override
visit node of type ast::ProcedureBlock
Represents a INITIAL block in the NMODL.
Visitor for measuring performance related information
void visit_destructor_block(const ast::DestructorBlock &node) override
visit node of type ast::DestructorBlock
bool visiting_lhs_expression
true while visiting lhs of binary expression (to count write operations)
void compact_json(bool flag)
std::unique_ptr< printer::JSONPrinter > printer
to print to json file
Forward references of symbols defined in namespace nmodl::printer.
Represents a BREAKPOINT block in NMODL.
void visit_program(const ast::Program &node) override
visit node of type ast::Program
Represents a DESTRUCTOR block in the NMODL.
void visit_suffix(const ast::Suffix &) override
visit node of type ast::Suffix
bool start_measurement
whether to measure performance for current block
Represents a CONSTRUCTOR block in the NMODL.
Represents USEION statement in NMODL.
void visit_solve_block(const ast::SolveBlock &node) override
solve is not a statement but could have associated block and hence could/should not be skipped comple...
static bool is_constant_variable(const std::shared_ptr< symtab::Symbol > &symbol)
bool under_net_receive_block
whether net receive block is being visited
int num_localized_global_variables
subset of global variables which are localized
Represents a prime variable (for ODE)
Represents DERIVATIVE block in the NMODL.
Represent symbol table for a NMODL block.
void visit_for_netcon(const ast::ForNetcon &node) override
visit node of type ast::ForNetcon
void visit_discrete_block(const ast::DiscreteBlock &node) override
visit node of type ast::DiscreteBlock
void visit_constructor_block(const ast::ConstructorBlock &node) override
visit node of type ast::ConstructorBlock
void visit_if_statement(const ast::IfStatement &node) override
visit node of type ast::IfStatement
Represents block encapsulating list of statements.
void measure_performance(const ast::Ast &node)
Helper function used by all ast nodes : visit all children recursively and performance stats get adde...
void visit_else_if_statement(const ast::ElseIfStatement &node) override
visit node of type ast::ElseIfStatement
int num_pointer_variables
count of pointer / bbcorepointer variables
std::stack< utils::PerfStat > blocks_perf
performance stats of all blocks being visited in recursive chain
Represents LINEAR block in the NMODL.
int num_global_variables
count of global variables
int num_localized_instance_variables
subset of instance variables which are localized
Represents NONLINEAR block in the NMODL.
bool under_solve_block
whether solve block is being visited
std::stringstream stream
if not json, all goes to string
utils::PerfStat current_block_perf
performance of current block
void visit_ba_block(const ast::BABlock &node) override
visit node of type ast::BABlock
void visit_linear_block(const ast::LinearBlock &node) override
visit node of type ast::LinearBlock
bool symbol_to_skip(const std::shared_ptr< symtab::Symbol > &symbol) const
Certain statements / symbols needs extra check while measuring read/write operations.
void visit_breakpoint_block(const ast::BreakpointBlock &node) override
visit node of type ast::BreakpointBlock
utils::PerfStat total_perf
total performance of mod file
const utils::PerfStat & get_total_perfstat() const noexcept
Helper class to collect performance statistics.
int get_const_global_variable_count() const noexcept
Represents top level AST node for whole NMODL input.
void visit_function_table_block(const ast::FunctionTableBlock &node) override
visit node of type ast::FunctionTableBlock
void visit_local_list_statement(const ast::LocalListStatement &) override
visit node of type ast::LocalListStatement
int num_constant_global_variables
subset of global variables which are constant
std::string const_memw_key
Represents a AFTER block in NMODL.
int num_random_variables
count of RANDOM variables
int get_state_variable_count() const noexcept
void visit_kinetic_block(const ast::KineticBlock &node) override
visit node of type ast::KineticBlock
void print_memory_usage()
void add_perf_to_printer(const utils::PerfStat &perf) const
add performance stats to json printer
Represents binary expression in the NMODL.
Implement class for performance statistics.
void visit_initial_block(const ast::InitialBlock &node) override
skip initial block under net_receive block
Forward declarations of symbols in namespace nmodl::symtab.
void visit_function_block(const ast::FunctionBlock &node) override
visit node of type ast::FunctionBlock
void visit_derivative_block(const ast::DerivativeBlock &node) override
visit node of type ast::DerivativeBlock
Concrete visitor for all AST classes.