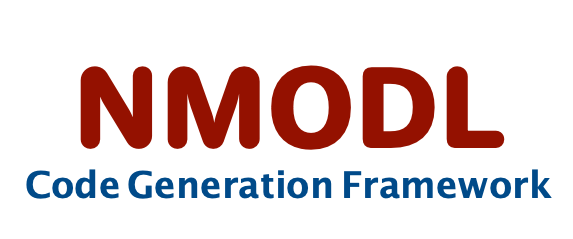 |
User Guide
|
bool unsupported_node
indicate that there is unsupported construct encountered
void visit_function_call(const ast::FunctionCall &node) override
Nothing to do if called function is not defined or it's external but if there is a function call for ...
DefUseAnalyzeVisitor(symtab::SymbolTable &symtab)
Base class for all AST node.
Represents a C code block.
std::string variable_name
variable for which to construct def-use chain
DUVariableType
Variable type processed by DefUseAnalyzeVisitor.
DUState eval(DUVariableType variable_type) const
analyze all children and return "effective" usage
void print(printer::JSONPrinter &printer) const
DUInstance to JSON string.
DUState
Represent a state in Def-Use chain.
Concrete constant visitor for all AST classes.
void visit_indexed_name(const ast::IndexedName &node) override
visit node of type ast::IndexedName
Base class for all Abstract Syntax Tree node types.
DUInstance(DUState state, const std::shared_ptr< const ast::BinaryExpression > binary_expression)
DUVariableType variable_type
variable type (Local or Global)
void update_defuse_chain(const std::string &name)
Update the Def-Use chain for given variable.
void visit_lin_equation(const ast::LinEquation &node) override
visit node of type ast::LinEquation
void visit_conductance_hint(const ast::ConductanceHint &node) override
statements / nodes that should not be used for def-use chain analysis
Represent CONSERVE statement in NMODL.
Represents CONDUCTANCE statement in NMODL.
encapsulates code generation backend implementations
void start_new_chain(DUState state)
void visit_unsupported_node(const ast::Node &node)
@ LD
local variable is defined
DefUseAnalyzeVisitor()=delete
DUState eval() const
return "effective" usage of a variable
void visit_from_statement(const ast::FromStatement &node) override
visit node of type ast::FromStatement
DUVariableType variable_type
type of variable
Visitor to return Def-Use chain for a given variable in the block/node
bool ignore_verbatim
ignore verbatim blocks
Helper class for printing AST in JSON form.
@ D
global variable is defined
symtab::SymbolTable * global_symtab
symbol table containing global variables
DUChain analyze(const ast::Ast &node, const std::string &name)
compute def-use chain for a variable within the node
@ U
global variable is used
DUState conditional_block_eval(DUVariableType variable_type) const
evaluate global usage i.e. with [D,U] states of children
Utility functions for visitors implementation.
Helper class for printing AST in JSON form.
void visit_with_new_chain(const ast::Node &node, DUState state)
DUState sub_block_eval(DUVariableType variable_type) const
if, elseif and else evaluation
void visit_conserve(const ast::Conserve &node) override
visit node of type ast::Conserve
Represents specific element of an array variable.
void visit_statement_block(const ast::StatementBlock &node) override
visit node of type ast::StatementBlock
std::ostream & operator<<(std::ostream &os, DUState state)
void process_variable(const std::string &name)
DefUseAnalyzeVisitor(symtab::SymbolTable &symtab, bool ignore_verbatim)
std::vector< DUInstance > * current_chain
def-use chain currently being constructed
@ NONE
variable is not used
std::shared_ptr< const ast::BinaryExpression > binary_expression
binary expression in which the variable is used/defined
void visit_verbatim(const ast::Verbatim &node) override
We are not analyzing verbatim blocks yet and hence if there is a verbatim block we assume there is va...
Represents GLOBAL statement in NMODL.
std::shared_ptr< const ast::BinaryExpression > current_binary_expression
std::string to_string(bool compact=true) const
return json representation
std::stack< symtab::SymbolTable * > symtab_stack
symbol tables in call hierarchy
Represent symbol table for a NMODL block.
@ CONDITIONAL_BLOCK
conditional block
void visit_argument(const ast::Argument &node) override
visit node of type ast::Argument
void visit_non_lin_equation(const ast::NonLinEquation &node) override
visit node of type ast::NonLinEquation
std::vector< DUInstance > children
usage of variable in case of if like statements
bool visiting_lhs
starting visiting lhs of assignment statement
Represents block encapsulating list of statements.
DUState state
state of the usage
Represents an argument to functions and procedures.
void visit_if_statement(const ast::IfStatement &node) override
visit node of type ast::IfStatement
void visit_local_list_statement(const ast::LocalListStatement &node) override
visit node of type ast::LocalListStatement
@ LU
local variable is used
std::vector< DUInstance > chain
def-use chain for a variable
void visit_name(const ast::Name &node) override
visit node of type ast::Name
symtab::SymbolTable * current_symtab
symbol table for current statement block (or of parent block if doesn't have) should be initialized b...
void visit_var_name(const ast::VarName &node) override
visit node of type ast::VarName
std::string name
name of the node
@ CD
global or local variable is conditionally defined
void visit_reaction_statement(const ast::ReactionStatement &node) override
unsupported statements : we aren't sure how to handle this "yet" and hence variables used in any of t...
DUChain(std::string name, DUVariableType type)
Represents binary expression in the NMODL.
Def-Use chain for an AST node.
void visit_binary_expression(const ast::BinaryExpression &node) override
Nmodl grammar doesn't allow assignment operator on rhs (e.g.
Forward declarations of symbols in namespace nmodl::symtab.
Represent use of a variable in the statement.
Concrete visitor for all AST classes.