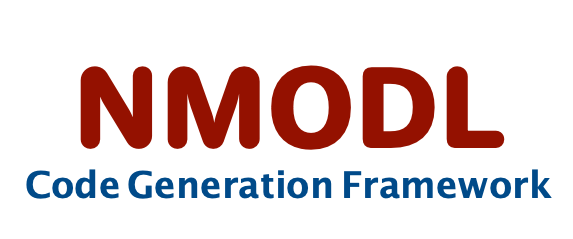 |
User Guide
|
void visit_constructor_block(const ast::ConstructorBlock &node) override
Go through the tree while checking the parents.
Represent LONGITUDINAL_DIFFUSION statement in NMODL.
CheckParentVisitor(bool is_root_with_null_parent=true)
Standard constructor.
Represents a BEFORE block in NMODL.
Represents a variable in the ast::ConstantBlock.
void visit_node(const ast::Node &node) override
Go through the tree while checking the parents.
void visit_before_block(const ast::BeforeBlock &node) override
Go through the tree while checking the parents.
Represents an INCLUDE statement in NMODL.
const bool is_root_with_null_parent
Flag to activate the parent check on the root node.
Base class for all AST node.
Represents a block to be executed before or after another block.
Represents a C code block.
void visit_function_table_block(const ast::FunctionTableBlock &node) override
Go through the tree while checking the parents.
void visit_mutex_lock(const ast::MutexLock &node) override
Go through the tree while checking the parents.
void visit_argument(const ast::Argument &node) override
Go through the tree while checking the parents.
Base class for all identifiers.
void visit_unit_block(const ast::UnitBlock &node) override
Go through the tree while checking the parents.
void visit_program(const ast::Program &node) override
Go through the tree while checking the parents.
void visit_if_statement(const ast::IfStatement &node) override
Go through the tree while checking the parents.
void visit_eigen_linear_solver_block(const ast::EigenLinearSolverBlock &node) override
Go through the tree while checking the parents.
Concrete constant visitor for all AST classes.
Represents differential equation in DERIVATIVE block.
Base class for all Abstract Syntax Tree node types.
Represents a statement in ASSIGNED or STATE block.
void visit_param_assign(const ast::ParamAssign &node) override
Go through the tree while checking the parents.
void visit_react_var_name(const ast::ReactVarName &node) override
Go through the tree while checking the parents.
Represents a double variable.
Represents RANGE variables statement in NMODL.
void visit_electrode_current(const ast::ElectrodeCurrent &node) override
Go through the tree while checking the parents.
void visit_procedure_block(const ast::ProcedureBlock &node) override
Go through the tree while checking the parents.
void visit_factor_def(const ast::FactorDef &node) override
Go through the tree while checking the parents.
Represents RANDOM statement in NMODL.
Represent a single variable of type BBCOREPOINTER.
void visit_verbatim(const ast::Verbatim &node) override
Go through the tree while checking the parents.
void visit_range_var(const ast::RangeVar &node) override
Go through the tree while checking the parents.
void visit_string(const ast::String &node) override
Go through the tree while checking the parents.
void visit_else_statement(const ast::ElseStatement &node) override
Go through the tree while checking the parents.
void visit_while_statement(const ast::WhileStatement &node) override
Go through the tree while checking the parents.
Represent CONSERVE statement in NMODL.
void visit_extern_var(const ast::ExternVar &node) override
Go through the tree while checking the parents.
void visit_constant_block(const ast::ConstantBlock &node) override
Go through the tree while checking the parents.
Represents CONDUCTANCE statement in NMODL.
void visit_mutex_unlock(const ast::MutexUnlock &node) override
Go through the tree while checking the parents.
void visit_statement(const ast::Statement &node) override
Go through the tree while checking the parents.
const ast::Ast * parent
Keeps track of the parents while going down the tree.
void visit_function_call(const ast::FunctionCall &node) override
Go through the tree while checking the parents.
encapsulates code generation backend implementations
void visit_nonspecific_cur_var(const ast::NonspecificCurVar &node) override
Go through the tree while checking the parents.
void visit_line_comment(const ast::LineComment &node) override
Go through the tree while checking the parents.
void visit_kinetic_block(const ast::KineticBlock &node) override
Go through the tree while checking the parents.
Represents a DEFINE statement in NMODL.
void visit_double_unit(const ast::DoubleUnit &node) override
Go through the tree while checking the parents.
void visit_unit_def(const ast::UnitDef &node) override
Go through the tree while checking the parents.
void visit_external(const ast::External &node) override
Go through the tree while checking the parents.
void visit_name(const ast::Name &node) override
Go through the tree while checking the parents.
void visit_for_netcon(const ast::ForNetcon &node) override
Go through the tree while checking the parents.
Represent MUTEXLOCK statement in NMODL.
void visit_lin_equation(const ast::LinEquation &node) override
Go through the tree while checking the parents.
void visit_indexed_name(const ast::IndexedName &node) override
Go through the tree while checking the parents.
void visit_ontology_statement(const ast::OntologyStatement &node) override
Go through the tree while checking the parents.
Represents TABLE statement in NMODL.
Represents an integer variable.
Represents SUFFIX statement in NMODL.
Single variable of type RANDOM.
void visit_random_var(const ast::RandomVar &node) override
Go through the tree while checking the parents.
void visit_lag_statement(const ast::LagStatement &node) override
Go through the tree while checking the parents.
void visit_assigned_definition(const ast::AssignedDefinition &node) override
Go through the tree while checking the parents.
void visit_linear_block(const ast::LinearBlock &node) override
Go through the tree while checking the parents.
Represents a ASSIGNED block in the NMODL.
void visit_float(const ast::Float &node) override
Go through the tree while checking the parents.
void visit_protect_statement(const ast::ProtectStatement &node) override
Go through the tree while checking the parents.
Represent newton solver solution block based on Eigen.
void visit_var_name(const ast::VarName &node) override
Go through the tree while checking the parents.
void check_parent(const ast::Ast &node) const
Check the parent, throw an error if not.
void visit_identifier(const ast::Identifier &node) override
Go through the tree while checking the parents.
void visit_binary_operator(const ast::BinaryOperator &node) override
Go through the tree while checking the parents.
void visit_solve_block(const ast::SolveBlock &node) override
Go through the tree while checking the parents.
void visit_suffix(const ast::Suffix &node) override
Go through the tree while checking the parents.
void visit_model(const ast::Model &node) override
Go through the tree while checking the parents.
Base class for all block scoped nodes.
Represents a INITIAL block in the NMODL.
void visit_bbcore_pointer_var(const ast::BbcorePointerVar &node) override
Go through the tree while checking the parents.
Visitor for checking parents of ast nodes
void visit_breakpoint_block(const ast::BreakpointBlock &node) override
Go through the tree while checking the parents.
Represent WATCH statement in NMODL.
Represents a float variable.
Represents a BREAKPOINT block in NMODL.
Represents specific element of an array variable.
void visit_bbcore_pointer(const ast::BbcorePointer &node) override
Go through the tree while checking the parents.
Represents a DESTRUCTOR block in the NMODL.
void visit_read_ion_var(const ast::ReadIonVar &node) override
Go through the tree while checking the parents.
void visit_destructor_block(const ast::DestructorBlock &node) override
Go through the tree while checking the parents.
void visit_conductance_hint(const ast::ConductanceHint &node) override
Go through the tree while checking the parents.
Represents a CONSTRUCTOR block in the NMODL.
void visit_valence(const ast::Valence &node) override
Go through the tree while checking the parents.
void visit_else_if_statement(const ast::ElseIfStatement &node) override
Go through the tree while checking the parents.
Represent MUTEXUNLOCK statement in NMODL.
void visit_initial_block(const ast::InitialBlock &node) override
Go through the tree while checking the parents.
void visit_derivative_block(const ast::DerivativeBlock &node) override
Go through the tree while checking the parents.
void visit_include(const ast::Include &node) override
Go through the tree while checking the parents.
void visit_electrode_cur_var(const ast::ElectrodeCurVar &node) override
Go through the tree while checking the parents.
int check_ast(const ast::Ast &node)
A small wrapper to have a nicer call in parser.cpp.
Represents USEION statement in NMODL.
void visit_paren_expression(const ast::ParenExpression &node) override
Go through the tree while checking the parents.
void visit_write_ion_var(const ast::WriteIonVar &node) override
Go through the tree while checking the parents.
void visit_local_var(const ast::LocalVar &node) override
Go through the tree while checking the parents.
void visit_statement_block(const ast::StatementBlock &node) override
Go through the tree while checking the parents.
void visit_unit(const ast::Unit &node) override
Go through the tree while checking the parents.
void visit_useion(const ast::Useion &node) override
Go through the tree while checking the parents.
void visit_integer(const ast::Integer &node) override
Go through the tree while checking the parents.
void visit_ba_block(const ast::BABlock &node) override
Go through the tree while checking the parents.
Represents GLOBAL statement in NMODL.
void visit_independent_block(const ast::IndependentBlock &node) override
Go through the tree while checking the parents.
void visit_number(const ast::Number &node) override
Go through the tree while checking the parents.
void visit_global_var(const ast::GlobalVar &node) override
Go through the tree while checking the parents.
void visit_neuron_block(const ast::NeuronBlock &node) override
Go through the tree while checking the parents.
Represent CONSTANT block in the mod file.
void visit_constant_statement(const ast::ConstantStatement &node) override
Go through the tree while checking the parents.
void visit_reaction_operator(const ast::ReactionOperator &node) override
Go through the tree while checking the parents.
Represents a prime variable (for ODE)
void visit_number_range(const ast::NumberRange &node) override
Go through the tree while checking the parents.
Represents DERIVATIVE block in the NMODL.
Represents a boolean variable.
void visit_diff_eq_expression(const ast::DiffEqExpression &node) override
Go through the tree while checking the parents.
void visit_conserve(const ast::Conserve &node) override
Go through the tree while checking the parents.
Represent linear solver solution block based on Eigen.
void visit_function_block(const ast::FunctionBlock &node) override
Go through the tree while checking the parents.
Represents a LAG statement in the mod file.
Represent COMPARTMENT statement in NMODL.
Statement to indicate a change in timestep in a given block.
void visit_solution_expression(const ast::SolutionExpression &node) override
Go through the tree while checking the parents.
void visit_after_block(const ast::AfterBlock &node) override
Go through the tree while checking the parents.
void visit_prime_name(const ast::PrimeName &node) override
Go through the tree while checking the parents.
void visit_wrapped_expression(const ast::WrappedExpression &node) override
Go through the tree while checking the parents.
Represents a STATE block in the NMODL.
void visit_random_var_list(const ast::RandomVarList &node) override
Go through the tree while checking the parents.
void visit_nonspecific(const ast::Nonspecific &node) override
Go through the tree while checking the parents.
void visit_block(const ast::Block &node) override
Go through the tree while checking the parents.
void visit_binary_expression(const ast::BinaryExpression &node) override
Go through the tree while checking the parents.
Represents the coreneuron nrn_state callback function.
Represents block encapsulating list of statements.
void visit_unary_operator(const ast::UnaryOperator &node) override
Go through the tree while checking the parents.
void visit_watch_statement(const ast::WatchStatement &node) override
Go through the tree while checking the parents.
Represents CURIE information in NMODL.
This construct is deprecated and no longer supported in the NMODL.
Represents an argument to functions and procedures.
void visit_constant_var(const ast::ConstantVar &node) override
Go through the tree while checking the parents.
void visit_unit_state(const ast::UnitState &node) override
Go through the tree while checking the parents.
Represents LINEAR block in the NMODL.
Represent a callback to NEURON's derivimplicit solver.
void visit_state_block(const ast::StateBlock &node) override
Go through the tree while checking the parents.
void visit_ba_block_type(const ast::BABlockType &node) override
Go through the tree while checking the parents.
void visit_define(const ast::Define &node) override
Go through the tree while checking the parents.
void visit_range(const ast::Range &node) override
Go through the tree while checking the parents.
Represent statement in CONSTANT block of NMODL.
Operator used in ast::BinaryExpression.
void visit_update_dt(const ast::UpdateDt &node) override
Go through the tree while checking the parents.
Type to represent different block types for before/after block.
Represents NONLINEAR block in the NMODL.
Represent solution of a block in the AST.
void visit_expression(const ast::Expression &node) override
Go through the tree while checking the parents.
void visit_from_statement(const ast::FromStatement &node) override
Go through the tree while checking the parents.
void visit_pointer(const ast::Pointer &node) override
Go through the tree while checking the parents.
Represents a INDEPENDENT block in the NMODL.
void visit_eigen_newton_solver_block(const ast::EigenNewtonSolverBlock &node) override
Go through the tree while checking the parents.
void visit_compartment(const ast::Compartment &node) override
Go through the tree while checking the parents.
Represents BBCOREPOINTER statement in NMODL.
Represents NONSPECIFIC_CURRENT variables statement in NMODL.
void visit_expression_statement(const ast::ExpressionStatement &node) override
Go through the tree while checking the parents.
void visit_table_statement(const ast::TableStatement &node) override
Go through the tree while checking the parents.
void visit_thread_safe(const ast::ThreadSafe &node) override
Go through the tree while checking the parents.
void visit_nrn_state_block(const ast::NrnStateBlock &node) override
Go through the tree while checking the parents.
void visit_derivimplicit_callback(const ast::DerivimplicitCallback &node) override
Go through the tree while checking the parents.
void visit_net_receive_block(const ast::NetReceiveBlock &node) override
Go through the tree while checking the parents.
Represents top level AST node for whole NMODL input.
void visit_unary_expression(const ast::UnaryExpression &node) override
Go through the tree while checking the parents.
void visit_discrete_block(const ast::DiscreteBlock &node) override
Go through the tree while checking the parents.
void visit_global(const ast::Global &node) override
Go through the tree while checking the parents.
void visit_assigned_block(const ast::AssignedBlock &node) override
Go through the tree while checking the parents.
Represents a AFTER block in NMODL.
Base class for all numbers.
Represents THREADSAFE statement in NMODL.
Base class for all expressions in the NMODL.
void visit_watch(const ast::Watch &node) override
Go through the tree while checking the parents.
Represents POINTER statement in NMODL.
Represents ELECTRODE_CURRENT variables statement in NMODL.
Represent NEURON block in the mod file.
void visit_double(const ast::Double &node) override
Go through the tree while checking the parents.
void visit_non_lin_equation(const ast::NonLinEquation &node) override
Go through the tree while checking the parents.
void visit_param_block(const ast::ParamBlock &node) override
Go through the tree while checking the parents.
Represents binary expression in the NMODL.
Represents a PARAMETER block in the NMODL.
void visit_boolean(const ast::Boolean &node) override
Go through the tree while checking the parents.
void visit_pointer_var(const ast::PointerVar &node) override
Go through the tree while checking the parents.
void visit_local_list_statement(const ast::LocalListStatement &node) override
Go through the tree while checking the parents.
void visit_block_comment(const ast::BlockComment &node) override
Go through the tree while checking the parents.
void visit_reaction_statement(const ast::ReactionStatement &node) override
Go through the tree while checking the parents.
void visit_non_linear_block(const ast::NonLinearBlock &node) override
Go through the tree while checking the parents.
Wrap any other expression type.
void visit_limits(const ast::Limits &node) override
Go through the tree while checking the parents.
void visit_lon_difuse(const ast::LonDifuse &node) override
Go through the tree while checking the parents.
Concrete visitor for all AST classes.