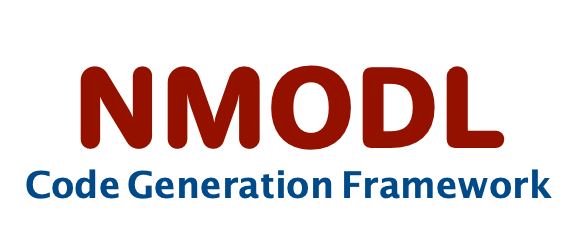 |
User Guide
|
Go to the documentation of this file.
27 bool under_state_block) {
30 if (token_ptr !=
nullptr) {
35 auto symbol = std::make_shared<Symbol>(node->
get_node_name(), node, token);
36 symbol->add_property(property);
39 if (property == NmodlType::nonspecific_cur_var) {
40 symbol->add_property(NmodlType::range_var);
44 if (under_state_block) {
45 symbol->add_property(NmodlType::state_var);
54 std::shared_ptr<Symbol> symbol;
63 symbol->set_order(prime->get_order()->eval());
64 symbol->add_property(property);
72 if (property == NmodlType::range_var || property == NmodlType::nonspecific_cur_var) {
74 if (s && s->has_any_property(NmodlType::nonspecific_cur_var | NmodlType::range_var)) {
75 s->add_property(property);
87 const auto& value = parameter->
get_value();
88 const auto& name = parameter->get_name();
90 symbol->set_value(value->to_double());
92 if (name->is_indexed_name()) {
94 auto length =
dynamic_cast<ast::Integer*
>(index_name->get_length().get());
95 symbol->set_as_array(length->eval());
101 auto length = variable->get_length();
103 symbol->set_as_array(length->eval());
111 symbol->set_value(value->to_double());
117 auto name = variable->get_name();
118 if (name->is_indexed_name()) {
120 auto length =
dynamic_cast<ast::Integer*
>(index_name->get_length().get());
121 symbol->set_as_array(length->eval());
127 symbol->set_value(define->get_value()->to_double());
135 auto name = use_ion->
get_name()->get_node_name();
138 auto symbol = std::make_shared<symtab::Symbol>(ion_variable);
139 symbol->add_property(NmodlType::codegen_var);
152 std::shared_ptr<Symbol> symbol;
154 symbol = std::make_shared<Symbol>(name, node, *node->
get_token());
156 symbol = std::make_shared<Symbol>(name, node,
ModToken{});
158 symbol->add_property(property);
161 symbol->add_property(NmodlType::to_solve);
171 for (
auto variable: variables) {
172 auto symbol = std::make_shared<Symbol>(variable, tok);
173 symbol->add_property(NmodlType::extern_neuron_variable);
178 auto symbol = std::make_shared<Symbol>(method, tok);
179 symbol->add_property(NmodlType::extern_method);
196 block_to_solve.insert(solve_block->get_block_name()->get_node_name());
250 for (
auto& var: variables) {
251 auto name = var->get_node_name();
254 symbol->add_property(property);
255 symbol->set_num_values(num_values);
259 int num_values = node.
get_with()->eval() + 1;
260 update_symbol(node.
get_table_vars(), NmodlType::table_statement_var, num_values);
261 update_symbol(node.
get_depend_vars(), NmodlType::table_assigned_var, num_values);
symtab::ModelSymbolTable * get_model_symbol_table()
Return global symbol table for the mod file.
const NameVector & get_depend_vars() const noexcept
Getter for member variable TableStatement::depend_vars.
Represents a variable in the ast::ConstantBlock.
Base class for all AST node.
std::vector< std::string > get_external_functions()
Return functions that can be used in the NMODL.
Base class for all Abstract Syntax Tree node types.
static std::vector< std::string > get_possible_variables(const std::string &ion_name)
for a given ion, return different variable names/properties like internal/external concentration,...
Represents a statement in ASSIGNED or STATE block.
void add_model_symbol_with_property(ast::Node *node, symtab::syminfo::NmodlType property)
virtual bool is_local_var() const noexcept
Check if the ast node is an instance of ast::LocalVar.
std::shared_ptr< Symbol > insert(const std::shared_ptr< Symbol > &symbol)
insert new symbol into current table
std::shared_ptr< Integer > get_with() const noexcept
Getter for member variable TableStatement::with.
encapsulates code generation backend implementations
Represents a DEFINE statement in NMODL.
static std::shared_ptr< Symbol > create_symbol_for_node(ast::Node *node, NmodlType property, bool under_state_block)
std::string get_node_type_name() const noexcept override
Return type (ast::AstNodeType) of ast node as std::string.
Map different tokens from lexer to token types.
Represents TABLE statement in NMODL.
void leave_scope()
leaving current nmodl block
Represents an integer variable.
virtual bool is_constant_var() const noexcept
Check if the ast node is an instance of ast::ConstantVar.
virtual bool is_assigned_definition() const noexcept
Check if the ast node is an instance of ast::AssignedDefinition.
void visit_table_statement(ast::TableStatement &node) override
Visit table statement and update symbol in symbol table.
void setup_symbol_table_for_program_block(ast::Program *node)
Symtab visitor could be called multiple times, after optimization passes, in which case we have to th...
std::vector< std::shared_ptr< Name > > NameVector
void setup_symbol_table(ast::Ast *node, const std::string &name, bool is_global)
Represents specific element of an array variable.
virtual symtab::SymbolTable * get_symbol_table() const
Return associated symbol table for the AST node.
void setup_symbol(ast::Node *node, symtab::syminfo::NmodlType property)
helper function to setup/insert symbol into symbol table for the ast nodes which are of variable type...
void visit_children(visitor::Visitor &v) override
visit children i.e.
symtab::ModelSymbolTable * modsymtab
void setup_symbol_table_for_scoped_block(ast::Node *node, const std::string &name)
Represents USEION statement in NMODL.
std::shared_ptr< Symbol > lookup(const std::string &name)
lookup for symbol into current as well as all parent tables
Represents a prime variable (for ODE)
virtual bool is_state_block() const noexcept
Check if the ast node is an instance of ast::StateBlock.
virtual const ModToken * get_token() const
Return associated token for the AST node.
Various types to store code generation specific information.
std::shared_ptr< Name > get_name() const noexcept
Getter for member variable Useion::name.
SymbolTable * enter_scope(const std::string &name, ast::Ast *node, bool global, SymbolTable *node_symtab)
entering into new nmodl block
virtual void set_symbol_table(symtab::SymbolTable *symtab)
Set symbol table for the AST node.
virtual bool is_define() const noexcept
Check if the ast node is an instance of ast::Define.
void set_mode(bool update_mode)
re-initialize members to throw away old symbol tables this is required as symtab visitor pass runs mu...
NmodlType
NMODL variable properties.
const NameVector & get_table_vars() const noexcept
Getter for member variable TableStatement::table_vars.
virtual bool is_param_assign() const noexcept
Check if the ast node is an instance of ast::ParamAssign.
virtual bool is_prime_name() const noexcept
Check if the ast node is an instance of ast::PrimeName.
virtual bool is_program() const noexcept
Check if the ast node is an instance of ast::Program.
static void add_external_symbols(symtab::ModelSymbolTable *symtab)
std::string get_node_type_name() const noexcept override
Return type (ast::AstNodeType) of ast node as std::string.
virtual bool is_solve_block() const noexcept
Check if the ast node is an instance of ast::SolveBlock.
std::set< std::string > block_to_solve
const static std::map< std::string, MethodInfo > methods
Integration methods available in the NMODL.
Represents top level AST node for whole NMODL input.
THIS FILE IS GENERATED AT BUILD TIME AND SHALL NOT BE EDITED.
void setup_symbol_table_for_global_block(ast::Node *node)
virtual void visit_children(visitor::Visitor &v)=0
Visit children i.e.
std::shared_ptr< Number > get_value() const noexcept
Getter for member variable ConstantVar::value.
Represent token returned by scanner.
std::vector< std::string > get_external_variables()
Return variables declared in NEURON that are available to NMODL.
virtual std::string get_node_name() const
Return name of of the node.
virtual bool is_useion() const noexcept
Check if the ast node is an instance of ast::Useion.
std::shared_ptr< Number > get_value() const noexcept
Getter for member variable ParamAssign::value.
static constexpr char ION_VARNAME_PREFIX[]
prefix for ion variable