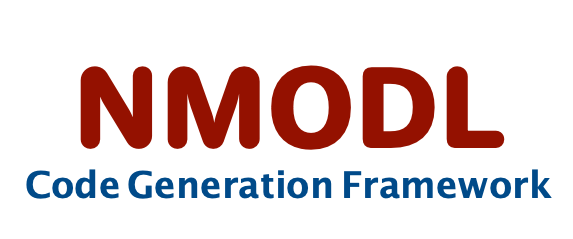 |
User Guide
|
Go to the documentation of this file.
19 using printer::JSONPrinter;
36 return "CONDITIONAL_BLOCK";
48 throw std::runtime_error(
"Unhandled DUState?");
71 std::ostringstream stream;
76 for (
const auto& instance:
chain) {
77 instance.print(printer);
92 const auto& child_state = chain.eval(variable_type);
136 bool block_with_none =
false;
139 auto child_state = chain.eval(variable_type);
142 result = child_state;
146 block_with_none =
true;
153 result = child_state;
179 for (
auto& inst:
chain) {
207 if (symbol ==
nullptr || symbol->is_external_variable()) {
216 if (symtab !=
nullptr) {
232 if (is_outer_binary_expression) {
237 node.
get_rhs()->visit_children(*
this);
241 node.
get_lhs()->visit_children(*
this);
244 if (is_outer_binary_expression) {
263 block->accept(*
this);
318 const std::string& variable =
to_nmodl(node);
323 const std::string& variable =
to_nmodl(node);
334 if (!length->is_integer()) {
337 if (name == variable_name_prefix) {
339 const std::string& text =
to_nmodl(node);
340 nmodl::logger->info(
"index used to access variable is not known : {} ", text);
344 auto index = std::dynamic_pointer_cast<ast::Integer>(length);
371 assert(symbol !=
nullptr);
373 const auto properties = NmodlType::local_var | NmodlType::argument;
374 const auto is_local = symbol->has_any_property(properties);
400 std::ostringstream fullname;
427 auto global_symbol_properties = NmodlType::global_var | NmodlType::range_var |
428 NmodlType::assigned_definition;
432 if (global_symbol !=
nullptr && global_symbol->has_any_property(global_symbol_properties)) {
std::string get_node_name() const override
Return name of the node.
std::shared_ptr< Expression > get_length() const noexcept
Getter for member variable IndexedName::length.
bool unsupported_node
indicate that there is unsupported construct encountered
void visit_function_call(const ast::FunctionCall &node) override
Nothing to do if called function is not defined or it's external but if there is a function call for ...
Base class for all AST node.
Represents a C code block.
std::string variable_name
variable for which to construct def-use chain
DUVariableType
Variable type processed by DefUseAnalyzeVisitor.
std::string to_nmodl(const ast::Ast &node, const std::set< ast::AstNodeType > &exclude_types)
Given AST node, return the NMODL string representation.
DUState eval(DUVariableType variable_type) const
analyze all children and return "effective" usage
void print(printer::JSONPrinter &printer) const
DUInstance to JSON string.
DUState
Represent a state in Def-Use chain.
void visit_indexed_name(const ast::IndexedName &node) override
visit node of type ast::IndexedName
Base class for all Abstract Syntax Tree node types.
DUVariableType variable_type
variable type (Local or Global)
std::shared_ptr< Ast > get_shared_ptr() override
Get std::shared_ptr from this pointer of the current ast node.
void update_defuse_chain(const std::string &name)
Update the Def-Use chain for given variable.
void visit_lin_equation(const ast::LinEquation &node) override
visit node of type ast::LinEquation
void visit_conductance_hint(const ast::ConductanceHint &node) override
statements / nodes that should not be used for def-use chain analysis
std::string get_node_name() const override
Return name of the node.
void visit_children(visitor::Visitor &v) override
visit children i.e.
Represent CONSERVE statement in NMODL.
Represents CONDUCTANCE statement in NMODL.
encapsulates code generation backend implementations
void start_new_chain(DUState state)
void visit_unsupported_node(const ast::Node &node)
@ LD
local variable is defined
DUState eval() const
return "effective" usage of a variable
void visit_from_statement(const ast::FromStatement &node) override
visit node of type ast::FromStatement
DUVariableType variable_type
type of variable
bool ignore_verbatim
ignore verbatim blocks
std::shared_ptr< Expression > get_rhs() const noexcept
Getter for member variable BinaryExpression::rhs.
@ D
global variable is defined
symtab::SymbolTable * global_symtab
symbol table containing global variables
DUChain analyze(const ast::Ast &node, const std::string &name)
compute def-use chain for a variable within the node
@ U
global variable is used
DUState conditional_block_eval(DUVariableType variable_type) const
evaluate global usage i.e. with [D,U] states of children
const ElseIfStatementVector & get_elseifs() const noexcept
Getter for member variable IfStatement::elseifs.
void add_node(std::string value, const std::string &key="name")
Add node to json (typically basic type)
std::shared_ptr< Expression > get_condition() const noexcept
Getter for member variable IfStatement::condition.
Helper class for printing AST in JSON form.
void visit_with_new_chain(const ast::Node &node, DUState state)
virtual std::string get_node_type_name() const =0
Return type (ast::AstNodeType) of ast node as std::string.
DUState sub_block_eval(DUVariableType variable_type) const
if, elseif and else evaluation
void visit_conserve(const ast::Conserve &node) override
visit node of type ast::Conserve
void compact_json(bool flag)
print json in compact mode
Represents specific element of an array variable.
void visit_statement_block(const ast::StatementBlock &node) override
visit node of type ast::StatementBlock
std::ostream & operator<<(std::ostream &os, DUState state)
void push_block(const std::string &value, const std::string &key="name")
Add new json object (typically start of new block) name here is type of new block encountered.
virtual void visit_children(visitor::Visitor &v) override
visit children i.e.
void pop_block()
We finished processing a block, add processed block to it's parent block.
symtab::SymbolTable * get_symbol_table() const override
Return associated symbol table for the current ast node.
void process_variable(const std::string &name)
std::vector< DUInstance > * current_chain
def-use chain currently being constructed
@ NONE
variable is not used
void visit_verbatim(const ast::Verbatim &node) override
We are not analyzing verbatim blocks yet and hence if there is a verbatim block we assume there is va...
std::shared_ptr< const ast::BinaryExpression > current_binary_expression
const BinaryOperator & get_op() const noexcept
Getter for member variable BinaryExpression::op.
std::string to_string(bool compact=true) const
return json representation
std::stack< symtab::SymbolTable * > symtab_stack
symbol tables in call hierarchy
std::string to_string(DUState state)
DUState to string conversion for pretty-printing.
@ CONDITIONAL_BLOCK
conditional block
void flush()
Dump json object to stream (typically at the end) nspaces is number of spaces used for indentation.
void visit_argument(const ast::Argument &node) override
visit node of type ast::Argument
void visit_non_lin_equation(const ast::NonLinEquation &node) override
visit node of type ast::NonLinEquation
std::vector< DUInstance > children
usage of variable in case of if like statements
Visitor to return Def-Use chain for a given variable in the block/node
bool visiting_lhs
starting visiting lhs of assignment statement
std::shared_ptr< StatementBlock > get_statement_block() const noexcept override
Getter for member variable IfStatement::statement_block.
Represents block encapsulating list of statements.
DUState state
state of the usage
NmodlType
NMODL variable properties.
Represents an argument to functions and procedures.
void visit_if_statement(const ast::IfStatement &node) override
visit node of type ast::IfStatement
void visit_local_list_statement(const ast::LocalListStatement &node) override
visit node of type ast::LocalListStatement
@ LU
local variable is used
void visit_children(visitor::Visitor &v) override
visit children i.e.
BinaryOp get_value() const noexcept
Getter for member variable BinaryOperator::value.
Implement logger based on spdlog library.
std::vector< DUInstance > chain
def-use chain for a variable
void visit_name(const ast::Name &node) override
visit node of type ast::Name
symtab::SymbolTable * current_symtab
symbol table for current statement block (or of parent block if doesn't have) should be initialized b...
std::shared_ptr< ElseStatement > get_elses() const noexcept
Getter for member variable IfStatement::elses.
void visit_var_name(const ast::VarName &node) override
visit node of type ast::VarName
std::string name
name of the node
@ CD
global or local variable is conditionally defined
std::shared_ptr< Expression > get_lhs() const noexcept
Getter for member variable BinaryExpression::lhs.
void visit_reaction_statement(const ast::ReactionStatement &node) override
unsupported statements : we aren't sure how to handle this "yet" and hence variables used in any of t...
virtual void visit_children(visitor::Visitor &v)=0
Visit children i.e.
Represents binary expression in the NMODL.
Def-Use chain for an AST node.
void visit_binary_expression(const ast::BinaryExpression &node) override
Nmodl grammar doesn't allow assignment operator on rhs (e.g.
Represent use of a variable in the statement.
Auto generated AST classes declaration.
std::shared_ptr< Symbol > lookup_in_scope(const std::string &name) const
check if symbol with given name exist in the current table (including all parents)